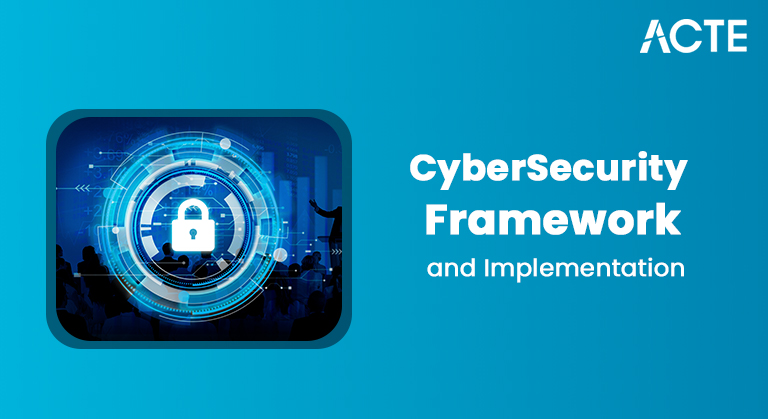
- Introduction to TextInput in React Native
- Handling User Input with TextInput
- Customizing Styles for TextInput
- Handling Multiline Text Inputs
- Validating Input Data in TextInput
- Placeholder Text and Text Formatting
- Secure Text Input with TextInput
- Handling Keyboard Events in React Native
- Managing Focus and Blur Events
- TextInput and Form Management
- Performance Optimization for TextInput
- Common Issues with TextInput in React Native
Introduction to TextInput in React Native
In React Native, the TextInput component is a core element used to capture user input, playing a crucial role in building features like forms, login screens, chat interfaces, contact forms, and search bars. As one of the most frequently used components in mobile app development, it offers a high degree of customization through a variety of props that allow developers to manage its behavior, style, and interaction with other elements. For those looking to strengthen their front-end and mobile UI skills, exploring Web Designing & Development Courses can provide valuable practical knowledge. You can control keyboard types, handle focus events, manage text changes, and integrate it with validation logic, making it suitable for both simple and complex input needs. One of its major strengths is cross-platform support, providing a consistent experience across iOS and Android without the need for separate codebases. This ensures that users on different devices interact with a unified and responsive UI. Understanding how to effectively use and tailor TextInput not only streamlines the development process but also enhances the overall usability and functionality of mobile applications, making it an essential tool for capturing user-driven data in React Native projects.
Handling User Input with TextInput
Handling user input using TextInput in React Native is a fundamental part of creating interactive mobile applications. It is most commonly used to capture and manage user-entered text for actions like form submissions, data validation, or live updates. React Native’s TextInput component provides several key props to make this possible, including value and onChangeText. The value prop allows the developer to control what text appears in the input field by binding it to a state variable, ensuring the input reflects the latest data at all times. Meanwhile, onChangeText is used to update that state whenever the user types, enabling real-time synchronization between the UI and the app’s logic. This approach creates a controlled component, where the displayed value is always determined by the application’s current state. As the user types, the state updates instantly, keeping the UI responsive and consistent. Understanding this process is an essential part of the broader Scope of Web Development where interactive and dynamic user interfaces play a central role. This mechanism is crucial for ensuring a smooth user experience and maintaining clean, predictable data flow throughout the app. By combining state management with TextInput’s built-in features, developers can easily implement dynamic, user-friendly interfaces in React Native.
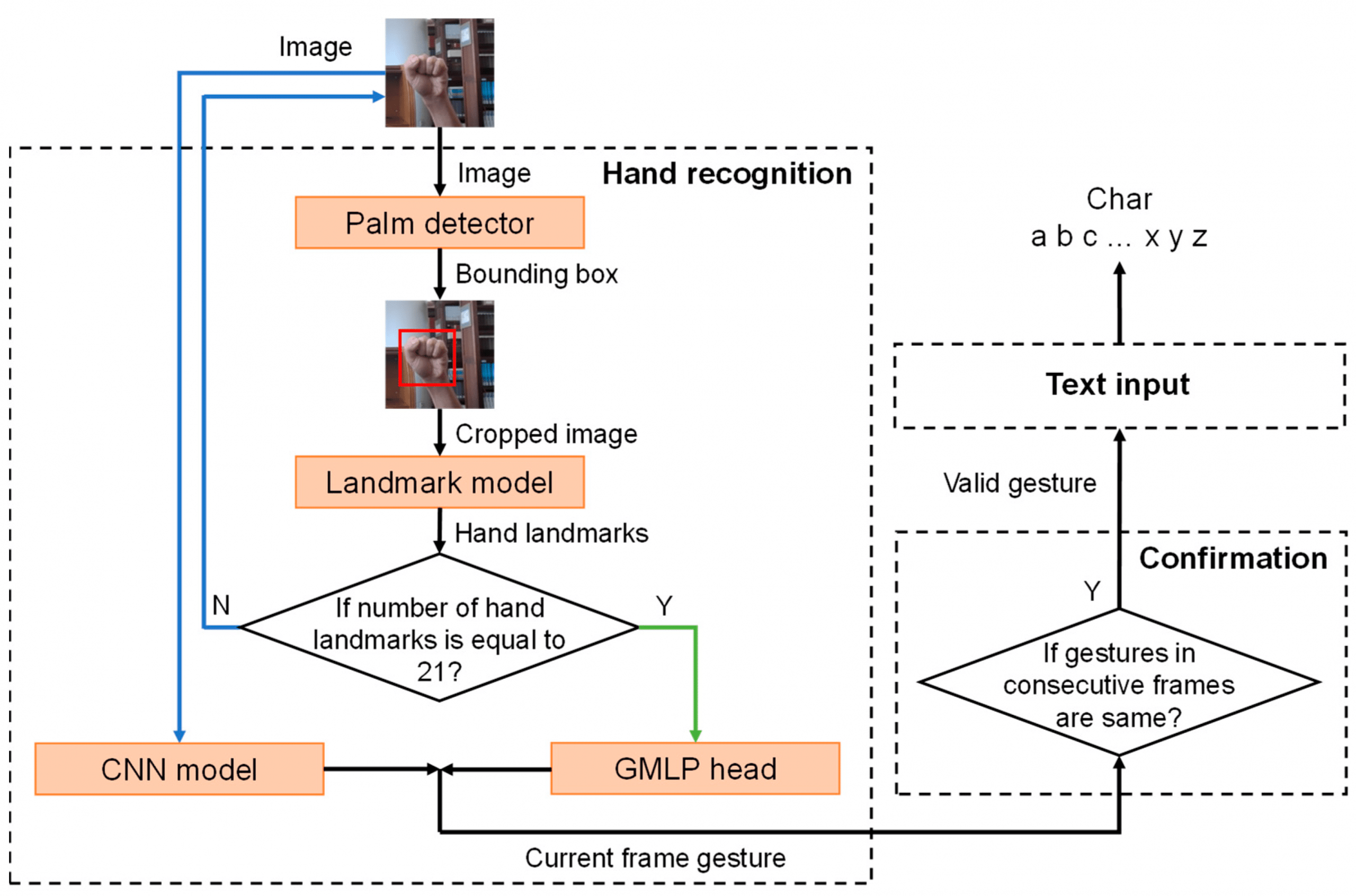
Customizing Styles for TextInput
Customizing the appearance of TextInput in React Native is an important step in building polished, user-friendly interfaces. With a wide range of styling options, developers can tailor input fields to match their app’s design language and improve overall usability. The style prop in React Native makes it easy to apply both basic and advanced styles, enabling full control over how the input looks and behaves.
Here are some key customization options available with TextInput:
- Font Styling: Modify font size, color, and family to align with your app’s typography.
- Borders: Adjust border width, color, and style to define input boundaries clearly.
- Padding and Margin: Use padding for inner spacing and margin for layout spacing between elements.
- Background Color: Set background colors to make the input field stand out or blend in with the UI.
- Rounded Corners: Apply borderRadius to give the input rounded edges for a softer look.
- Shadow Effects: Use shadowColor, shadowOffset, and shadowOpacity (mainly on iOS) to create depth.
- Custom Fonts: Assign a fontFamily to use branded or stylistic fonts within your input fields.
- TextInput
- multiline={true}
- numberOfLines={4}
- style={{
- height: 100,
- borderColor: ‘gray’,
- borderWidth: 1,
- padding: 10,
- }}
- placeholder “Enter multiple lines of text”
- Real-Time Validation: Use the onChangeText prop to apply validation logic as the user types.
- Email Format Check: Use regular expressions to ensure the input matches standard email formats.
- Error Handling: Store and display error messages in state to guide users when input is invalid.
- Required Fields: Ensure that critical fields are not left blank before allowing form submission.
- User Feedback: Show error messages near the input to improve usability and reduce confusion.
- Custom Rules: Implement custom validation rules such as password strength, length restrictions, or specific formats depending on your app’s needs.
- Placeholder: Displays “Enter your username” when the input is empty.
- Placeholder Text Color: Sets the color of the placeholder text to gray.
- Border: Adds a black border with a thickness of 1 pixel around the input field.
- Padding: Adds 10 pixels of space on the left inside the input field.
- Height: Sets the input field height to 40 pixels.
- Font Size: Sets the text size inside the input field to 18 pixels.
- TextInput
- secureTextEntry={true}
- placeholder “Enter your password”
- style={{
- borderColor: ‘black’,
- borderWidth: 1,
- paddingLeft: 10,
- height: 40,
- fontSize: 18,
- }}
- Triggered when the “return” key is pressed.
- Logs “Submit key pressed”! to the console. Placeholde
- Use useCallback or useMemo to prevent unnecessary re-renders.
- Avoid storing large datasets in the component’s state.
- Utilize React. Memo or PureComponent for wrapping components that don’t need to re-render unless their props change.
- Please limit the number of uncontrolled TextInput fields or manage them effectively with libraries like Formik.
- Keyboard Overlap: The keyboard may overlap TextInput fields on some devices, especially in forms. To resolve this, use the KeyboardAvoidingView or ScrollView component to adjust the layout dynamically.
- TextInput not Updating: If your TextInput doesn’t update correctly, it might be due to improper state handling or misconfiguration of the value prop. Ensure the component’s value is tied to a state variable and updated using onChangeText.
- Placeholder Not Showing: The placeholder may not display properly if the TextInput’s background color is too similar to the placeholder text. Make sure there is enough contrast to make the placeholder visible. Enrolling in Web Designing Training can help you better understand design principles like color contrast, accessibility, and UI best practices to avoid such issues.
- Performance Issues: The TextInput can cause performance issues if it’s not used efficiently in forms with many fields. Optimize your components using techniques like memoization or state management libraries.
- Accessibility Concerns: Ensure your TextInput is accessible to all users, including screen readers. Use the accessibilityLabel and accessibilityHint props to make TextInput accessible to users with disabilities.
These styling capabilities ensure that TextInput not only functions well but also integrates seamlessly into the visual design of your app.
Handling Multiline Text Inputs
For scenarios where users need to input more than one line of text, React Native provides a multiline option for the TextInput component. This is particularly useful in applications such as messaging, note-taking, or feedback forms. Understanding how this fits within broader Web Development Technologies helps developers build more versatile and user-centric interfaces. By setting the multiline prop to true, TextInput will expand vertically to accommodate multiple lines of text.
In this example, the number of lines sets the initial visible height of the TextInput to display four lines. The TextInput expands as the user types more lines, making it suitable for handling large amounts of text. Handling multiline text input can be tricky on mobile devices due to varying screen sizes and keyboard behavior. However, React Native provides automatic layout handling, so developers don’t have to worry about manual adjustments.
Advance your Web Development career by joining this Web Developer Certification Courses now.
Validating Input Data in TextInput
Validating user input in a TextInput field is an essential part of building reliable and user-friendly mobile applications. It ensures that the data entered by the user meets expected formats and prevents invalid or incomplete information from being submitted. Whether you’re checking for a valid email address, enforcing password complexity, or ensuring required fields are filled, applying validation logic improves both functionality and user experience. In fact, understanding input validation is a common thread across various software engineering types as it plays a critical role in ensuring data integrity and application stability. Real-time validation, especially using the onChangeText callback, provides immediate feedback as the user types, helping them correct errors before submission.
Here are key points to consider when validating input in TextInput:
These practices ensure that data entered through TextInput is valid, improving the quality of data and enhancing the overall user experience.
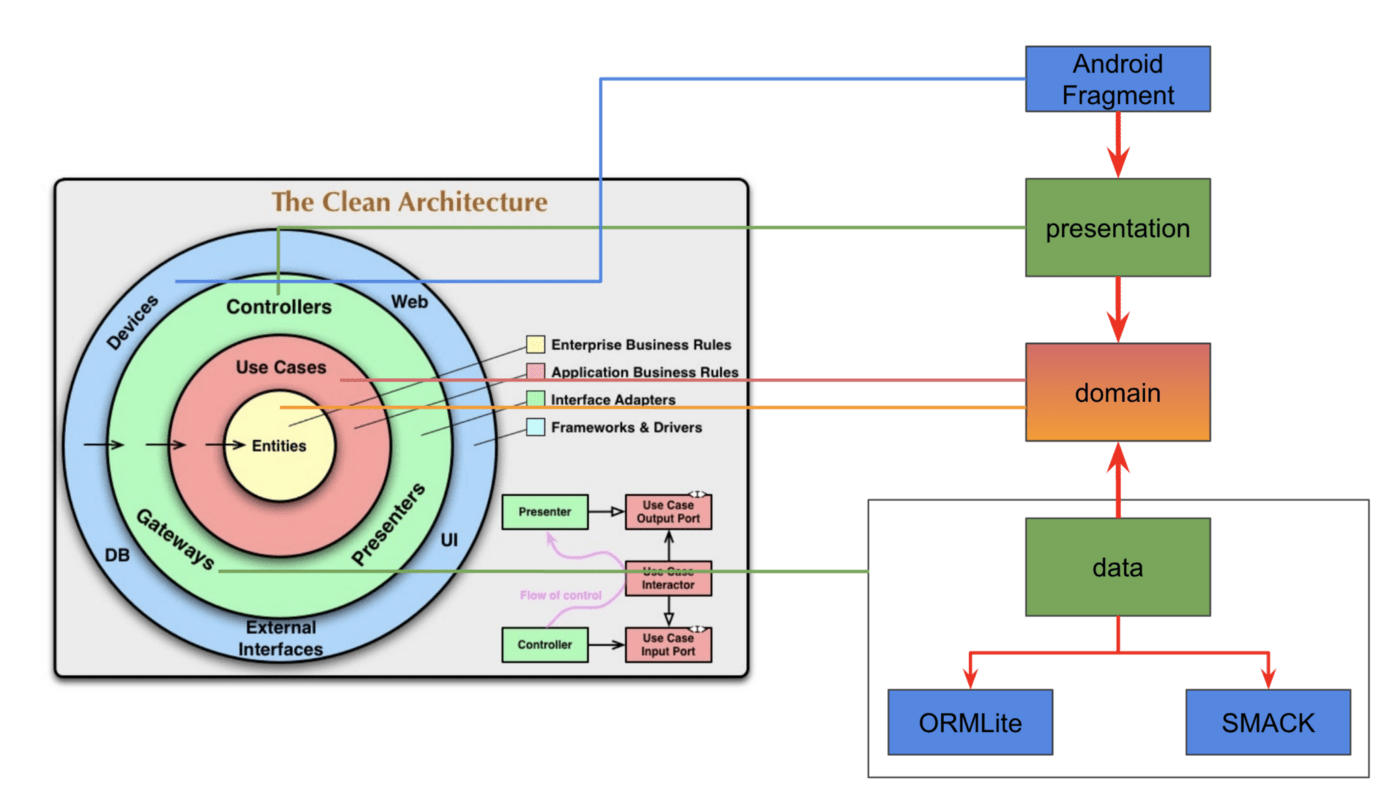
Placeholder Text and Text Formatting
The placeholder text in a TextInput component is a helpful feature to guide users about the expected input type. It appears in the text field before the user begins typing. Understanding how such UI elements Render in ReactJS provides valuable insight into how placeholder behavior integrates with React’s rendering lifecycle. You can customize the placeholder’s appearance using the placeholderTextColor prop to change its color and make it stand out in a visually pleasing way.
This code customizes the appearance of a TextInput field.
In this example, the placeholder text is set to gray, and the style, including a border, padding, and font size, is applied to the TextInput field. Formatting placeholder text enhances the user experience by giving visual hints about the type of input expected.
Secure Text Input with TextInput
Secure text input is significant for sensitive information like passwords or credit card numbers. React Native provides a built-in feature to mask the text entered into a TextInput field using the secureTextEntry prop. This prop ensures that text entered into the TextInput is obscured with dots or asterisks, making it suitable for passwords or other sensitive data.
In this example, the secureTextEntry prop is set to true, which ensures that the input text is hidden, providing better security and privacy for users. This is an essential feature in any login or authentication process.
Handling Keyboard Events in React Native
React Native’s TextInput component interacts directly with the device’s keyboard, and developers can control how the keyboard behaves. React Native provides several events for handling keyboard interactions, such as onFocus, onBlur, onSubmitEditing, and onEndEditing. These events help you manage when the keyboard shows, hides, or submits, improving the user experience. Understanding how these interactions align with backend processes can also be crucial for seamless app development, especially when considering How to Become a Backend Developer and the need for efficient server-client communication. For example, you can use the onSubmitEditing event to automatically navigate to the next input field when the user presses onSubmitEditing
Displays “Type something” when the input is empty.
Additionally, React Native provides the Keyboard API, which gives developers finer control over the keyboard, such as dismissing it when a user taps outside the TextInput or navigates the app. Managing keyboard events enhances the interaction flow and ensures the UI is responsive to user input.
Preparing for Web Development interviews? Visit our blog for the best Web Development Interview Questions and Answers!
Managing Focus and Blur Events
Managing focus and blur events is key to improving user interactions with forms and input fields. The focus event triggers when the TextInput gains focus, while the blur event triggers when it loses focus. These events can be helpful for tasks such as styling the TextInput or triggering actions based on whether the input field is active. In React Native, you can use the onFocus and onBlur events to detect when a TextInput field gains or loses focus. For example, the onFocus event triggers when the input field is focused, and it can log a message such as “Focused!” to the console, while the onBlur event is triggered when the input field loses focus, logging “Blurred!” to the console. These events can also be used to apply custom styles dynamically. For instance, you can change the border color of the TextInput when it is focused by using the onFocus event to set the border color to blue and reset it to gray when the field is blurred using the onBlur event. This is done by managing the border color in a state variable and updating the style accordingly. Pursuing a Web Developer Certification can help solidify your understanding of user interaction patterns like these and enhance your ability to build responsive, user-friendly applications. This approach enhances the user experience by providing visual feedback and making the app feel more interactive and responsive.
TextInput and Form Management
Managing multiple TextInput fields becomes essential for collecting and validating user input when working with forms. React Native’s controlled components and state management are ideal for handling form input. You can manage a group of TextInput fields using useState for each field or through a form management library like Formik or React Hook Form. These libraries provide convenient form validation, submission, and state management methods. When comparing tools, it’s useful to understand Angular vs React in terms of handling forms and state management, as both frameworks have their strengths. In this example, the useState hook is used to handle multiple input fields in a form. Two state variables, name and email, are created with their respective setter functions, setName and setEmail, to store the values entered by the user. The TextInput components are each linked to one of these state variables, so the input field reflects the current state. The onChangeText prop is used to update the state as the user types. When the user presses the “Submit” button, the handleSubmit function is called, which performs the form submission or validation and logs the entered values for name and email to the console. This approach helps in managing multiple fields efficiently and ensures that each input field is properly handled in the component’s state. Using this approach, you can efficiently handle multiple TextInput fields in a form. Libraries like Formik simplify this process by automatically handling the form state, validation, and submission.
Performance Optimization for TextInput
Optimizing performance to ensure that your app remains responsive is essential when dealing with many TextInput fields. React Native TextInput components are lightweight, but excessive re-renders or improper state management can cause performance issues.
To optimize performance, you should:
Applying performance optimizations ensures a smoother user experience, especially in forms or chat applications with numerous TextInput components.
Common Issues with TextInput in React Native
Despite its versatility, TextInput can pose several challenges during development: