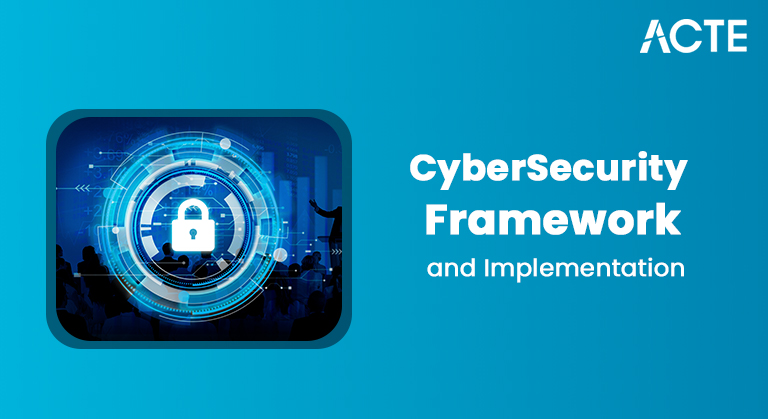
- Introduction to React
- What are Pure Components?
- How Pure Components Work
- Creating Pure Components
- Pure Components vs Regular Components
- When to Use Pure Components
- Performance Considerations
- Limitations of Pure Components
- Best Practices
- Conclusion
Introduction to React
React is a powerful and popular JavaScript library developed by Facebook. It is primarily used for building user interfaces (UIs) for single-page applications (SPAs). It allows developers to create reusable components, each responsible for rendering a part of the user interface. The key concept behind React is the idea of components, which can be either stateful or stateless, and the efficient re-rendering mechanism that React employs to keep the UI up-to-date with the application’s state a fundamental topic in Web Developer Training. React’s design revolves around virtual DOM and reconciliation algorithms, which help optimize performance when updating the UI. However, despite these optimizations, React applications can still become slow if components are re-rendered unnecessarily. This is where Pure Components come into play, effectively preventing redundant rendering and improving performance.
Excited to Achieve Your Business Analyst Certification? View The Business Analyst Online course Offered By ACTE Right Now!
What are Pure Components?
A Pure Component in React only re-renders when it’s props or state change. In contrast to regular components, Pure Components perform a shallow comparison of their props and state to determine whether a re-render is necessary. If the props and state have not changed, React skips the re-rendering process, leading to performance improvements, especially in complex UIs. A pure component optimizes over regular components. React provides the React. Pure Component class, which extends React. Component. By using React. Pure Component, you don’t need to implement a should Component Update method to perform the shallow comparison manually; it’s done automatically.
How Pure Components Work
React components, whether functional or class-based, render the UI based on the current state and props. Each time the state or props change, React triggers a re-render to reflect the changes in the UI. This process can be inefficient if components are re-rendered even when their props or state haven’t changed. A Pure Component leverages a built-in mechanism to avoid unnecessary re-renders, which is especially beneficial in Web Developer for optimizing performance. If there are no changes (i.e., the shallow comparison returns accurate), the component won’t re-render. This shallow comparison checks whether primitive values like strings, numbers, or booleans have changed and whether references to objects or arrays differ. This mechanism works well when the component’s state and props are immutable or don’t change often. However, if the props or state are mutable or complex data structures are used, developers must ensure that data mutations are managed carefully to avoid issues.
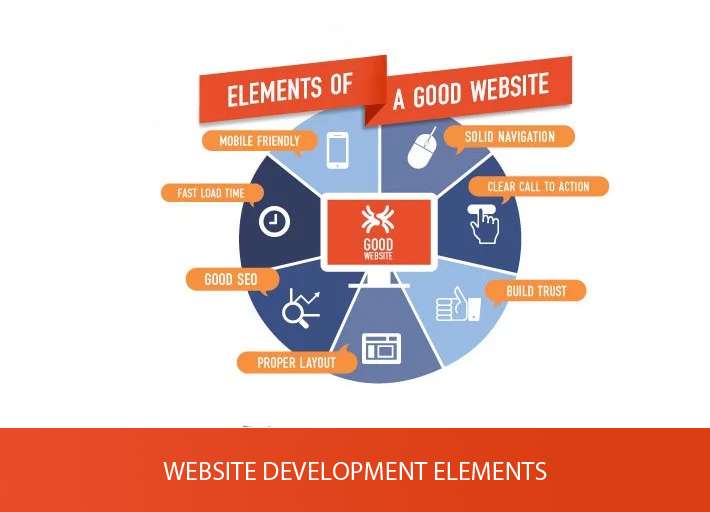
Creating Pure Components
Creating a Pure Component is simple. For class-based components, you can extend React.PureComponent instead of React.Component. React provides hooks like useMemo and useCallback for functional components to achieve similar optimizations without the need for class-based components. In the case of class components, here is how you create a Pure Component:
Using React.PureComponent: When extending React.PureComponent, React automatically performs a shallow comparison of props and state, skipping re-renders when the values are unchanged.
- Import React from ‘react’;
- Class MyPureComponent extends React.PureComponent {
- render() {
- return {this.props.name};
- }
- }
In this example, MyPureComponent will only re-render if the name prop changes. For functional components, you can achieve similar functionality using the React.memo() higher-order component (HOC). This React function will optimize rendering by memoizing the result and skipping unnecessary re-renders when the props haven’t changed.
- Import React from ‘react’;
- const MyPureComponent = React.memo(function MyPureComponent(props) {
- return {props.name};
- });
In this case, the React. Memo ensures that the MyPureComponent will only re-render when the name prop changes.
Pure Components vs Regular Components
To fully understand the importance of Pure Components, it’s essential to compare them to regular React components:
Re-rendering Behavior:
- Regular Components: In regular React components, the should ComponentUpdate method must be implemented manually (if optimizing Performance Testing). This method allows you to decide whether the element should re-render based on changes to its state and props.
- Pure Components: In Pure Components, React automatically implements should ComponentUpdate with a shallow comparison of state and props, thus optimizing the re-rendering process without any extra code.
- Regular Components: Every time a prop or state change occurs, React re-renders the component, even if the changes don’t affect its output.
- Pure Components: Pure Components will only re-render if the props or state change, thus improving performance in situations where components repeatedly receive the same data.
- Regular Components: Developers have more control over the rendering process because they can define custom logic in should ComponentUpdate. However, this also means that developers must remember to optimize rendering manually.
- Pure Components: Pure Components automate this optimization, so developers don’t have to write extra code to ensure that re-renders are only triggered when necessary. However, this comes with the assumption that props and state are shallowly comparable.
- Regular Components: More flexible and suitable for complex scenarios where Web Developer need precise control over the component’s rendering behavior.
- Pure Components: These are best suited for situations where components are used with immutable data, and the props and state can be shallowly compared.
- shouldComponentUpdate
- useMemo
- useCallback
- Shallow Comparison Limitations: React’s shallow comparison only works for primitive types (strings, numbers, booleans, etc.) and references (objects, arrays, functions). If the props or state contain nested structures or mutable data, Pure Components might not detect changes correctly. In such cases, you may use deep comparisons or change how data is passed to the component.
- Overhead of Comparison: The process of performing the shallow comparison itself introduces some overhead. While this is generally a small cost, this overhead could offset the performance gains from skipping re-renders in highly dynamic Dynamic Web Applications with frequent updates.
- Complex State and Props: When state and props are complex (such as deeply nested objects or arrays), developers must ensure that data mutations are handled immeasurable and carefully. Otherwise, the shallow comparison might fail to detect changes, leading to bugs or incorrect behavior.
- Memoization: For functional components, React’s memo() function can provide optimizations similar to ours. Memoization is a technique that involves caching the results of expensive function calls and only recalculating the result when the inputs change. This can be useful for preventing unnecessary renders in functional components.
- Use Pure Components for Simple, Immutable Data Pure Components are most effective when props and state are immutable and composed of simple data types like primitives or stable references.
- Ensure Proper Data Handling Avoid directly mutating objects or arrays. Instead, follow immutable patterns and consider libraries like Redux or Immer for managing complex data structures.
- Avoid Overuse Don’t make every component a Pure Component. Focus on areas where performance issues are observed, and optimize those components selectively.
- Consider Memoization For functional components, use React.memo to prevent unnecessary re-renders, and utilize useMemo and useCallback hooks for optimizing computations and event handlers.
- Profile and Optimize Use React Developer Tools’ Profiler to detect unnecessary re-renders and assess the impact of optimizations. Make data-driven decisions to apply Pure Components effectively.
Performance:
Excited to Obtaining Your Business Analyst Certificate? View The Business Analyst Training Offered By ACTE Right Now!
Ease of Use:
Use Cases:
Interested in Pursuing Business Intelligence Master’s Program? Enroll For Business Intelligence Master Course Today!
When to Use Pure Components
Pure Components are beneficial when performance optimization is required, particularly in large applications with many components, but they should be used thoughtfully and selectively. They are especially useful in scenarios where components rely on immutable data, as Pure Components perform shallow comparisons of props and state to determine if a re-render is necessary a concept often emphasized in Web Developer Training. When the data passed to a component doesn’t change frequently, this can effectively prevent unnecessary re-renders. Another ideal use case is when a component repeatedly renders the same props and state for example, in static lists or UI elements that don’t update often where Pure Components can help avoid redundant rendering. In large-scale applications with complex UI structures, they can contribute significantly to smoother performance by reducing the number of updates React needs to process. However, Pure Components are not always the best solution. If the component relies on mutable data or uses complex, deeply nested objects or arrays, shallow comparison may not detect changes accurately. In such cases, implementing custom
may offer better control over rendering behavior.
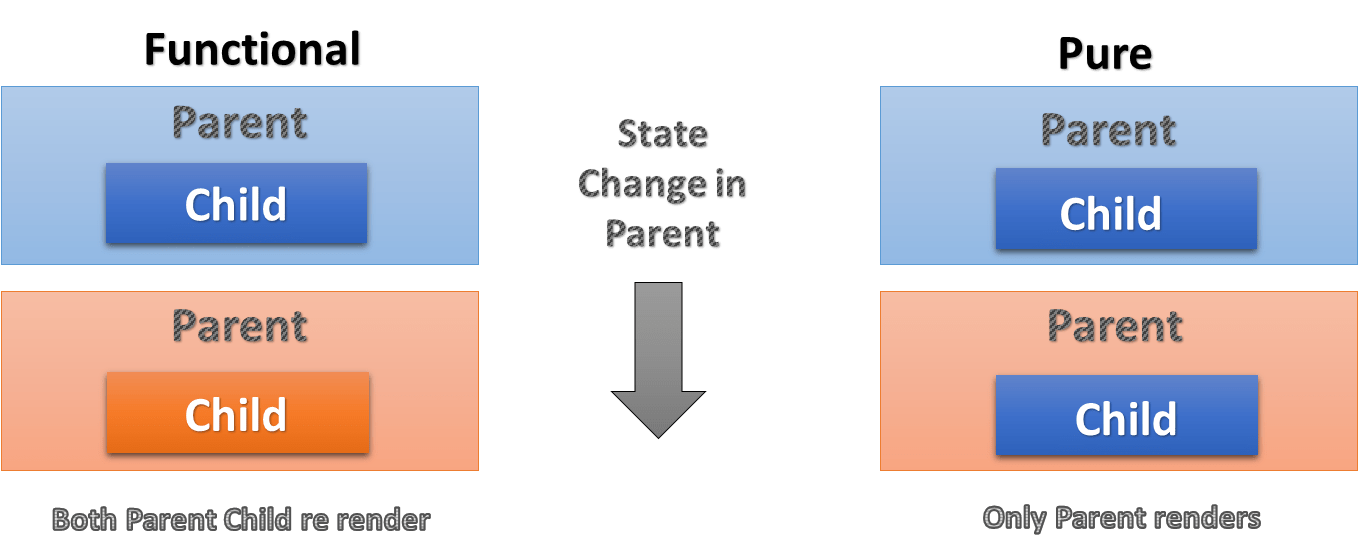
Performance Considerations
While Pure Components can significantly improve performance in many cases, there are scenarios where their use might not provide the expected benefits. It’s crucial to understand the limitations and potential pitfalls:
Getting Ready for a Business Analyst Job Interview? Check Out Our Blog on Business Analyst Interview Questions & Answer
Limitations of Pure Components
Despite their performance benefits, Pure Components have certain limitations that developers should be aware of. One key issue is their reliance on shallow comparison of props and state, which can lead to problems with complex data structures. If props or state are deeply nested objects or arrays, shallow comparison may fail to detect changes accurately, potentially causing UI inconsistencies when a component doesn’t re-render as expected. Additionally, Pure Components can be problematic when working with mutable objects, as changes within an object won’t be recognized unless the object’s reference changes, requiring careful management of state and props to ensure reliability. Moreover, Pure Components are not always necessary—especially in small applications where the performance gains are minimal. Overusing them can introduce unnecessary complexity without delivering noticeable improvements, so they are best used selectively, targeting specific performance bottlenecks.
Best Practices for Using Pure Components
Conclusion
Pure Components can significantly enhance performance in React applications by minimizing unnecessary re-renders and improving rendering efficiency, making them an important concept in Web Designing Training. However, they should be used thoughtfully and strategically to avoid potential pitfalls. When applied correctly especially in scenarios involving large, complex, or frequently updated user interfaces Pure Components contribute to building fast, maintainable, and high-performing applications. They are particularly valuable in cases where components depend heavily on immutable data structures and where performance optimizations can lead to a noticeably smoother user experience.