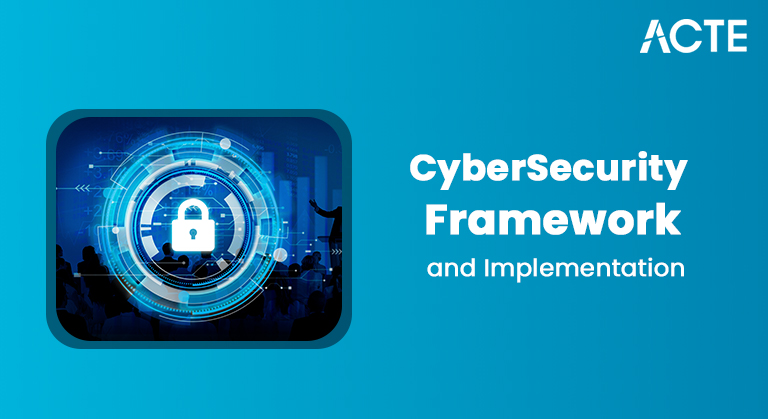
- Introduction to Node.js and Its Relevance
- Core Features of Node.js
- Node.js vs. Traditional Server-Side Technologies
- Key Frameworks and Libraries in Node.js
- Building RESTful APIs with Node.js
- Understanding Node.js Asynchronous Programming
- Deployment and Scaling with Node.js
- Career Opportunities for Node.js Developers
- Conclusion
Introduction to Node.js and Its Relevance
Node.js is a robust open-source runtime environment that allows developers to build scalable, high-performance web applications using JavaScript on the server side. Initially introduced by Ryan Dahl in 2009, Node.js has grown exponentially in popularity due to its ability to handle asynchronous events, enabling non-blocking operations. It utilizes the V8 JavaScript engine, developed by Google, which is known for its speed and efficiency in executing JavaScript code. Node.js provides an environment that supports event-driven, non-blocking I/O models, making it particularly suitable for building Web Designing and Development Courses web applications . Today’s relevance stems from the increasing demand for real-time applications, such as chat applications, gaming servers, collaborative tools, and IoT systems. Node.js is well-suited for developing applications requiring fast, efficient handling of numerous concurrent connections, making it a popular choice for startups and large companies.The platform is especially favored in full-stack JavaScript development, as it allows developers to use JavaScript on both the client and server sides, fostering consistency in the codebase and making JavaScript development more streamlined.
Core Features of Node.js
Node.js is known for several key features that make it stand out from other server-side technologies:
- Event-Driven and Asynchronous: Node.js uses an event-driven architecture where events trigger asynchronous callbacks. database queries non-blocking I/O model allows Node.js applications to handle multiple requests simultaneously without waiting for any single task to complete, significantly improving performance, especially in I/O-heavy applications.
- Single-Threaded Model: While traditional server-side languages use multi-threading, Node.js operates on a single-threaded event loop. This allows it to process a large number of requests in parallel without the overhead of managing multiple threads. This model works particularly well for applications involving many I/O operations, like database queries or API calls.
- Built-in Libraries and Modules: Node.js has a rich set of built-in libraries and modules that handle everything from HTTP requests and file operations to URL parsing and data compression. The Node.js package manager, npm, Front-End Developer Salary in India access to over a million packages, making it easy to find and install libraries that can speed up development.
- Cross-Platform: Node.js is cross-platform and runs on various operating systems, including Windows, Linux, and macOS. This flexibility makes it ideal for developing applications that can be used across different environments without additional modification.
- Scalable and Fast: The architecture of Node.js is optimized for performance and scalability. It can handle many concurrent connections with minimal resource usage. It benefits real-time applications requiring high scalability, such as online gaming, chat apps, and live data feeds.
Dive into Web Development by enrolling in this Web Developer Certification Courses today.
Node.js vs. Traditional Server-Side Technologies
- Traditional Server-Side Technologies (e.g., PHP, Java, Ruby): Traditional server-side technologies often use multi-threading to handle multiple requests. Each client request is assigned to a separate thread, leading to significant memory consumption and performance bottlenecks when dealing with numerous concurrent requests. These technologies perform better when handling CPU-bound tasks but struggle with I/O-bound tasks like database interactions or external API calls.
- Node.js: In contrast, Node.js uses a single-threaded event loop to handle all requests. This allows Node.js to handle more concurrent requests without the need for heavy resource consumption. Since Downloading Node Js and NPM operates on a non-blocking I/O model, it can handle thousands of connections simultaneously without waiting for any request to complete before moving on to the next. This is particularly beneficial in scenarios where the application involves many I/O operations and less CPU-intensive processing, like web servers, chat applications, or live-streaming platforms.
- Concurrency and Performance: Node.js outperforms traditional server-side technologies when it comes to concurrency. While conventional jQuery vs JavaScript rely on threading models that can be resource-intensive, Node.js’s non-blocking, event-driven model leads to better performance in high-concurrency situations, allowing it to process requests faster with fewer resources.
- Development Speed and Ecosystem: Through its npm package manager, one of the largest in the world, Node.js benefits from a vast ecosystem. Traditional server-side frameworks like Java or PHP also have their package managers, but the sheer volume of Node.js libraries and tools allows developers to build and deploy applications faster.
- Set Up the Project: To build a REST API in Node.js, the first step is to initialize a new project using npm. Then, install dependencies like Express to handle routing and middleware.
- Create Routes: With Express.js, creating RESTful routes is simple. For example, you can create endpoints like GET /users, POST /users, PUT /users/:id, and DELETE /users/:id. Each route is mapped to a controller function that performs the corresponding logic.
- Handle Requests: For each route, define functions that handle the request and response objects. Control Statements in Java functions should handle CRUD (Create, Read, Update, Delete) operations and interact with databases or other external services as needed.
- Connect to a Database: Most applications require persistent data storage. Node.js can be easily connected to databases like MongoDB, MySQL, or PostgreSQL to store and retrieve data.
- Return JSON Responses: One of the core principles of REST is that APIs should communicate using standard HTTP methods and data formats, typically JSON. In Express, you can send JSON responses using res.json().
- Error Handling: It’s essential to implement proper error handling in your API to handle cases like invalid input, authentication issues, or database queries errors. Use middleware to catch errors and send meaningful error messages.
- Testing and Documentation: Test the API using tools like Postman or Swagger and ensure it adheres to REST principles. Documentation is also vital to helping other developers understand how to interact with the API.
- Clustering : By default, Node.js runs on a single thread. To React Native Made Simple: Beginner’s Step-by-Step Guide advantage of multi-core systems, It supports clustering, which allows multiple instances of the application to run in parallel.
- Load Balancing : In a production environment, multiple instances of your Node.js app can be distributed across multiple servers or containers, with a load balancer managing traffic to ensure that requests are evenly distributed.
- Containerization : Using Docker, developers can containerize Node.js applications, making them easier to deploy, scale, and manage across different environments.
- Monitoring : Monitoring tools like New Relic, Prometheus, or Datadog can help track performance, errors, and other key metrics to ensure your application runs smoothly
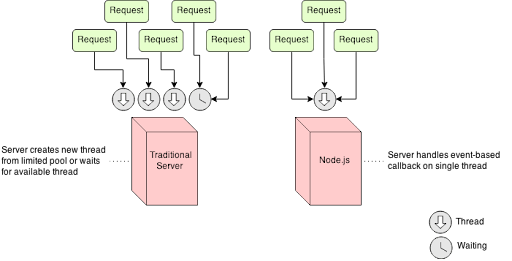
Gain in-depth knowledge of Web Development by joining this Web Developer Certification Courses now.
Key Frameworks and Libraries in Node.js
Node.js boasts a rich ecosystem of frameworks and libraries that simplify application development, each tailored for different use cases. One of the most popular frameworks is Express.js, widely used for building web applications and APIs. It offers minimal yet flexible features such as routing, middleware support, and template rendering, enabling developers to quickly get started while remaining highly extensible. A more modern and lightweight alternative to Express is Koa.js, created by the same team. Koa uses async/await natively for cleaner, more readable code and focuses on providing a minimal core that can be extended with additional libraries and middleware. Another powerful framework is NestJS, a TypeScript-based framework designed for building scalable server-side applications. It promotes modular development, encouraging clean and maintainable codebases, and is built on top of Express (or Fastify), with built-in support for features like dependency injection, decorators, and GraphQL/WebSocket integrations. For real-time, bidirectional communication, Web Developer Certification Courses is a popular JavaScript library, enabling live updates, chat systems, and notifications in Node.js applications. Mongoose is an Object Data Modeling (ODM) library for MongoDB, providing an intuitive API for managing MongoDB databases, schema validation, and model-based data management. Lastly, Passport.js serves as an authentication middleware that handles user authentication and authorization, supporting various strategies like OAuth, OpenID, and LDAP, making it easily integrable into Express applications.
Building RESTful APIs with Node.js
One of the most common use cases for Node.js is building RESTful APIs. REST (Representational State Transfer) is an architectural style for distributed systems, and Node.js, with its non-blocking I/O model, is an excellent choice for creating scalable, performant APIs. Here’s a high-level process for building RESTful APIs in Node.js:
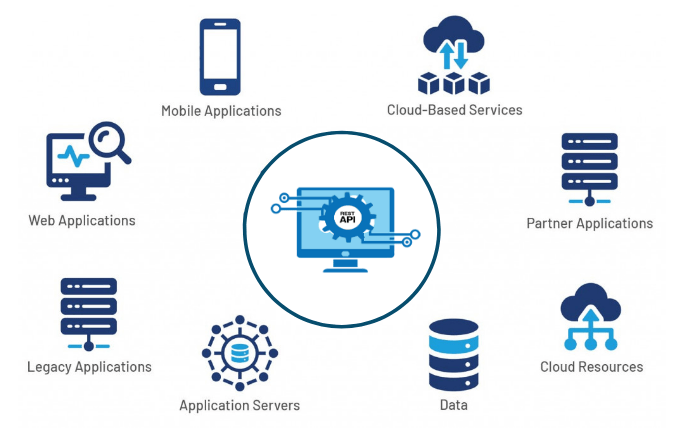
Understanding Node.js Asynchronous Programming
Node.js is designed around asynchronous programming, which helps improve resource efficiency, particularly when dealing with tasks like database queries or file handling. This approach allows multiple operations to run concurrently without blocking other tasks. Understanding how asynchronous programming works is essential for effectively using Node.js. In Node.js, asynchronous operations are often handled using Http Request Methods . A callback is a function that gets executed once an operation completes. For example, when reading a file, once the reading is finished, the callback function is triggered to process the data or handle any errors that may have occurred. Promises were introduced to simplify handling asynchronous tasks compared to callbacks. A Promise represents the eventual result of an asynchronous operation, either its successful completion or failure. Promises allow for cleaner, more readable code by enabling chaining, where you can handle success or failure through specific methods like .then() and .catch(). Introduced in ES2017, async/await builds on Promises by offering a way to write asynchronous code that looks and behaves more synchronously. With async/await, functions can pause at specific points, making the flow of logic easier to follow. This approach allows for better readability and simplifies debugging, as it eliminates the need for chaining methods and nested callbacks.
Get hands-on experience in web technologies by signing up for this Web Developer Certification Course now.
Deployment and Scaling with Node.js
Node.js applications can be deployed in various environments, including cloud services like AWS, Google Cloud, and Microsoft Azure, or on dedicated servers. Here are some strategies for scaling Node.js applications:
Want to ace your Web Development interview? Read our blog on Web Development Interview Questions and Answers now!
Career Opportunities for Node.js Developers
Node.js developers are in high demand as businesses increasingly require fast, scalable server-side technologies. Here are some potential career paths: Backend Developer Node.js is a popular choice for backend development, particularly for building APIs and microservices. Many companies seek Node.js developers for their backend systems. Full-Stack Developer Full-stack developers proficient in frontend (e.g., React, Angular) and backend (Node.js) development are highly sought for building end-to-end web applications. DevOps Engineer Node.js developers with a strong Downloading Node Js and NPM of deployment and scaling can pursue careers in DevOps, working to ensure seamless application deployment and infrastructure management. Real-Time Application Developer Given its strength in real-time communication, Node.js developers can focus on building applications like chat apps, multiplayer games, or live-streaming services. Freelance/Contract Developer With the popularity of Node.js, many businesses look for freelance developers to build custom solutions, which can be a rewarding path for independent developers.
Conclusion
Node.js has become an integral part of modern web development. Its asynchronous, event-driven architecture, rich ecosystem, and scalability make Web Designing Training ideal for building high-performance, real-time applications. With strong community support and vast career opportunities, Node.js is an exciting technology to learn and master.