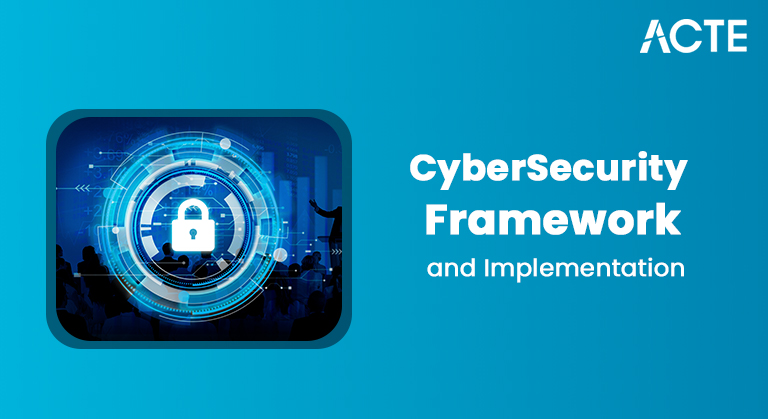
- Introduction to React.js
- Key Features of React.js
- Getting Started with React.js
- React Components and JSX
- State and Props in React
- React Hooks
- React Router
- React Best Practices and Performance Optimization
- Conclusion
Introduction to React.js
React.js is a powerful and popular open-source JavaScript library for building user interfaces, particularly single-page applications (SPAs). Initially developed by Facebook in 2011 and open-sourced in 2013, React has gained immense popularity due to its simplicity, scalability, and performance. It is not a framework but rather a library focused specifically on building user interfaces. At the core of React’s functionality is its component-based architecture. This means that UI elements are broken down into reusable components that are easy to manage, maintain, and scale. React components allow developers to build complex UIs from small, isolated pieces of code, which makes it easier to design and debug web applications. For those looking to enhance their skills in front-end technologies, our Web Designing & Development Courses offer a great starting point. React.js also utilizes a Virtual DOM (Document Object Model) to optimize rendering UI elements. This Virtual DOM ensures that only parts of the web page that need to be updated are rendered, reducing the number of changes to the real DOM and significantly improving the application’s performance. In summary, React allows developers to create fast, interactive, and scalable web applications by offering a declarative approach to UI development, efficient rendering through the Virtual DOM, and an ecosystem that promotes reusable components.
Key Features of React.js
React.js has several key features that make it a popular choice for modern web development:
- Component-Based Architecture: React divides the user interface into small, independent components, each managing its own state and logic. Components can be reused across the application, reducing redundancy and improving maintainability. Each component functions as a building block for the UI.
- Declarative UI: React makes it easy to design interactive UIs. Developers describe how the UI should look at any given point in time, and React updates the view based on changes to the application’s state. This declarative approach simplifies complex UI development.
- Virtual DOM: The Virtual DOM is a lightweight representation of the actual DOM. React uses it to minimize the updates made to the real DOM. For those interested in building efficient web applications and exploring How to Become a Web Developer understanding concepts like the Virtual DOM is essential. Whenever a change occurs, React first updates the Virtual DOM, calculates the difference (or “diff”), and then updates only the parts of the real DOM that need to be changed. This leads to better performance, especially in applications with complex UIs.
- Unidirectional Data Flow: React uses a one-way data flow, meaning data is passed down from parent to child components via props (properties). This ensures predictable behavior and makes tracking application changes easier. Unidirectional data flow also helps avoid issues such as circular dependencies and makes state management more transparent.
- JSX (JavaScript XML): JSX is a syntax extension for JavaScript that allows developers to write HTML-like code within JavaScript. JSX makes the Code more readable and expressive by enabling developers to describe the UI structure in a familiar format. Under the hood, JSX is transformed into JavaScript, making integrating React components into existing projects easy.
- React Developer Tools: React provides a set of powerful developer tools for inspecting React components, debugging state and props, and monitoring the component tree. These tools enhance the development workflow and help users understand how React applications behave.
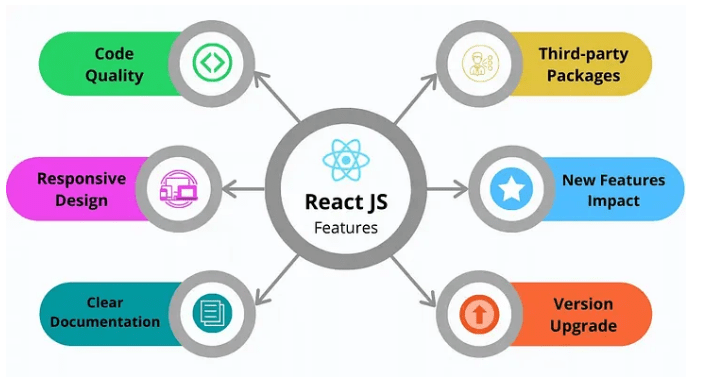
Getting Started with React.js
To get started with React.js, follow these steps:
Setting Up the Development Environment: The easiest way to set up a new React application is by using the Create React App tool, which automates the setup process. It comes pre-configured with all the necessary build tools and development dependencies.
You can create a new React project by running the following command:
- This will create a folder called my-app with all the files and configurations needed to start building your application.
- Basic Structure of a React App: Once you’ve set up your React app, you’ll find a basic file structure in your project directory. If you’re looking to apply your knowledge in real-world scenarios, exploring some full stack project ideas can be a great way to get started. The most important file is src/App.js, which contains the main React component for your application. The public/index.html file is where the root div of your app will be injected into the DOM.
- This will open your app in a browser, and any changes to your Code will automatically be reflected in real time without needing to refresh the page.
- React Syntax and Structure: React’s syntax differs slightly from traditional HTML and JavaScript. Components in React are typically written as JavaScript functions or classes that return JSX to define the UI structure. JSX allows you to mix HTML-like code with JavaScript logic, making it easy to create dynamic user interfaces.
Running the React Application: After setting up your project, you can run the development server by running the following:
React Components and JSX
React components are the heart of React.js. A component is a self-contained unit that encapsulates its logic, state, and rendering process. Understanding the Scope of Web Development helps put the role of React components into perspective, especially as front-end technologies continue to evolve. Components can be functional or class-based, but functional components are now more commonly used due to the introduction of React Hooks.
Functional Components vs. Class ComponentsFunctional Components: These are simpler components that are written as JavaScript functions. Functional components receive props as arguments and return JSX. With the introduction of hooks in React 16.8, functional components can now manage state and handle lifecycle events, making them as powerful as class components.
Example:- function Welcome(props) {
- return Hello,
- {props.name};
- }
- Class Components: These are ES6 classes that extend React. Each component requires a render() method to return JSX. Class components can hold and manage state and lifecycle events using the componentDidMount and shouldComponentUpdate methods. Example:
- Class Welcome extends React.
- Component {
- render() {
- return Hello, {this.props.name};
- }
- }
- const element = Hello, world!;
- JSX also allows you to embed JavaScript expressions within curly braces {}:
- const user = ‘John’;
- const element = Hello,
- {user}!;
- function Greeting(props) {
- return Hello, {props.name}!;
- }
- useEffect(() => {
- //Code to run on component mount
- },
- [dependencies]);
- Code Splitting: Code splitting allows you to load parts of your application only when needed, improving initial load time and overall performance. React supports code splitting using React.lazy() and Suspense.
- Memoization: Use React. Memo to optimize functional components by memorizing them. This prevents unnecessary re-renders when props don’t change.
- Lazy Loading: React supports lazy loading, which defers the loading of components until they are needed. This improves performance by reducing the initial JavaScript bundle size.
- Avoiding Unnecessary Renders: Use shouldComponentUpdate in class components or React to avoid unnecessary renders memo in functional components.
JSX Syntax: JSX allows you to write HTML-like code within JavaScript. Using JSX in React is not mandatory, but it provides a cleaner and more readable syntax. JSX is eventually compiled into React.createElement() calls.
Example:Excited to Obtaining Your web developer Certificate? View The web developer course Offered By ACTE Right Now!
State and Props in React
In React, data is passed between components through props and state.
Props: Props (short for properties) are read-only and used to pass data from a parent component to a child component. They are immutable, meaning the child component cannot change them.
Example:State: State is used to store data that changes over time. Unlike props, the state is mutable and can be modified within a component. The state typically tracks user interactions, form inputs, or any other dynamic data in an app.
Example using hooks:The Counter function is a simple React component that uses a state hook to track the number of times a button is clicked. It initializes a state variable count with a starting value of 0 using useState. For those aiming to build real-world projects and validate their skills, earning a Web Developer Certification can be a valuable step. Inside the returned JSX, it displays the current count in a paragraph and includes a button labeled “Click me.” When the button is clicked, it triggers an event handler that updates the count by incrementing it by one using the setCount function. This causes the component to re-render and display the updated count. However, the code contains a few syntax errors, such as estate(0) instead of useState(0) and set count instead of setCount. Correcting these issues will ensure the component functions as intended.
Interested in Pursuing Web Developer Master’s Program? Enroll For Web developer course Today!
React Hooks
React hooks allow functional components to manage state and side effects (e.g., data fetching or event listeners). Introduced in React 16.8, hooks have become the standard for writing React components.
useState: The useState hook allows you to add state to functional components.
const [state, setState] = useState(initialState);
useEffect: The useEffect hook handles side effects, such as fetching data or subscribing to a service, within functional components.
Custom Hooks: Custom hooks allow you to reuse logic across components. A custom hook is a function that uses built-in hooks like useState and useEffect. If you’re preparing for job opportunities, having a well-crafted Full Stack Developer Resume can make a strong impression. React Router: React Router is a popular library for navigation in React applications. It allows you to create single-page applications with multiple views by defining routes and linking them to different components.
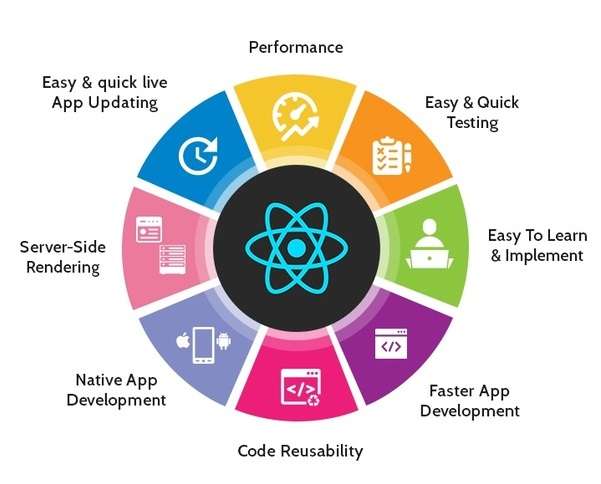
React Router
React Router: You can install React Router with the following:
#npm install react-router-dom
Creating Routes: Define routes using
Nested Routing: React Router also supports nested routing, which allows you to create hierarchical navigation structures.
React Router’s nested routing feature enables the creation of structured, hierarchical navigation. This allows you to define routes inside parent routes, making it ideal for layouts with shared components like headers or sidebars. Before diving into routing and other advanced features, it’s important to complete initial setup steps like Downloading Node.js and NPM which are essential for running React projects. For example, in a dashboard, the main layout can remain constant while different subcomponents render based on the nested route. This helps maintain consistent UI and improves the flow of your app. Nested routes keep the structure clean and scalable, especially when dealing with complex applications. By using components like
React Best Practices and Performance Optimization
Conclusion
React.js has revolutionized the way we build modern web applications by offering a component-based architecture, declarative UI, and powerful tools for performance optimization. From its efficient Virtual DOM to the flexibility of JSX and React Hooks, React empowers developers to create interactive, scalable, and maintainable user interfaces. For those looking to strengthen their front-end development skills, our Web Designing Training provides a solid foundation in modern design principles and practices. Whether you’re using it for single-page applications or full-stack development, React’s vast ecosystem and community support continue to make it a top choice for web development. By understanding key concepts such as state, props, and routing, and implementing best practices, developers can harness the full potential of React to build fast and dynamic applications.