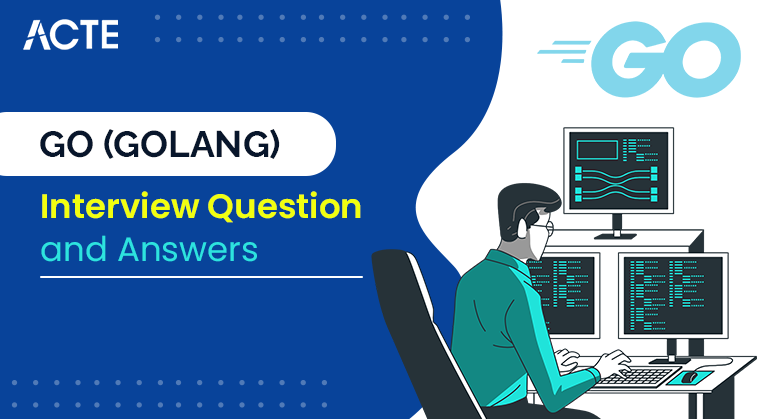
Go [Golang] Interview Question and Answers [ FRESHERS ]
Last updated on 10th Nov 2021, Blog, Interview Questions
The Go programming language, or Golang, is an open-source programming language similar to C but is optimized for quick compiling, seamless concurrency, and developer ease of use.Whether you’re preparing for a Google job interview or just want to remain a cutting edge developer, Go is the right choice for you. Today, we’ll help you practice your Go skills with 50 of the most important Go questions and answers.
1. What is Go programming language, and why used?
Ans:
Go is the modern programming language developed by a Google. It is designed to simple, efficient, and reliable. It is often used for the building scalable and highly concurrent applications. It combines the ease of use of high-level language with the performance of low-level language. Go has built-in support for the concurrency, making it ideal for the developing applications that require dealing with the multiple tasks simultaneously. Overall, Go is used for developing fast, efficient, and robust software, especially field of web development and cloud computing. T
2. How do implement concurrency in Go?
Ans:
In Go, concurrency is implemented using the goroutines and channels. Goroutines are the lightweight threads that can be created with go keyword. They allow the concurrent execution of functions.
3. How do handle errors in Go?
Ans:
In Go, errors are the handled using the error type. When function encounters an error, it can return the error value indicating the problem. The calling code can then check if error is nil. If not, it handles error appropriately. Go provides the built-in panic function to handle the exceptional situations. When the panic occurs, it stops execution of the program and starts unwinding the stack, executing the deferred functions along way. To recover from the panic, can use the recover function in the deferred function. This allows to handle the panic gracefully and continue program execution.
4. How do implement interfaces in Go?
Ans:
Interfaces are the implemented implicitly in Go. This means that don’t need to explicitly declare that the type implements an interface. Instead, if type satisfies all methods defined in the interface, it is considered to implement that interface. This is done by a first defining interface type using the type keyword followed by interface name and the methods it should contain. The next step is creating a struct type or any existing type that has all methods required by the interface. Go compiler will automatically recognize that type as implementing interface.
5. How do optimize performance of Go code?
Ans:
- Minimize memory allocations: Avoid the unnecessary allocations by reusing an existing objects or using buffers.
- Use goroutines and channels efficiently: Leverage power of concurrent programming, but ensure the proper synchronization to avoid race conditions.
- Optimize loops and conditionals: Reduce number of iterations by simplifying logic or using the more efficient algorithms.
- Profile your code: Use the Go’s built-in profiling tools to identify the bottlenecks and hotspots in code.
6. What is “init” function in Go?
Ans:
The “init” function is the special function in Go that is used to initialize the global variables or perform any other setup tasks needed by the package before it is used. The init function is called automatically when package is first initialized. Its execution order within the package is not guaranteed.
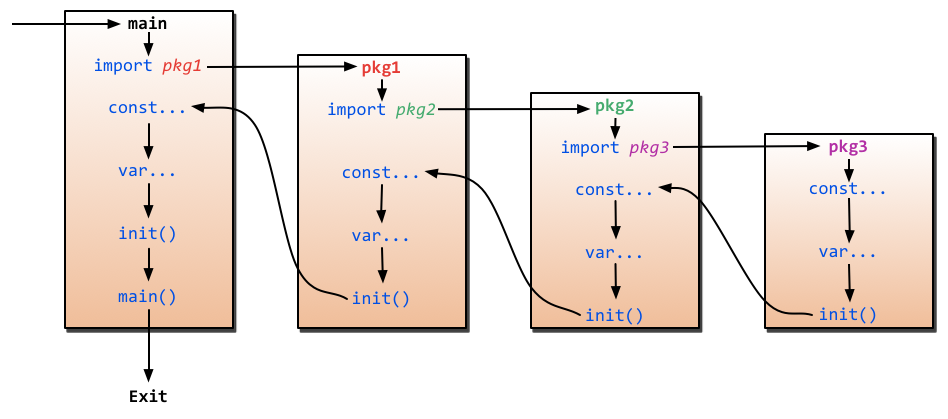
7. What are dynamic and static types of declaration of variable in Go?
Ans:
The compiler must interpret a type of variable in the dynamic type variable declaration based on value provided to it. The compiler does not consider it necessary for variable to be typed statically. Static type variable declaration assures compiler that there is only a one variable with provided type and name, allowing compiler to continue compiling without having all of variable’s details. A variable declaration only has meaning when a program is being compiled; compiler requires genuine variable declaration when the program is linked.
8. What is syntax for declaring variable in Go?
Ans:
In Go, variables can be declared using var keyword followed by a variable name, type, and optional initial value. For example: var age int = 29 Go also allows the short variable declaration using := operator, which automatically infers the variable type based on assigned value. For example: age := 29 In this case, type of the variable is inferred froma value assigned to it.
9. What are Golang packages?
Ans:
Go Packages (abbreviated pkg) are the simply directories in Go workspace that contain Go source files or other Go packages. Every piece of the code created in the source files, from the variables to functions, is then placed in the linked package. Each source file should be associated with the package.
10. What are different types of data types in Go?
Ans:
Numeric types : Integers, floating-point, and a complex numbers.
Boolean types : Represents the true or false values.
String types : Represents the sequence of characters.
Array types : Stores the fixed-size sequence of elements of the same type.
Slice types : Serves as flexible and dynamic array.
Struct types : Defines the collection of fields, each with the name and a type.
11. How do create a constant in Go?
Ans:
To create the constant in Go, can use the const keyword, followed by a name of the constant and its value. The value must be the compile-time constant such as string, number, or boolean. Here’s an example: const Pi = 3.14159
12. What data types does Golang use?
Ans:
- Slice
- Struct
- Pointer
- Function
- Method
- Boolean
- Numeric
- String
- Array
- Interface
- Map
- Channel
13. Distinguish unbuffered from buffered channels.?
Ans:
The sender will block on an unbuffered channel until receiver receives a data from channel, and the receiver will block on channel until the sender puts data into channel. The sender of buffered channel will block when there is a no empty slot on channel, however, the receiver will block on channel when it is empty, as opposed to unbuffered equivalent.
14. Explain string literals?
Ans:
concatenated string constant. Raw string literals and interpreted string literals are two types of string literals. Raw string literals are the enclosed in backticks (foo) and contain uninterpreted UTF-8 characters. Interpreted string literals are the strings that are written within a double quotes and can contain any character except the newlines and unfinished double-quotes.
15. What is Goroutine and how do stop it?
Ans:
A Goroutine is the function or procedure that runs concurrently with the other Goroutines on dedicated Goroutine thread. Goroutine threads are lighter than ordinary threads, and most Golang programs use the thousands of goroutines at a same time. A Goroutine can be stopped by passing it signal channel. Because Goroutines can only respond to the signals if they are taught to check, must put checks at a logical places, such as at top of the for a loop.
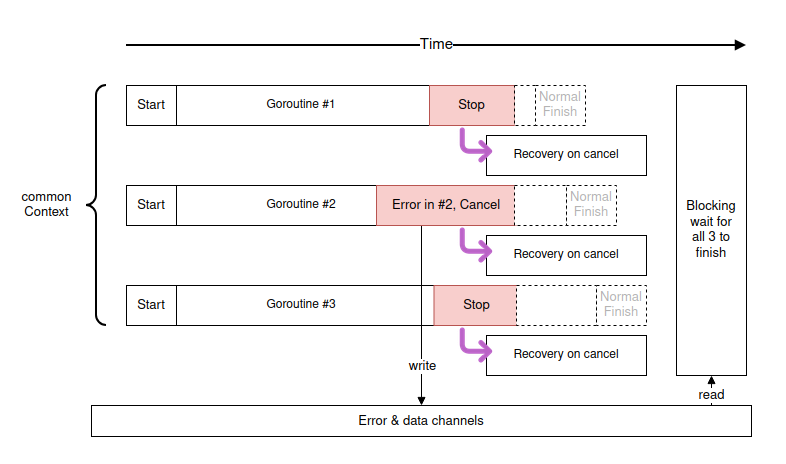
16. What is syntax for creating a function in Go?
Ans:
To create the function in Go, need to use keyword func, followed by a function name, any parameter(s) enclosed in the parentheses, and any return type(s) enclosed in parentheses. The function body is enclosed in the curly braces {}. Declare the function called add that takes a two parameters, x and y, and returns sum as an int.
17. What are components in for loop in Go?
Ans:
The most commonly used loop is a for loop.
It has three components:
- the initialization statement,
- the condition statement,
- the post statement.
18. What is if statement in Go?
Ans:
If statement in Go is a straightforward and similar to the other programming languages. The if keyword is followed by the condition enclosed in the parentheses, and body of the statement is enclosed in the curly braces.
19. What are some benefits of using Go?
Ans:
- On single machine, a big Go application can be compiled in the matter of seconds.
- Go provides the architecture for software development that simplifies the dependency analysis while avoiding much of complexity associated with C-style programs, like files and libraries.
- Because there is a no hierarchy in Go’s type system, no work is wasted describing relationships between types. Furthermore, while Go uses static types, the language strives to make types feel the lighter weight than in a traditional OO languages.
20. How do create a pointer in Go?
Ans:
Can use the & symbol, followed by the variable to create the pointer in Go. This returns a memory address of the variable. For example, if have a variable num of type int, can create a pointer to num like this: var num int = 42 var ptr *int = &num
Here, ptr is the pointer to num. can use the * symbol to access value stored in the memory address pointed by the pointer. For instance, *ptr will give the value 42. Pointers are useful for the efficient memory sharing and passing references between the functions.
21. What is syntax for creating a struct in Go?
Ans:
Need to define the blueprint for the struct, which may consist of the fields of different data types. The blueprint for struct is defined using the ‘type’ keyword, followed by the namewant to give struct. Then use the ‘struct’ keyword, followed by the braces (‘{}’) where list the fields, each with the name and a data type separated by comma. For instance, syntax for creating the struct named Person with the fields name, age, and job of the string, integer, and string data types, respectively,
22. How do create an array in Go?
Ans:
Creating array in Go is simple. First, need to declare the array by specifying type and size. var myArray [size]datatype Replace size and datatype with size and data type want to use for array. After declaring the array, can then initialize it by assigning the values to each index. can also access and modify the elements of array using their index number.
23. How will perform inheritance with Golang?
Ans:
Golang does not support the classes, hence there is no inheritance. However, may use composition to imitate the inheritance behavior by leveraging an existing struct object to establish initial behavior of a new object. Once new object is created, the functionality of original struct can be enhanced.
24. How do create a slice in Go?
Ans:
First need to define the variable of type slice using make() function. The make() function takes a two arguments: the first is a type of the slice want to create (for example, []string for the slice of strings) and second is a length of the slice. The length of slice is not fixed and can be changed dynamically as the elements are added or removed.
Here’s example to create slice of strings with the length of 5: mySlice := make([]string, 5) can access and modify elements in the slice using their index.
25. What is difference between array and slice in Go?
Ans:
In Go, an array is the fixed-length sequence of the elements of the same type. Once an array is a defined, the length cannot be changed. On other hand, a slice is a dynamically-sized, flexible view of underlying array. It is created with the variable length and can be resized. Slices are typically used when need to work with the portion of an array or when want to pass a subset of an array to function. Slices provide a more flexibility and are widely used in the Go for convenience and efficiency in the managing collections of data.
26. How do create a map in Go?
Ans:
Can use the make keyword, followed by map keyword and data types for a key and value. The syntax would be make(map[keyType]valueType). For example, to create the map of string keys and integer values, would use make(map[string]int). can assign the values to a map using the bracket notation like mapName[key] = value. To access the values, simply use mapName[key].
27. How do iterate through a map in Go?
Ans:
To iterate through the map in Go, can use a for loop combined with range keyword. ,A key represents a key of each key-value pair in the map, and value represents a corresponding value. Can perform the any desired operation within loop. The range keyword automatically iterates over a map and gives access to its keys and values.
28. What are looping constructs in Go?
Ans:
There is only a one looping construct in Go: for loop. The for loop is made up of the three parts that are separated by semicolons:
- Before loop begins, the Init statement is run. It is frequently the variable declaration that is only accessible within scope of the for a loop.
- Before each iteration, condition statement is evaluated as Boolean to determine if loop should continue.
- At end of each cycle, the post statement is executed.
29. What is channel in Go?
Ans:
In Go, channel is the data structure that allows the goroutines (concurrent functions) to communicate and synchronize with each other. It can be thought of as a conduit through which can pass values between the goroutines. A channel has specific type that indicates a type of values that can be sent and received on it. Channels can be used to implement the synchronization between the goroutines and data sharing. They provide safe and efficient way to coordinate the flow of information, ensuring that a goroutines can send and receive data in the controlled and synchronized manner.
30. How do create channel in Go?
Ans:
Can use the built-in make function with chan keyword to create the channel in Go. Here’s an example: ch := make(chan int) In above code, a channel called ch has been created that can transmit integers. This channel can be used to be send and receive data between the goroutines. By default, channels are the unbuffered, meaning that sender blocks until receiver is ready. Can also create buffered channels by providing the buffer capacity as a second argument to make function. Channels are the powerful synchronization mechanism in Go, allowing the safe communication and coordination between the concurrent processes.
31. How do close a channel in Go?
Ans:
The close() function is used to close the channel in Go. The function is used to indicate no more values will sent through the channel. Once the channel is closed, any subsequent attempt to send data through it will result in the runtime panic. However, receiving from closed channel is still possible. With built-in v, ok := <-ch syntax, can receive the values from the closed channel. The ok boolean flag will be set to be false if channel is closed. It's important to note that closed channels should be used for signaling and not for synchronization.
32. How do range over a channel in Go?
Ans:
To range over the channel in Go, a for loop with the range keyword can be used. This allows to iterate over all the values sent on channel until it is closed. When using range, loop will continue until the channel is closed or until no more values are the available.
33. How do handle panics and recover from them in Go?
Ans:
To handle the panics and recover from them in Go, built-in panic() and recover() functions can be used. When error occurs, panic() is called and program execution stops. can use the defer statement to call the recover(), which stops panic and resumes execution from a point of nearest enclosing function call, after all the deferred functions have been run. It’s important to note that should only use panic and recover for an exceptional cases such as when program encounters unexpected errors. It’s not meant to handle normal control flow or act as replacement for checking errors. Using these functions properly can help to ensure programs remain robust and reliable.
34. Explain defer statement in Golang. ?
Ans:
The defer statement in the Golang is used to postpone the execution of function until the surrounding function completes. It is often used when want to make sure some cleanup tasks are performed before an exiting a function, regardless of errors or the other conditions.
35. How do create and use package in Go?
Ans:
- Create the directory for package. Use a meaningful name that represents a package’s functionality.
- Inside directory, create a Go source file. The name of file should match directory name.
- Add code to source file, defining functions, variables, and types that make up the package.
- At the top of source file, add the package statement with the name of package.
- To use package in another Go program, import it by using package name, followed by a directory path.
36. What is difference between package and module in Go?
Ans:
Feature | Package | Module | |
Definition |
A package is a collection of Go source files in the same directory sharing a common package declaration. |
A module is a collection of related Go packages that are versioned together | |
Scope | Limited to a single directory. | Can span multiple directories and may consist of multiple packages. | |
Dependency Management | Dependencies are managed using the import statement, and there is no explicit versioning within a package. | Introduced to address versioning and dependency management at a higher level. Uses go.mod file to specify module information and dependencies. |
37. How do create a custom type in Go?
Ans:
Custom types are the useful for improving a code readability and creating abstractions. To create the custom type in Go, can use the type keyword, followed by the name of type and the underlying type it should be based on. For example, if want to create a custom type called the Person based on string type, would use type Person string. can also create a custom type based on the struct or any other built-in type in Go. Once the custom type is defined, can use it like any other data type in ya code.
38. What is type casting in Go?
Ans:
In Go, type casting is a done using the Type(value) syntax. To convert the value to a specific type, need to mention the desired type in parentheses, followed bya value want to convert. For example, if want to convert an integer to the floating-point number, can use the syntax float64(42). Similarly, if want to convert the floating-point number to an integer, can use the syntax int(3.14).
39. How do use “blank identifier” in Go?
Ans:
The blank identifier, represented by the underscore character, is used in Go as the placeholder for a variable or value that is not needed or used in a code. It allows the programmers to ignore an unused variable without generating the compiler warning. If function returns the multiple values but only one of them needs to be used, can use the blank identifier to discard others. Additionally, it can be used in the variable declarations to indicate that variable is not needed for a code to compile.
40. How do embed a struct in Go?
Ans:
To embed the struct in Go, only have to declare a field in a struct and assign it value of another struct. This field with the struct value is then known as an embedded struct. Can also access the embedded struct’s fields by using the dot notation with the parent struct. This allows to reuse the fields and methods of embedded struct without explicitly declaring them in a parent struct. Additionally, can use the anonymous fields to embed a struct without specifying the name for the field. This helps to simplify a code and make it more concise.
41. How do create and use function closure in Go?
Ans:
- Closures are useful for creating the private variables and to bind the values to callback functions.
- To create function closure in Go, first define the function containing the variables want to access and return it. Then, assign a function to a variable. This will create the closure where the variables within function are accessible to the assigned variable and any functions returned by it.
- To use closure, call the assigned variable, which will execute a contained function. The inner variables will retain values between the function calls.
42. How do use “select” statement in Go?
Ans:
The “select” statement in Go is used for the multiplexing channels, allowing Go program to handle the multiple channels at once. With “select,” can send and receive data from the multiple channels which allows for an efficient communication between goroutines.The basic syntax of “select” allows to specify the multiple channels and assign their input and output to the different cases. It can also be used with default case that executes if no other case is ready. The “select” statement allows for a powerful concurrent programming in Go and facilitates simpler and more efficient communication between the independently executing goroutines.
43. How do use “sync/atomic” package to perform atomic operations in Go?
Ans:
To use “sync/atomic” package in Go, need to import it into the code using the import statement. Once done, package provides functions and types to perform atomic operations on the variables. To perform atomic operation, define the variable of the desired type using one of atomic types provided by a package (e.g., int32, int64). Then, use atomic functions like a atomic.LoadXXX and atomic.StoreXXX to atomically load and store values. These atomic operations ensure that operations on variable are performed in the atomic manner, avoiding race conditions and guaranteeing a data integrity.
44.How do use “context” package to carry around request-scoped values in Go?
Ans:
- Import “context” package: import “context”.
- Create the new context with context.Background() function: ctx := context.Background().
- Add values to a context using the WithValue method: ctxWithValue := context.WithValue(ctx, key, value).
- To retrieve value from the context, use Value method: val := ctxWithValue.Value(key).
45. How do use “net/http” package to build HTTP server in Go?
Ans:
To build HTTP server in Go, can use the built-in “net/http” package that provides the range of functions and methods to handle HTTP requests and responses. To get started, define handler function that takes in HTTP response writer and request. Then, register handler function with the “http” package that handles incoming requests and invokes handler function. With the “http” package, can specify a port number, listen for incoming requests, and gracefully shut down server. Overall, “net/http” package offers the simple and effective way to quickly build the HTTP servers in Go.
46. How do use “encoding/json” package to parse and generate JSON in Go?
Ans:
- To use “encoding/json” package in Go have two main functions at disposal: “json.Marshal” and “json.Unmarshal”.
- Can use “json.Marshal” to generate the JSON from Go data structure. This function takes a Go data and encodes it into the JSON string can then use as needed.
- Meanwhile, can use “json.Unmarshal” to parse JSON and convert it into the Go data. This function takes the JSON string and decodes it into Go data structure. Both of these functions require to define struct tags on Go data structure to specify how JSON should be formatted.
47. How do use “reflect” package to inspect the type and value of variable in Go?
Ans:
- To use “reflect” package in Go, import it using import “reflect”. can then inspect the type and value of the variable using a reflect.TypeOf() and reflect.ValueOf() functions, respectively.
- For example, can use reflect.TypeOf(x) to inspect a type of a variable x. This will return the reflect.Type object. Similarly, can use reflect.ValueOf(x) to inspect the value of x. This will return the reflect.Value object.
- The methods provided by objects can be used to further inspect and manipulate a variable’s type and value.
48. How do use “testing” package to write unit tests in Go?
Ans:
To write unit tests in Go using “testing” package, first need to create the test file with a name that ends in _test.go. In this file, write functions that start with Test, followed by a name of the function want to test, for example, TestAddition(). Then, inside a test function, write assertions using t parameter that represents testing.T type. The testing package includes the variety of useful functions, like Errorf() and Fatal(), to help write better tests.
49. How do use “errors” package to create and manipulate errors in Go?
Ans:
- Import “errors” package into the code: import “errors”.
- Create the new error by using errors.New() function and passing in a string describing error: err := errors.New(“Something went wrong”).
- Manipulate error by checking its value. And can compare the error with another error using errors.Is() function or retrieve error message by calling err.Error().
50. How do use “net” package to implement networking protocols in Go?
Ans:
The “net” package is the built-in Go library that provides a basic networking functionality. It allows for the communication over network protocols such as TCP, UDP, and Unix domain sockets. To use this package, import it into the code and then create network connections using functions provided by “net”. For example, “net.Dial()” is used to establish the TCP connection to a server.
51. How do use “time” package to handle dates and times in Go?
Ans:
To use time” package in Go, first import it with the import “time”. This package provides the functions and types to handle dates and times.
A few useful methods to perform the various operations:
- To get a current time, use time.Now().
- To format it, use Format() method, specifying the desired layout or format string like time.Now().Format(“2006-01-02 15:04:05”).
- To parse a string into the time value, use time.Parse() and provide a layout of the string.
52. How do use “math” and “math/rand” packages ?
Ans:
The “math” and “math/rand” packages are the built into Go and provide extensive mathematical and statistical operation functionalities. The “math” package, for instance, offers the fundamental mathematical constants like Pi, mathematical functions for trigonometry, exponential, and logarithmic, as well as rounding and floating-point operations. The “math/rand” package is a specially designed to generate the random numbers based on the pseudo-random number generator algorithms. This package can be used for a statistical simulations or for generating random passwords and encryption keys.
53. What is “crypto” package to perform cryptographic operations in Go?
Ans:
The “crypto” package in Go provides the set of tools for performing cryptographic operations. To use it, need to import the package using: import “crypto” can then use the various functions provided by a package for cryptographic operations like hashing, encryption, and decryption. For example, to generate SHA-256 hash of message.
54. What is “os” package to interact with operating system in Go?
Ans:
In Go, “os” package provides the way to interact with the operating system. can use the package to perform the operations like creating, opening, reading, writing, and deleting files and directories, among other things. To use the “os” package, need to import it using import statement, after which can access its functions and types. Some of common functions that can use are os.Open(), os.Create(), os.ReadDir(), os.Mkdir(), and os.RemoveAll(), among others.
55. What does “bufio” package to read and write buffered data in Go?
Ans:
- To use bufio package in Go, first need to import the package using a statement import “bufio”. The bufio package provides the buffered I/O operations for reading and writing data.
- To read buffered data, create the new bufio.Reader object by passing an io.Reader object to bufio.NewReader() function. Then use the methods like ReadString(), ReadBytes(), or ReadLine() to read the data.
- To write a buffered data, create the new bufio.Writer object by passing an io.Writer object to bufio.NewWriter() function.
56. How does “strings” package to manipulate strings in Go?
Ans:
- Use strings.Contains(str, substr) to check if string contains the specific substring.
- strings.HasPrefix(str, prefix) and strings.HasSuffix(str, suffix) can be used to check if string starts or ends with the specific prefix/suffix.
- strings.ToLower(str) and strings.ToUpper(str) can be used to convert the string to lowercase or uppercase.
- strings.Replace(str, old, new, n) replaces all the occurrences of substring with a new substring.
- strings.Split(str, sep) splits the string into slice of substrings based on separator.
57. How “bytes” package to manipulate byte slices in Go?
Ans:
To use “bytes” package in Go to manipulate a byte slices, import package using import statement:
import “bytes”
Once package is imported, can perform the various operations on byte slices. Some commonly used functions in “bytes” package include:
Join : Joins the multiple byte slices into single byte slice.
Split : Splits a byte slice into the multiple byte slices based on a separator.
Contains : Checks if byte slice contains another byte slice.
58. How “encoding/binary” package to encode and decode binary data in Go?
Ans:
- In Go, “encoding/binary” package is used to convert the binary data to Go data types and vice versa. To encode a binary data, need to create a buffer object using the “bytes” package. Next, use appropriate encoding function, such as binary.Write(), to encode binary data into buffer.
- To decode binary data, create the buffer object. Then, use appropriate decoding function, such as binary.Read(), to read binary data intoa buffer. The data can then be extracted from a buffer using appropriate Go data type.
59. What is “compress/gzip” package to using gzip algorithm in Go?
Ans:
Compressing and decompressing a data using gzip algorithm entails following steps:
- Import the package into the code with import “compress/gzip”
- To compress data, create the new gzip.Writer by passing io.Writer as its parameter.
- Write a data to be compressed using Write method of a gzip.Writer.
- Flush and close gzip.Writer to be finalize compression.
60. What “database/sql” package to access SQL database in Go?
Ans:
Accessing the SQL database in Go with “database/sql” package involves following steps:
- Import database/sql package and specific driver package for a database want to connect to.
- Open the connection to database using appropriate driver’s Open function.
- Use DB object returned by Open function to execute SQL queries or statements.
61. What is “html/template” package to generate HTML templates in Go?
Ans:
To begin using “html/template” package in Go, need to import it into the code and create a new template using ParseFiles() function. This takes a string of one or more file paths as argument. Once have the template, can execute it using the Execute() method, passing in the Writer object as well as any data want to render in a template. When defining the template, use special syntax to indicate where want values to be dynamically inserted. For example, {{.}} for a current value and {{range}} for iterating over the collection.
62. Explain byte and rune types and how represented?
Ans:
- A byte is the unit of data that typically consists of a 8 bits and is used to represent single character, numeric value or instruction in computer systems. It’s the smallest unit of data computer system can address and is commonly represented in the hexadecimal notation.
- A rune, on other hand, is a Unicode character that represents single code point or a symbol in a script like alphabet, digits, and punctuation. It can be as small as a byte or as large as 4 bytes and is used to represent the characters from a different languages and scripts.
63. Go program on Linux and to compile it for both Windows and Mac. Is it possible?
Ans:
Yes, it is possible to compile the Go program on Linux for both the Windows and Mac. The Go language provides the cross-compilation feature that allows to build binaries for a different operating systems and architectures. To compile Go program for Windows, can use GOOS=windows environment variable. For example, run GOOS=windows go build the main.go to generate a Windows executable. Similarly, to compile for a Mac, use GOOS=darwin. This flexibility makes it simple to target the multiple platforms from Linux development environment. Just remember to test program on a target platform to ensure compatibility.
64. How can compile Go program for Windows and Mac?
Ans:
To compile Go program for Windows and Mac, y need to cross-compile it from development environment can use the GOOS and GOARCH environment variables to specify a target operating system and architecture. For Windows, set GOOS to “windows” and for a Mac, set it to “darwin.” Use “amd64” as the architecture for the 64-bit systems.
For Windows : GOOS=windows GOARCH=amd64 go build -o myprogram.exe
For Mac : GOOS=darwin GOARCH=amd64 go build -o myprogram
65. How can format Go source code in idiomatic way?
Ans:
There are the several best practices to format Go source code idiomatically. They ensure that code is a more maintainable and readable.
- Use gofmt command to automatically format Go code according to language specification.
- Keep the lines short, preferably less than 80 characters. This makes code easier to read and review.
- Use the braces (curly brackets) on new line to define control structures.
- Use the descriptive names for variables, constants, and functions in the concise and readable manner.
66. Can change specific character in string?
Ans:
Yes, can change the specific character in the string by determining its position and assigning the new value to that position. For example, in Python can use indexing to access the individual characters in a string. The index starts at 0 for a first character, 1 for a second character, and so on. Once have determined position of a character want to change, can reassign it a new value using either slicing or concatenation.
67. How can y concatenate string values?
Ans:
Concatenating string values is a simply the process of linking the two or more strings together to form a one longer string. To concatenate string values, can use the “+” operator or string interpolation feature available in the some programming languages like the Python, JavaScript, Java, C#, etc.
68. Explain backing array of slice value?
Ans:
In Go, backing array of a slice value is the hidden data structure that holds elements of the slice. When create a slice from an existing array or slice, slice value itself only holds the pointer to starting element of a slice in backing array, along with length and capacity of the slice. This allows the efficient manipulation of slice without needing to copy underlying data. If slice is modified and exceeds its capacity, new backing array may be allocated and elements will be copied to a new array. It’s important to understand concept of the backing array to avoid the unexpected behavior when working with slices in Go.
69. Explain Golang map type and its advantages.?
Ans:
Golang’s map type is the powerful data structure that allows to store key-value pairs. It is similar to the dictionaries in the other programming languages. The key advantage of using map in Golang is flexibility and efficiency. With maps, can easily retrieve a values by using corresponding keys, making it ideal for the scenarios where quick lookups are required. Maps also allow to add, modify, and delete key-value pairs dynamically.
70. Explain object-oriented architecture of Golang?
Ans:
Go’s object-oriented architecture refers to the way in which language supports the principles of the object-oriented programming. While Go does not have a traditional classes and inheritance like the some other languages, it does have a structures and methods that can be defined on structures. This provides the way to encapsulate data and behavior within single entity. By using the structs and methods, can achieve similar benefits to the object-oriented programming like code reusability, modularity, and encapsulation. Go promotes the composition-based approach where objects are built by the combining smaller objects, rather than relying on inheritance hierarchies. This makes a code easier to manage and understand.
71. What are anonymous structs and anonymous struct fields?
Ans:
Anonymous structs in Go are structs without specific name defined. They are used when want to create a struct type that is only used in a one place code. Anonymous struct fields are the similar but are used to embed anonymous fields within the struct.
72. Explain defer statement in Golan?
Ans:
In Golang, defer statement is used to postpone execution of a function untila surrounding function completes. This is useful when want to ensure that certain clean-up actions or resource releases are the performed before exiting a function.
73. What are advantages of passing pointers to functions?
Ans:
Efficiency : can avoid making copies of a data and work directly with original data instead. This can save memory and improve the performance, especially when dealing with the large data structures.
Shared memory : Pointers allow the multiple functions to access and modify a same data, facilitating data sharing and communication between the functions.
Flexibility : Pointers provide the flexibility in modifying data within function since changes made through the pointer are reflected in original data.
Dynamic memory allocation : Pointers can be used to allocate the memory dynamically, allowing the efficient management of a memory resources.
74. What are Golang methods?
Ans:
Golang methods are the functions associated with the specific type that allow to define behaviors and actions for that type. Methods can be defined for both the user-defined types and built-in types.
There are two types of the methods in Golang:
Value receiver methods : These operate on copy of value and do not modify original value.
Pointer receiver methods : These can modify original value and are generally used when there is need to modify underlying data.
75. How can ensure Go channel never blocks while sending data to it?
Ans:
Can use a buffered channel to prevent the Go channel from a blocking during a send operation. can specify the buffer size when creating a channel using the make function. For example:
ch := make(chan int, 10) // Create the buffered channel with a buffer size of 10 This allows channel to hold up to 10 values before blocking. When channel is full, further send operations will block until there is a space in the buffer. Using a buffered channel can help to prevent goroutines from blocking, which improves the concurrency in Go programs.
76. Explain why concurrency is not parallelism?
Ans:
Concurrency and parallelism are often used interchangeably, but t have distinct differences. Concurrency refers to the multiple tasks being executed simultaneously, while parallelism involves the splitting a task into the smaller sub-tasks and running them simultaneously. The difference lies in a nature of the tasks being executed. In concurrency, multiple tasks may be executing at a same time but they may not necessarily be related to same task. In parallelism, focus is on breaking down single task into the smaller sub-tasks.
77. What is data race?
Ans:
A data race occurs in the multi-threaded programs when two or more threads access the shared data concurrently without proper synchronization. This can lead to be unpredictable and erroneous behavior as order of execution and access to shared data becomes non-deterministic. Data races can result in the incorrect calculations or data corruption, compromising integrity and correctness of a program. To mitigate data races, can use techniques like a locks, mutexes, and atomic operations tobe enforce exclusive access to shared data.
78. How can detect data race in Go code?
Ans:
One way to detect the data race in Go code is to use built-in data race detector. It can be enabled with -race flag when building or running program. The detector will use the combination of lock-set and happens-before-based algorithms to report any data race that occurs during program’s execution. It’s important to note that a race-enabled binaries use 10 times CPU and memory, so it’s impractical to enable race detector all the time.
79. What are packages in Golang?
Ans:
Go programs are the usually organized into packages. A package is nothing but the collection of source files in the same directory. These files are compiled together. The main thing is that variables, functions, and constants are stored in a one source file. The files are visible to the other files in a same package.
80. What are two crucial operators used in Golang?
Ans:
‘*’ and ‘&’ operators are used in the Golang. ‘*’ is the dereferencing operator that use to declare a pointer. Also, use this operator to access the value stored at the address. When it comes to ‘&’ operator, it is known as a address operator, and use this operator to get address of a variable.
81. Name the Golang operators.
Ans:
- Arithmetic operators
- Bitwise operators
- Relational operators
- Logical operators
- Assignment operators
82. What is Golang variable scope?
Ans:
Golang variable scope is a nothing but a part of a code. This is where can access and modify specified variables. There are the two categories of scope variables: local variables and global variables. Local variables are usually declared inside the function or block of code, whereas global variables are declared outside of a function or block of code.
83. Mention three directories of Golang workspace?
Ans:
Golang workspace is usually the directory hierarchy of Go programs. A workspace consists of a three subdirectories, as mentioned below.
- src – This directory includes a source files as packages.
- pkg – Go package objects are the stored in this directory
- Bin – This directory includes executable programs.
84. List out advantages of Golang?
Ans:
- Quick and simple coding
- Golang comes with the garbage collector
- Go compilation is a quicker
- It provides the increased availability and reliability
- It can be employed for a large-scale projects
- It provides the powerful performance
- The learning curve is a low for Golang Golang supports the concurrency
85. Why is Golang so popular?
Ans:
- It is interpreted and statically typed language
- It supports the cross-platform application development
- It compiles the codes with speed
- Its syntax is a compact and simple
86. What are decision-making statements of Golang?
Ans:
Below are different decision-making statements of Golang.
- If statement – It is the simple decision-making statement If…else statement – If given condition is true, it will run the set of codes.
- Otherwise, it will run the another set of codes.
- Nested if statement – It is a statement where an if statement consists of the another if statement inside of it.
87. What is GoROOT variable in Golang?
Ans:
It is used to find a standard libraries. In other words, it is the variable used to locate Go SDK. It is always set to installation directory. use this variable to determine a root of the workspace.
88. what is GOPATH environment variable?
Ans:
- The GOPATH environment variable determines a location of the workspace.
- It is only environment variable that have to set when developing a Go code.
89. How arrays in GO works differently then C ?
Ans:
In GO Array works differently than it works in the C:
- Arrays are the values, assigning one array to the another copies all the elements
- If pass an array to a function, it will receive copy of array, not a pointer to it The size of an array is part of type. The types [10] int and [20] int are the distinct
90. Explain Type assertion is used for and how it does it?
Ans:
Type conversion is used to convert the dissimilar types in the GO. A type assertion takes an interface value and retrieve from it value of a specified explicit type.