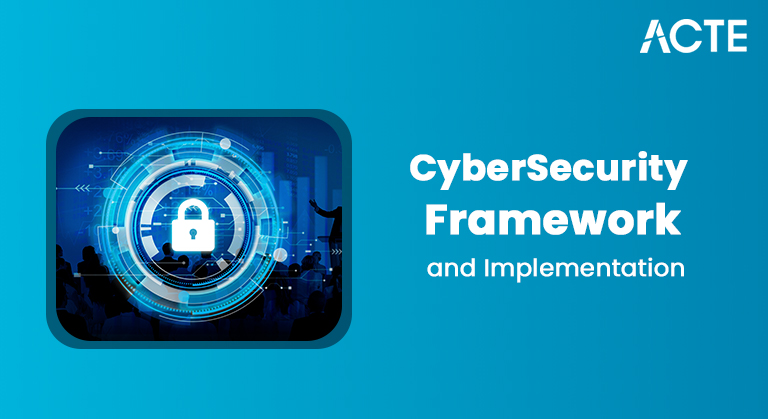
- Introduction to React Redux
- Core Principles of Redux
- Setting Up Redux with React
- Actions and Action Creators in Redux
- Reducers in Redux
- The Redux Store and State Management
- Connecting Redux with React Components
- Advanced Concepts in React Redux
- Conclusion
Introduction to React Redux
React Redux is a library that helps manage the state of React applications. While React provides a built-in state management system through state and reducer hooks, larger and more complex applications often require a more robust and centralized way to manage states across multiple components. React Redux enables this by storing the application’s state in a single object (the store) and providing a system of actions and reducers to modify and access this state. This concept is a key topic covered in our Web Designing & Development Courses which help learners build scalable, maintainable web applications.
Redux is based on three core principles:
- Single Source of Truth:The entire application state is stored in one central object called the store.
- State is Read-Only: The only way to change the State is by dispatching actions.
- Changes are Made with Pure Functions: Reducers (which specify how the state changes) are pure functions that take the current State and action as arguments and return the new State.
Core Principles of Redux
To understand React Redux, it’s essential to grasp its core principles, as they are fundamental to building maintainable and predictable applications. One of the key concepts is the Single Source of Truth. In Redux, the entire state of the application is stored in a single JavaScript object known as the store. This centralized approach simplifies state management and debugging since all state changes originate from one place, rather than being scattered across various components. A solid foundation in JavaScript and other relevant technologies like those covered in our guide on the Best Web Development Languages To Learn can help you better understand these concepts and apply them effectively. Another important principle is that the state is read-only. This means you cannot directly modify the Redux state. Instead, any changes must be made by dispatching actions structured payloads that describe what changes should occur. This controlled method of state change ensures consistency and predictability, preventing unexpected updates from components. Lastly, Redux emphasizes that changes are made with pure functions, known as reducers. These reducers define how the state transitions in response to actions. Being pure functions, reducers rely solely on their inputs to determine outputs and avoid any side effects. Rather than modifying the existing state, they return a new state object, maintaining the principle of immutability.
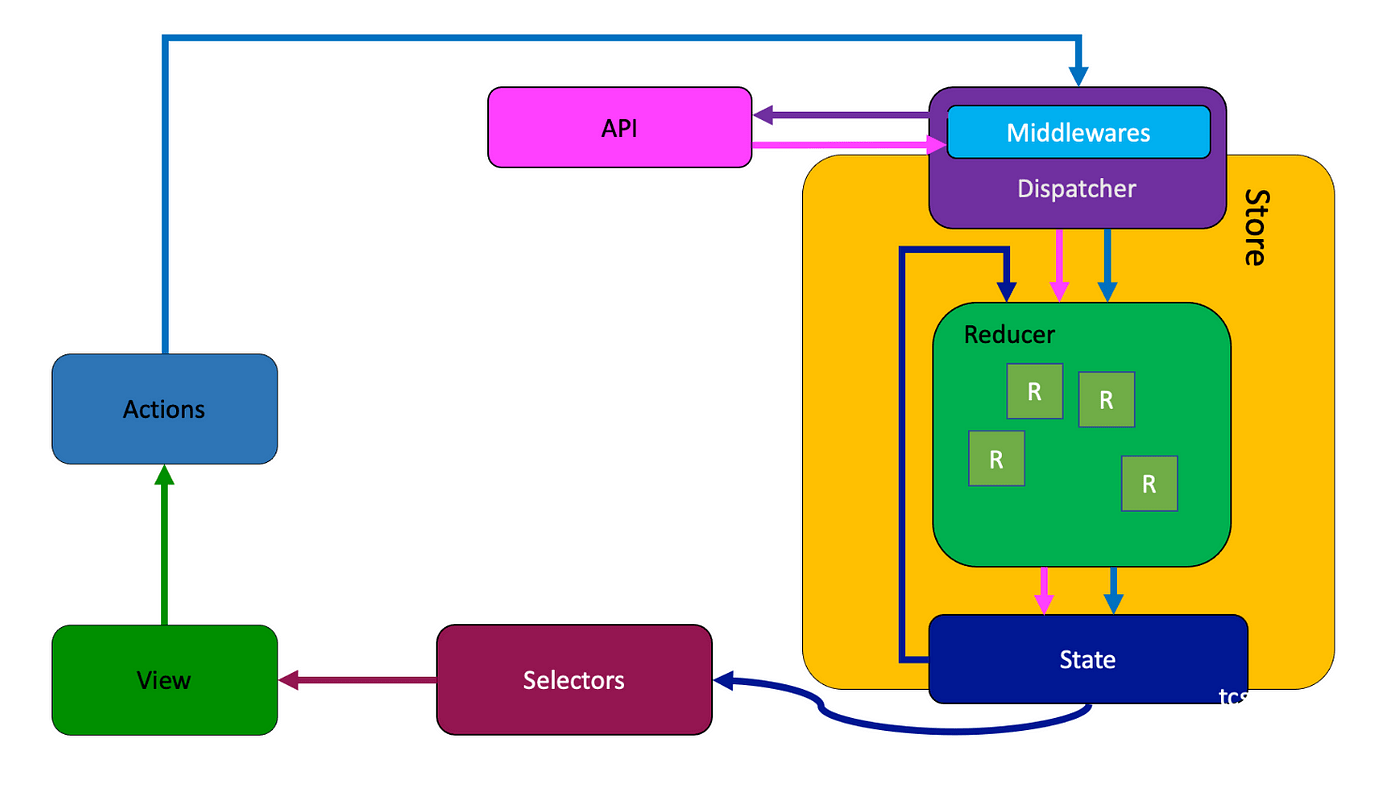
Setting Up Redux with React
To use Redux with React, you must install the react-redux library, which connects Redux to your React components. Here’s how you set up Redux in a React application:
Install Redux and React-Redux: Use npm or yarn to install the required packages:
npm install redux react-redux
- Create the Redux Store: The store holds the application’s State and allows actions to be dispatched. It’s created using the createStore function from Redux.
- import { create store } from ‘redux’;
- import rootReducer from ‘./reducers’;
- const store = createStore(rootReducer);
- Creating the Store: The store uses the createStore() function, passing the root reducer as an argument. Middleware can also be included for enhanced functionality, such as logging or asynchronous actions.
- Dispatching Actions: The state in a Redux store can only be updated by dispatching actions. Actions are sent to the store using the dispatch() method, such as store.dispatch(action), which triggers the store to update the state based on the action and the reducer logic.
- Unsubscribing from State Updates: If you no longer need to listen to state changes, you can unsubscribe by calling the function returned from store.subscribe(). This prevents unnecessary re-renders and optimizes performance when the state listener is no longer needed.
- State Immutability: Redux enforces the principle of immutability, meaning that instead of directly modifying the state, a new state object is returned by the reducer whenever an action is dispatched. This ensures that the state remains consistent and predictable throughout the application.
- const fetch data = () => {
- return async dispatch => {
- const response = await fetch(‘https://api.example.com/data’);
- const data = await response.json();
- dispatch({ type: ‘SET_DATA’, payload: data });
- };
- };
To provide the Redux store to your React application, you use the Provider component from the react-redux library. This component wraps the root App component and takes the Redux store as a prop, allowing all components within the app to access the store. In the code example, React, ReactDOM, Provider, and the main App component are imported. Then, within ReactDOM.render(), the App is wrapped inside the Provider, with the store passed to it. Finally, this structure is rendered into the DOM element with the ID ‘root’, enabling Redux to manage state across the entire React app.
Actions and Action Creators in Redux
In Redux, actions are plain JavaScript objects that describe the intention to change the state. Every action must have a type property, which specifies the action being performed. For example, a simple action might be const action = { type: ‘INCREMENT’ };, where the type indicates the increment operation. To simplify action creation, Redux uses action creators functions that return action objects. For instance, an action creator for the increment action could be written as const incrementation = () => ({ type: ‘INCREMENT’ });. This approach centralizes action logic and ensures consistency. If you’re setting up a React project on Windows to start working with Redux, check out this guide on how to Install React on Windows. To modify the state, actions must be dispatched using the store’s dispatch() method. For example, store.dispatch(incrementation()); would send the action to the store, triggering the state change. Dispatching actions is the primary way to update the state in Redux, allowing developers to manage application state in a predictable and organized manner.
Excited to Obtaining Your web developer Certificate? View The web developer course Offered By ACTE Right Now!
Reducers in Redux
Reducers are functions in Redux that determine how the application’s state changes in response to actions. They take the current state and an action as arguments and return a new state based on the action type. A basic reducer function receives two parameters: the current state and the dispatched action. If the action type matches one of the defined cases, the reducer returns a new state; otherwise, it returns the existing state unchanged. For example, a simple counter reducer might use an initialState with a count of 0 and update this count based on ‘INCREMENT’ or ‘DECREMENT’ action types. These foundational concepts are a core part of our Web Developer Certification which prepares learners to build scalable applications using state management best practices. In larger applications, it’s common to have multiple reducers handling different parts of the state. Redux provides the combineReducers function to merge these individual reducers into a single root reducer, organizing them under separate keys—for instance, combining a counterReducer under the counter key in the root reducer.
Interested in Pursuing Web Developer Master’s Program? Enroll For Web developer course Today!
The Redux Store and State Management
The Redux store stores the application’s State and provides methods for accessing and updating it.
To create a Redux store, the createStore function is used, typically passing the root reducer as an argument, like so: const store = createStore(rootReducer);. Once the store is created, you can access the current state of the application by using the getState() method, which returns the current state object. For example, const currentState = store.getState(); will retrieve the current state. Additionally, you can subscribe to the store to be notified whenever the state changes. This is useful for responding to state updates in real-time. By calling the subscribe() method on the store and providing a callback function, you ensure that the callback is executed every time an action is dispatched and the state is updated. For instance, store.subscribe(() => console.log(store.getState())); will log the current state to the console each time the state changes. If you’re trying to decide between building a web or mobile application, our detailed comparison of Web Development vs. App Development can help you understand the best approach for your project.
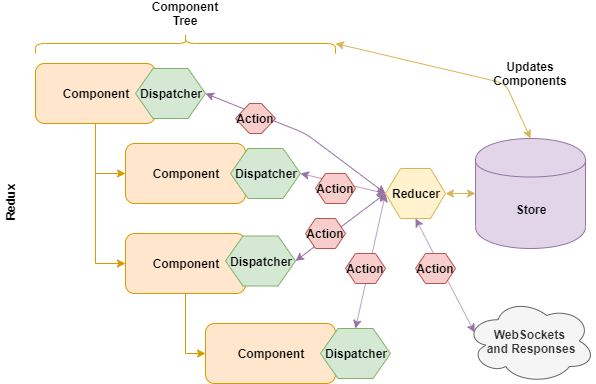
Connecting Redux with React Components
React Redux provides the connect() function to link Redux state and dispatch actions to React components, allowing components to access the state and dispatch actions without needing to manually pass them as props. To achieve this, two main functions are used: mapStateToProps and mapDispatchToProps. The mapStateToProps function receives the Redux state and returns an object whose properties will be passed as props to the component, enabling the component to access specific pieces of state. For example, mapStateToProps might return { count: state.counter.count } to expose the count value to the component. On the other hand, mapDispatchToProps maps dispatchable actions to props by wrapping action creators in a dispatch call, allowing the component to trigger state changes for instance, using increment: () => dispatch(incrementation()). Once both functions are defined, the connect() function from react-redux is used to wrap the component, binding the mapped state and actions to it. An example is a Counter component that displays the count and includes buttons to increment or decrement it, which is then exported using connect(mapStateToProps, mapDispatchToProps)(Counter), enabling full Redux integration. If you’re looking to understand how the map function can simplify array transformations in React components, check out our guide on Map Function in React JS
Advanced Concepts in React Redux
After mastering the basic concepts, you can explore React Redux’s advanced features, including Middleware, asynchronous actions, and optimizations.
a. MiddlewareMiddleware in Redux allows you to intercept actions before they reach the reducer. Standard Middleware includes redux-thunk for handling asynchronous actions and redux-logger for logging actions.
b. Async ActionsBy default, Redux actions must be plain objects. To handle asynchronous actions, you can use Middleware like redux-thunk, which allows action creators to return functions instead of plain objects.
To optimize performance, Redux uses shallow equality checks. React-Redux also provides hooks like useSelector and useDispatch, which optimize how components subscribe to the store, reducing unnecessary re-renders.
Conclusion
React Redux provides a structured way to manage state in large React applications by centralizing state management and enforcing predictable patterns through actions, reducers, and the Redux store. This approach ensures that the state remains consistent, easy to trace, and maintainable as applications grow in complexity. Concepts like these are covered in our Web Designing Training which equips learners with the skills to build robust and responsive web applications. Actions are dispatched to describe changes, reducers handle those changes by returning a new state, and the store acts as the single source of truth for the application. By using the Provider component to give React components access to the store and connect() or hooks like useSelector and useDispatch to bind state and actions to components, React Redux simplifies the process of syncing UI with state changes. It also supports middleware for handling asynchronous logic and debugging tools like Redux DevTools. Overall, React Redux is essential for developers building scalable, maintainable applications with a clean separation between logic and presentation.