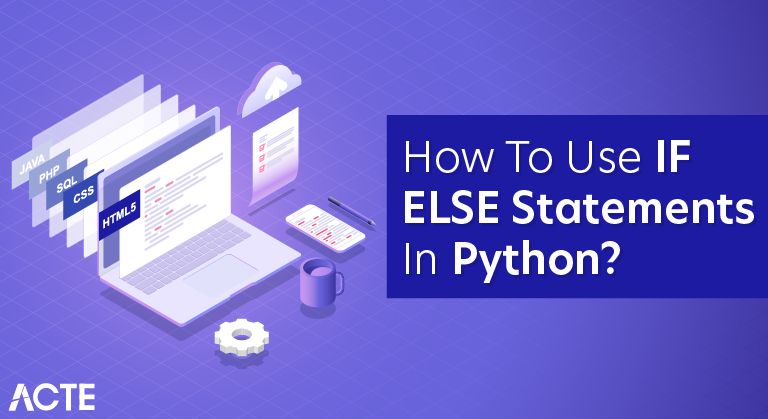
Must-Know How to Use IF ELSE Statements in Python & How To Master It
Last updated on 15th Jul 2020, Blog, General
The Python if else statement is an extended version of the if statement. While the Python if statement adds the decision-making logic to the programming language, the if else statement adds one more layer on top of it.
Both of them are conditional statements that check whether a certain case is true or false. If the result is true, they will run the specified command. However, they perform different actions when the set condition is false.
When an if statement inspects a case that meets the criteria, the statement will execute the set command. Otherwise, it will do nothing. Look at this basic example of Python if statement:
Example Copy
x = 10 y = 15
if x < y:
print(‘X is greater than Y.’)
Meanwhile, the if else statement will execute another specified action if it fails to meet the first condition.
Simply put, the if else statement decides this: if something is true, do something; if something is not true, then do something else.
Python Conditions/Decision-Making Statements
Table of Content
- if Statement
- Flowchart
- Example
- if Else in One Line
- if Else Statement
- Flowchart
- Example
- if-Elif-Else Statement
- Flowchart
- Example
- Nested If-Else Statement
- Flowchart
- Example
- Using Not Operator with If Else
- Examples
- Using And Operator with If Else
- Flowchart
- Example
- Using In Operator with If Else
- Examples
Syntax
Syntax
Syntax
Syntax
Go back to Python Tutorial
Python if Statement
A bare Python if statement evaluates whether an expression is True or False. It executes the underlying code only if the result is True.
Given below is the syntax of Python if statement.
Syntax
if Logical_Expression :
Indented Code Block
Flowchart
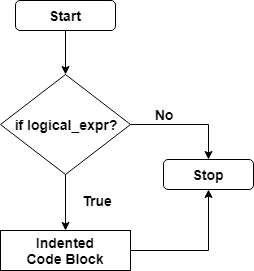
Basic Python if Statement Flowchart
Example
days = int(input(“How many days in a leap year? “)) if days == 366: print(“You have cleared the test.”) print(“Congrats!”)
The output of the above code is –
How many days in a leap year? 366
You have cleared the test.
Congrats!
Python if Else Statement
A Python if else statement takes action irrespective of what the value of the expression is.
If the result is True, then the code block following the expression would run. Otherwise, the code indented under the else clause would execute.
Given below is the syntax of Python if Else statement.
Syntax
if Logical_Expression : Indented Code Block 1 else : Indented Code Block 2
Flowchart
If-Else Statement Flowchart
Example
answer = input(“Is Python an interpreted language? Yes or No >> “).lower()
if answer == “yes” :
print(“You have cleared the test.”)
else :
print(“You have failed the test.”)
print(“Thanks!”)
When you run the above code, it asks for your input. It converts the entered value it into lower case and runs the if-else condition.
If you enter a ‘yes,’ then the output of the above code would be –
Is Python an interpreted language? Yes or No >> yes
You have cleared the test.
Thanks!
If you enter a ‘no,’ then the result of the above code would be –
Is Python an interpreted language? Yes or No >> no
You have failed the test.
Thanks!
Python If Else in One Line
Python provides a way to shorten an if/else statement to one line. Let’s see how can you do this.
The one-liner If-else has the following syntax:
- # If Else in one line – Syntax
- value_on_true if condition else value_on_false
- See the below example of If-Else in one line.
- >>> num = 2
- >>> ‘Even’ if num%2 == 0 else ‘Odd’
- ‘Even’
- >>> num = 3
- >>> ‘Even’ if num%2 == 0 else ‘Odd’
- ‘Odd’
- >>> num = 33
- >>> ‘Even’ if num%2 == 0 else ‘Odd’
- ‘Odd’
- >>> num = 34
- >>> ‘Even’ if num%2 == 0 else ‘Odd’
- ‘Even’
- >>>
Python: It’s All About the Indentation
Python follows a convention known as the off-side rule, a term coined by British computer scientist Peter J. Landin. (The term is taken from the offside law in association football.) Languages that adhere to the off-side rule define blocks by indentation. Python is one of a relatively small set of off-side rule languages.
nbsp; Recall from the previous tutorial on Python program structure that indentation has special significance in a Python program. Now you know why: indentation is used to define compound statements or blocks. In a Python program, contiguous statements that are indented to the same level are considered to be part of the same block.
Thus, a compound if statement in Python looks like this:
- if <expr>:
- <statement>
- <statement>
- …
- <statement>
- <following_statement>
Here, all the statements at the matching indentation level (lines 2 to 5) are considered part of the same block. The entire block is executed if <expr> is true, or skipped over if <expr> is false. Either way, execution proceeds with <following_statement> (line 6) afterward.
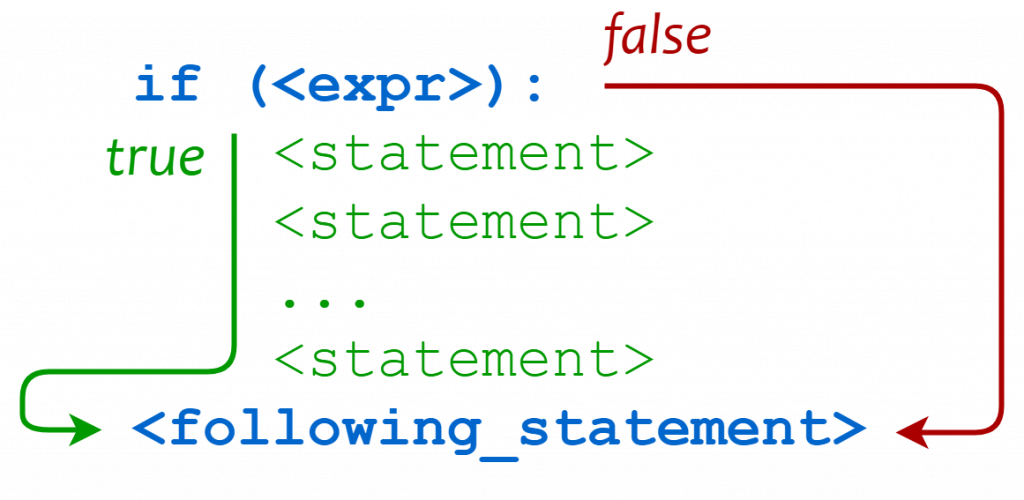
Python Compound if Statement
nbsp; nbsp; nbsp; Notice that there is no token that denotes the end of the block. Rather, the end of the block is indicated by a line that is indented less than the lines of the block itself.
Note: In the Python documentation, a group of statements defined by indentation is often referred to as a suite. This tutorial series uses the terms block and suite interchangeably.
Consider this script file foo.py:
- if ‘foo’ in [‘bar’, ‘baz’, ‘qux’]:
- print(‘Expression was true’)
- print(‘Executing statement in suite’)
- print(‘…’)
- print(‘Done.’)
- print(‘After conditional’)
Running foo.py produces this output:
C:\Users\john\Documents>python foo.py
After conditional
nbsp; nbsp; nbsp; The four print() statements on lines 2 to 5 are indented to the same level as one another. They constitute the block that would be executed if the condition were true. But it is false, so all the statements in the block are skipped. After the end of the compound if statement has been reached (whether the statements in the block on lines 2 to 5 are executed or not), execution proceeds to the first statement having a lesser indentation level: the print() statement on line 6.
Blocks can be nested to arbitrary depth. Each indent defines a new block, and each outdent ends the preceding block. The resulting structure is straightforward, consistent, and intuitive.
Here is a more complicated script file called blocks.py:
- # Does line execute? Yes No
- # — —
- if ‘foo’ in [‘foo’, ‘bar’, ‘baz’]: # x
- print(‘Outer condition is true’) # x
- if 10 > 20: # x
- print(‘Inner condition 1’) # x
- print(‘Between inner conditions’) # x
- if 10 < 20: # x
- print(‘Inner condition 2’) # x
- print(‘End of outer condition’) # x
- print(‘After outer condition’) # x
The output generated when this script is run is shown below:
C:\Users\john\Documents>python blocks.py
Outer condition is true
Between inner conditions
Inner condition 2
End of outer condition
After outer condition
Note: In case you have been wondering, the off-side rule is the reason for the necessity of the extra newline when entering multiline statements in a REPL session. The interpreter otherwise has no way to know that the last statement of the block has been entered.