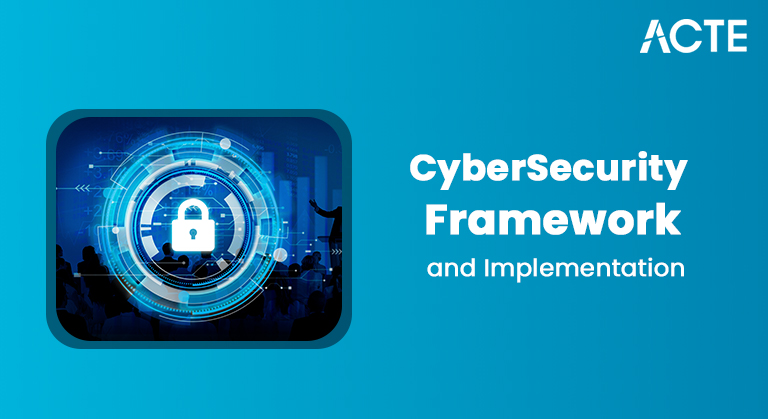
- What is a List in Python?
- The len() Function: The Key to Determining List Length
- How the len() Function Works
- Determining Length for Nested Lists
- Performance Considerations When Determining Length
- Common Mistakes to Avoid
- sing len() with Other Data Types
- Conclusion
Python is a user-friendly, powerful, and versatile programming language that has gained the interest of developers worldwide. The list is one of the most used data structures in Python. A list enables you to keep more than one item in a single variable, and it is an inevitable tool for programming language. Whether you’re maintaining numbers, strings, or other objects in store, knowing how to work with and manipulate lists is an elementary skill that any Python developer needs to know. One of the most essential parts of working with lists is getting their length or the number of elements they hold. Knowing the length of a list is vital when Data Science Course Training comes to things like iterating over its constituents, checking data, or regulating program flow dependent on the size of the list. Luckily, Python projects have a built-in function, len(), that enables you to get the length of a list instantly. For instance, invoking len(my_list) will give the length of my_list. In this blog, we will discuss different methods to find the length of a list, with emphasis on the len() function and how it is used. We will also cover some key considerations and common pitfalls to watch out for when using lists, including dealing with empty lists or non-standard data types. By mastering these methods, you can work with lists efficiently in your Python projects.
What is a List in Python?
Before diving into how to find the length of a list, let’s first clarify what a list is in Python. A list is a built-in data structure that can hold a collection of items, which can be of various types. Parsing in NLP can contain integers, strings, other lists, and custom objects. Lists in Python are created using square brackets ([]), and commas separate the items inside the list. Here’s a basic example of a Python list: my_list = [1, 2, 3, 4, 5] In this example, my_list contains five elements, all integers.
Properties of Python Lists:
- Ordered: The order of elements in a list is maintained.
- Mutable: You can change, add, or remove elements.
- Heterogeneous: A list can contain items of different types.
Gain in-depth knowledge of Data Science by joining this Data Science Online Course now.
The Len() Function: The Key to Determining List Length
The `len()` function in Python is a built-in function used to determine the length of a list. It’s a simple and highly efficient function that returns the number of elements in the list. The syntax is straightforward: `len(my_list)`. For instance, if you have a list like `my_list = [1, 2, 3, 4, 5]` and you use the command `print(len(my_list))`, the output will be `5`, since the list contains Macros in Excel . This function is widely used in Python programming for a variety of tasks, such as checking the size of a list, iterating over lists, and performing conditional checks based on list length.
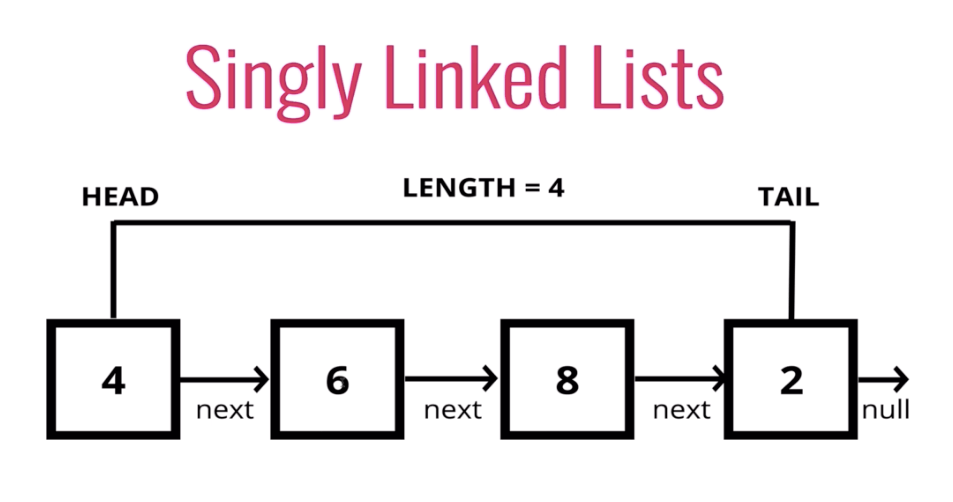
How the len() Function Works
The len() function works by internally maintaining a reference to the list size. When you call len() on a list, Python projects return the number of elements it contains. It’s important to note that the list itself must already exist for the function to work, as len() operates on a list object created in memory.
- my_list = [“apple”, “banana”, “cherry”]
- print(len(my_list))
Output: 3
The list contains three items in the above example, so len(my_list) returns 3.
Key Points about the len() Function:
- It is a built-in Python function that works with any iterable, including lists, strings, and tuples.
- It runs in constant time (O(1)) for lists, meaning the time taken to compute the length is the same regardless of the list size.
- Calling len() on Non-Iterable Objects: The len() function can only be used with iterable objects like lists, strings, or tuples. If you try to call it on a non-iterable object, such as an integer, it will raise a TypeError.
- number = 10
- print(len(number))
- Misunderstanding Nested List Length: As discussed earlier, len() only counts top-level elements. Be mindful when working with nested lists.
- Modifying the List During Iteration: Iterating through a list and changing it at the same time (adding or removing elements) can lead to unexpected behavior. Always be cautious when modifying a list while iterating over it.
Start your journey in Data Science by enrolling in this Data Science Online Course .
Determining Length for Nested Lists
Once one has data, the second step is selecting the right AI model. Different problems must be solved differently, and the right model selection is crucial to the project’s success.
Determining the length of a nested list in Python can be a bit more complex, as the `len()` function only counts the top-level elements and does not consider the elements within the sub-lists. For example, consider a nested list like `nested_list = [[1, 2, 3], [4, 5], [6, 7, 8, 9]]`. Using `len(nested_list)` will return `3`, as there are three top-level elements (each being a sub-list). However, Website Analytics you’re interested in finding the total number of elements across all the sub-lists, you would need to iterate through the list and sum the lengths of each sub-list manually. This can be done with the following code: `total_length = sum(len(sublist) for sublist in nested_list)`, which would return `9`, indicating the total number of elements in all sub-lists.
Performance Considerations When Determining Length
The len() function is highly efficient for determining the length of a list because it operates with constant time complexity, O(1). This means it returns the length almost instantly, regardless of the list size. This efficiency is due to how Python developers internally store the length of objects like lists, tuples, and strings. For these data structures, the length is tracked, so len() can return that value without iterating through the entire list. However, you may need to consider performance and memory usage if you’re working with large lists or more complex data structures, such as Data Science Course Training. For example, when building a list by dynamically adding elements, Python developers might resize the list to accommodate more items, which can have a slight performance impact. In cases where you work with massive datasets, it’s often beneficial to keep track of the length manually as you modify the list to avoid unnecessary resizing operations. Considering these factors, you can make more informed decisions on when and how to use len() efficiently in your Python projects.
Take charge of your Data Science career by enrolling in ACTE’s Data Science Master Program Training Course today!
Common Mistakes to Avoid
While determining the length of a list in Python is straightforward, beginners often make a Python for Data Science common mistakes. Here are some of the most frequent pitfalls:
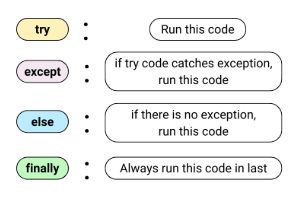
Raises TypeError
Preparing for Data Science interviews? Visit our blog for the best Data Science Interview Questions and Answers!
sing len() with Other Data Types
While the len() function is commonly used with lists in Python, it is also versatile and works with several other data types that are considered iterables or collections. Lightgbm Algorithm include strings, tuples, dictionaries, sets, and even custom objects that implement the __len__() method. For instance, len(“Hello”) would return 5, and len((1, 2, 3)) would return 3. When working with dictionaries, len() returns the number of key-value pairs. This makes len() a powerful and consistent tool for checking the size of various data structures in Python, enhancing code readability and efficiency across a wide range of use cases.
Conclusion
Python is a versatile, powerful, and user-friendly programming language that has become a favorite among Python developers worldwide. One of the most commonly used data structures in Python is the list. Lists allow you to store multiple items in a single variable, making them an essential tool in programming language. Whether you’re storing numbers, strings, or other objects, understanding how to manipulate and interact with lists is a fundamental skill for any Python programmer. A key aspect of working with lists is determining their length or the number of elements they contain. Knowing the length of a list is Data Science Course Training for tasks such as iterating through its components, validating data, or controlling program flow based on the list’s size. Fortunately, Python provides a built-in function, len(), that allows you to determine a list’s length quickly. For example, calling len(my_list) will return the number of elements in my_list. In this blog, we will explore the various ways to determine the length of a list, focusing on the len() function and its usage. We’ll also discuss crucial considerations and common pitfalls to avoid when working with lists, such as handling empty lists or lists containing non-standard data types. Mastering these techniques will ensure you can handle lists effectively in your Python projects.