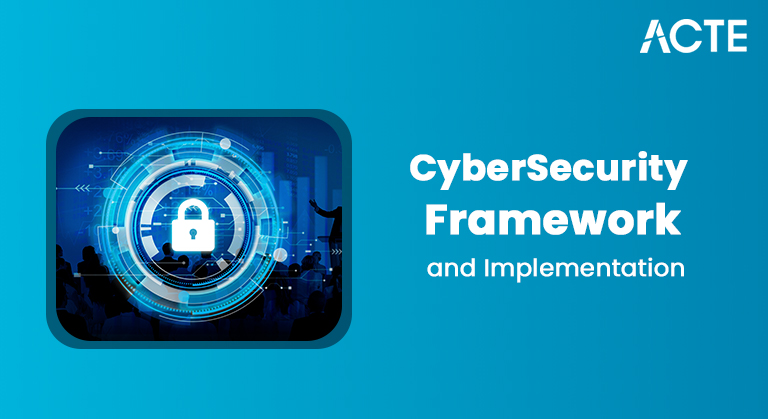
- Introduction
- Setting Up the Project
- Understanding the JavaScript Basics
- Creating the Calculator Interface
- Adding the JavaScript Functionality
- Testing and Debugging the Calculator
- Conclusion
Building a simple calculator using JavaScript is a great project for beginners to grasp the core concepts of JavaScript, HTML, and CSS. In this tutorial, we will guide you step-by-step in creating a basic calculator that can perform four fundamental operations: addition, subtraction, multiplication, and division. The first step involves structuring the layout of the calculator using HTML, creating buttons for each number and operation. Next, we will style the calculator using CSS, making sure it looks clean and user-friendly, similar to interfaces often seen in Data Science Training tools and applications. Finally, we will add JavaScript to bring the calculator to life, enabling it to perform calculations when the user clicks on the buttons. By the end of this tutorial, you’ll have built a fully functional calculator that can be embedded in any webpage. This hands-on project will not only boost your web development skills but also give you a deeper understanding of how HTML, CSS, and JavaScript work together to create interactive web applications.
Interested in Obtaining Your Data Science Certificate? View The Data Science Course Training Offered By ACTE Right Now!
Introduction
JavaScript is a powerful and versatile programming language commonly used for adding interactivity to web pages. In this tutorial, we will walk you through creating a simple calculator capable of performing four basic operations: addition, subtraction, multiplication, and division. The process will begin with structuring the calculator’s user interface using HTML, providing the foundation for the app, similar to how Python Keywords provide the foundational building blocks in Python programming. We will then enhance its appearance by styling it with CSS, ensuring that the calculator is both functional and visually appealing. Finally, we will integrate JavaScript to enable the calculator’s operations, such as responding to button clicks and displaying results on the screen.
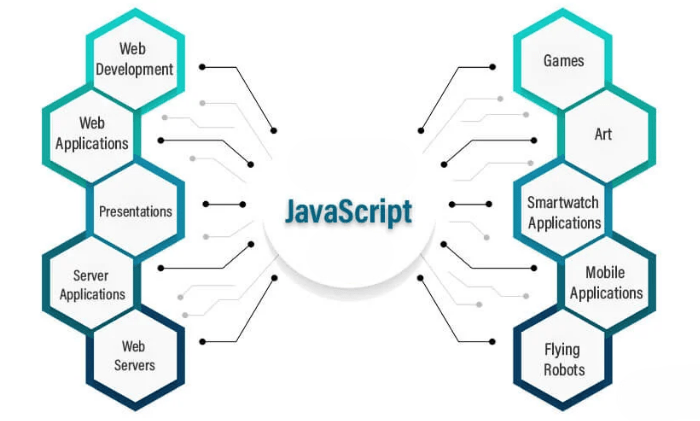
Setting Up the Project
HTML Structure
The HTML will create the calculator’s structure, including buttons for each number, the operations, and a display area to show the results.The HTML structure of a calculator provides the foundation for its layout and user interface. It consists of a container that holds all the elements, including the display screen and the buttons for numbers and operations. At the top, there is usually an input or a div element that acts as the display, showing the numbers entered and the results of calculations similar to how data is presented in dashboards when exploring What is Data Science. Below the display, buttons are arranged in a grid layout representing digits (0–9), basic arithmetic operators (+, −, ×, ÷), an equals (=) button to perform the calculation, and a clear (C) button to reset the display. Each button is typically created using the “button” tag and assigned specific classes or IDs for styling and JavaScript functionality. This structure ensures that the calculator is easy to interact with and visually intuitive. Organizing elements properly in HTML also helps in applying CSS styles effectively and allows JavaScript to easily access and control elements for dynamic interactions. A clean and well-structured HTML layout is key to building a responsive and functional calculator.
To Earn Your Data Science Certification, Gain Insights From Leading Data Science Experts And Advance Your Career With ACTE’s Data Science Course Training Today!
CSS Styling
Let’s make our calculatorLet’slet’se neat and presentable. We will define the size of the buttons, align them properly, and give the display a clean look.Here isHere’Here’sCSS code for basic styling:
- {
- margin: 0;
- padding: 0;
- box-sizing: border-box;
- }
- body {
- font-family: Arial, sans-serif;
- display: flex;
- justify-content: center;
- align-items: center;
- height: 100vh;
- background-color: #f5f5f5;
- }
- calculator {
- background-color: white;
- border-radius: 10px;
- box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
- padding: 20px;
- width: 260px;
- }
- display {
- width: 100%;
- height: 50px;
- text-align: right;
- font-size: 24px;
- padding: 10px;
- margin-bottom: 20px;
- border: 2px solid #ccc;
- border-radius: 5px;
- background-color: #f9f9f9;
- }
- buttons {
- display: grid;
- grid-template-columns: repeat(4, 1fr);
- gap: 10px;
- }
- button {
- font-size: 18px;
- padding: 20px;
- border: 2px solid #ccc;
- border-radius: 5px;
- background-color: #f1f1f1;
- cursor: pointer;
- transition: background-color 0.3s;
- }
- button: hover {
- background-color: #ddd;
- }
- .operator {
- background-color: #f7a700;
- color: white;
- }
- operator: hover {
- background-color: #e68a00;
- }
Understanding the JavaScript Basics
Before diving into the implementation of our calculator, let’s fly review a few key JavaScript concepts that we will be using:
Functions
In JavaScript, a function is a block of code designed to perform a specific task. Functions help organize code and make it reusable. In our calculator project, we’ll define functions to handle various actions such as appending numbers to the display, setting the chosen operation (like addition or subtraction), and calculating the final result when the equals button is pressed. By using functions, we can keep our code clean, efficient, and easy to manage as we build more features—just like the structured approach taught in Data Science Training programs.
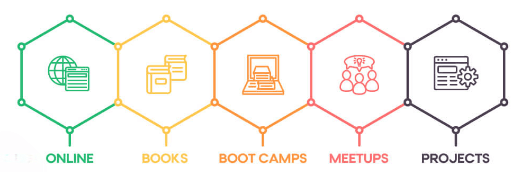
Event Handling
Event handling in JavaScript enables us to respond to user interactions, such as mouse clicks, key presses, or form submissions. For our calculator, we will use the onclick event to detect when a user presses a button. Each button will have an associated function that gets triggered on click, performing specific actions like adding a number to the display or calculating a result. This approach makes the calculator interactive and allows us to link user input directly to JavaScript functions.
Creating the Calculator Interface
Now that we’ve completed the HTML structure and styled it with CSS, let’s add JavaScript functionality to bring the project to life much like how Big Data vs Data Science compares the foundation and functionality of data-driven technologies.
- The main functionalities of the calculator will include:
- Appending numbers to the display when a number is clicked.
- Setting operations like addition, subtraction, multiplication, and division.
- Calculating the result when the equals button is clicked.
- Clearing the display when clicking the” “” “b” “”” on.
- let current input = ”;
- Store the current input string
- let previous input = “;
- Sto” the previous input (used for operations)
- let current operation = ”;
- Store the current operation
- Append number to the display
- function appendNumber(number) {
- current input += number;
- Add number to the current input
- document.getElementById(‘display’).value = current input;
- Update display
- }
- Set the operation (addition, subtraction, etc.)
- function separation(operator) {
- if (cif (cif (cif (current input ===”) return;
- Prevent operation if there’s no number (previous input !==”) {put ===”) result();
- If there is existing input,
- catherthere’sexistinglculate before setting a new operation
- }
- current operation = operator;
- Store the current operation
- previous input = current input;
- Store the previous input for the operation
- current input = “; “Rese” cu “ent input for next number
- }
- Calculate the result based on the operation
- function calculateResult() {
- if (previous (previous input if (previous input === ” || current input === ”) return;
- Prevent calculation if there’s no input current input result;
- previous input === ” ||put === ”) rent input result;previous input current input result
- current operation) {
- case ‘+’:
- result = parseFloat(previous input) + parseFloat(current input);
- break;
- case ‘-‘:’ ‘result = parseFloat(previous input) – parseFloat(current input);
- break;
- case ‘*’:
- result = parseFloat(previous input) * parseFloat(current input);
- break;
- case case ‘result = parseFloat(previous input) / parseFloat(current input);
- break;
- default:
- return;
- }
- document.getElementById(‘display’).value = result;
- Display the result
- previousInput = result.toString();
- Set the result as the previous input for further calculations
- current input = “;
- Res” current input
- }
- Clear the display and reset the calculator
- function clearDisplay() {
- current input = ”;
- previous input = ”;
- current operation = ”;
- document.getElementById(‘display’).value = ”;
- }
Are You Considering Pursuing a Data Science Master’s Degree? Enroll For Data Science Masters Course Today!
Adding the JavaScript Functionality
Handling Button Clicks
We will define functions to handle the button clicks. Let’s do this by appendLet’s Letting numbers to the display.
Implementing Basic Operations
Next, we will implement the functionality for setting the operations (addition, subtraction, multiplication, and division).
Calculating the Result
Now, we’ll implement the calculateResult function, which performs the actual calculations based on the selected operation demonstrating logical thinking and coding skills essential for exploring Python Career Opportunities.
Clearing the Display
Finally, we will add a function to clear the display when the “C,” “u, “ton,” i,” and “r “are used.
Testing and Debugging the Calculator
After implementing all the necessary functions for your calculator, it’s time to test how well it works. Start by opening the HTML file in your preferred web browser. Begin by pressing the number buttons to ensure they correctly appear on the calculator’s display. Next, select one of the basic operations addition, subtraction, multiplication, or division and make sure it registers the input. Press the equals (=) button to calculate the result and confirm that the correct value is displayed. You should also test the clear (C) button to verify that it resets the calculator’s display properly. Try out different combinations of inputs, including edge cases like dividing by zero or entering multiple operations without pressing equals practicing debugging and logic handling, which are among the Top Reasons To Learn Python. If something doesn’t work as expected, open the browser’s developer console (usually with F12 or right-click > Inspect > Console tab) and look for any error messages. These can help you identify and fix problems in your JavaScript code. Testing thoroughly ensures a smooth and reliable user experience.
Preparing for a Data Science Job Interview? Check Out Our Blog on Data Science Interview Questions & Answer
Conclusion
You’ve built a simple calculator using JavaScript, and in doing so, you’ve taken an important step in your web development journey. This project introduced you to essential JavaScript concepts such as event handling, functions, and performing basic arithmetic operations. Alongside the JavaScript logic, you also learned how to create a clean and functional user interface using HTML and enhance its appearance with CSS. These skills form the foundation of interactive web development. This tutorial not only helped you understand how to structure a project but also how to bring static content to life with interactivity an approach often emphasized in Data Science Training to create dynamic and engaging user experiences. It’s a great starting point for anyone new to web development. Now that you’ve completed the basic version, you can challenge yourself by adding more advanced features like decimal support, keyboard input handling, memory functions, or even scientific operations. By following this tutorial, you’ve gained valuable hands-on experience in combining HTML, CSS, and JavaScript to build a real, working web application that can be used and expanded further.