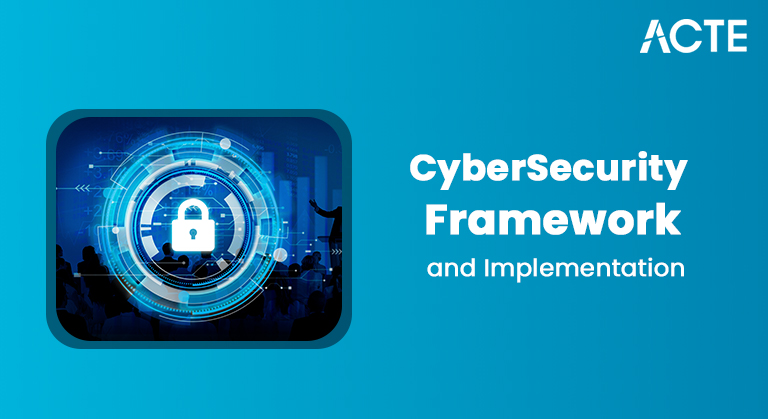
- Introduction
- Why Email Validation is Important
- Basic Email Structure
- Methods of Email Validation
- Steps to Implement Email Validation in JavaScript
- Understanding Regular Expressions for Email Validation
- Testing the Email Validation
- Conclusion
Introduction Email Validation
Email validation is an essential step in web development to ensure users enter a correctly formatted email address before submitting a form. This process helps maintain the accuracy and reliability of the data collected, especially when the email is required for communication, password recovery, or account verification. An invalid email address can lead to issues such as undelivered messages, failed registration, or even security risks. Therefore, validating user input, particularly email addresses, improves both functionality and user experience. In this guide, we will walk through the process of email validation using JavaScript a concept that also plays a role in form handling within Data Science Training projects. JavaScript allows developers to check the format of an email input in real-time, providing instant feedback to the user. We’ll explore different methods such as using regular expressions (regex) to ensure the email follows a standard pattern for example, containing an “@” symbol and a valid domain. We will also look at how to trigger validation on form submission or while typing. Implementing proper email validation helps keep your application secure, efficient, and user-friendly.
Are You Interested in Learning More About Data Science? Sign Up For Our Data Science Course Training Today!
Why Email Validation is Important
- Correct Information: Users provide accurate and valid data.
- Prevents Errors: It minimizes the chances of errors such as bounced emails.
- Improves User Experience: Users are immediately informed if they enter an incorrect email format, similar to how Python Generators efficiently handle iterations and yield results without needing to store large amounts of data in memory.
- Security: It helps prevent the use of fake or invalid email addresses, which is important for applications that require user verification or send important information.
- Server Load Reduction: Prevents unnecessary server-side validation if the input is verified on the client side.
- Data Quality: Ensures that your database contains high-quality, usable contact information for future communication or marketing purposes.
- Faster Form Submission: Immediate client-side validation speeds up the form submission process by catching mistakes early.
- Professionalism: Demonstrates attention to detail and builds trust with users by showing that the website actively checks and validates input.
- document.getElementById(“email”).setCustomValidity(“Invalid email address format.”);
- document.getElementById(“email form”).addEventListener(“submit”, function(event) {
- event.preventDefault();
- var email = document.getElementById(“email”).value;
- var errorMessage = document.getElementById(“error-message”);
- if (!isValidEmail(email)) {
- errorMessage.textContent = “Please enter a valid email address.”;
- } else {
- errorMessage.textContent = “”;
- alert(“Form submitted successfully!”);
- Proceed with form submission or further processing
- }
- });
- function isValidEmail(email) {
- var regex = /^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/;
- return regex.test(email);
- }
- ^: Anchors the match at the start of the string.
- [a-zA-Z0-9._-]+: Matches one or more characters in the local part (letters, numbers, periods, underscores, and hyphens).
- @: The “@” symbol must be present.
- [a-zA-Z0-9.-]+: Matches one or more characters in the domain part (letters, numbers, periods, and hyphens), similar to how What is Logistic Regression involves matching patterns and relationships between variables in a dataset to make predictions.
- \.: Escapes the period to ensure it matches a literal dot.
- [a-zA-Z]{2,}: Matches the top-level domain (TLD), which must be at least two characters long.
- $: Anchors the match at the end of the string.
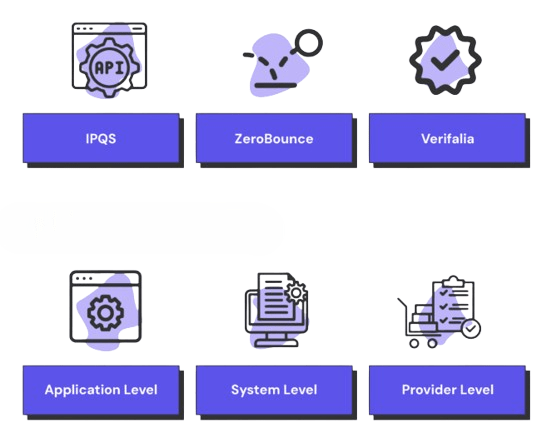
Basic Email Structure
A valid email address follows a specific format that allows it to be correctly interpreted by email systems. Understanding this basic structure is essential when validating user input. A standard email address consists of three main parts: the local part, the “@” symbol, and the domain part. The local part comes before the “@” symbol and typically includes the username or identifier of the email account. It can contain letters, numbers, and certain special characters such as periods (.), underscores (_), and hyphens (-), but it should not start or end with a special character—similar to understanding the Advantages & Disadvantages of Python, where syntax rules and flexibility come into play. Following the “@” symbol is the domain part, which represents the mail server that will handle the email. This part includes a domain name (like gmail, yahoo, or your company name) and a top-level domain (TLD) such as .com, .org, or .net. For example, in the email address “example.user@gmail.com”, “example.user” is the local part and “gmail.com” is the domain part.A properly structured email ensures reliable delivery and is essential for user verification, communication, and form validation in web applications.
To Explore Data Science in Depth, Check Out Our Comprehensive Data Science Course Training To Gain Insights From Our Experts!
Methods of Email Validation
Using Regular Expressions
Regular expressions (regex) are patterns used to match character combinations in strings. A regex can be used to validate email formats, a skill often emphasized in Data Science Training for efficient data cleaning and preprocessing. This method is powerful because it checks the email format against predefined patterns that match valid email addresses.
Event Handling
Using JavaScript setCustomValidity Method JavaScript allows you to implement custom validation using the setCustomValidity() method, which can provide more complex validation than the default HTML5 form validation.
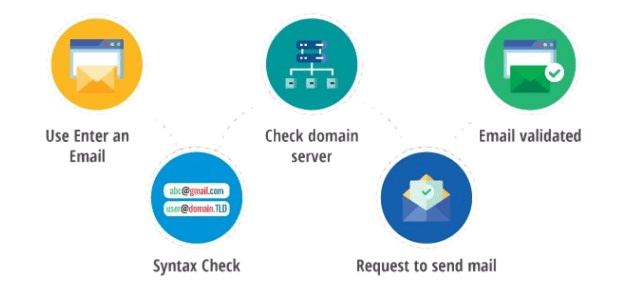
Using HTML5 Input Type
HTML5 introduced new input types that help simplify form validation, and one of the most useful for email validation is the “email” input type. When you set the input field’s type to “email”, the browser automatically checks whether the entered value follows the basic structure of an email address, such as containing an “@” symbol and a domain. This helps catch formatting errors before the form is submitted. It’s a simple yet effective way to add a layer of validation without writing any JavaScript. Most modern browsers also provide helpful error messages if the format is incorrect.
Steps to Implement Email Validation in JavaScript
Step 1: Create the HTML Form
The first step in email validation is to build a basic HTML form that allows users to enter their email address. This form includes a label for the email field, an input field set to type “email”, and a submit button. Using the “email” input type enables built-in browser validation, which checks for common formatting rules such as the presence of the “@” symbol and a valid domain name. Additionally, the “required” attribute can be added to make sure the field is not left empty. This simple structure provides a solid foundation for adding JavaScript validation later on, much like understanding the strengths and use cases in Python vs R vs SAS when choosing the right tool for data analysis. It ensures that users are prompted to enter a properly formatted email address before submitting the form. Creating a clean and functional HTML form is an essential first step in building a user-friendly and secure validation process. Once this is set up, you can enhance the experience further by implementing real-time feedback and more advanced validation techniques using JavaScript.
Gain Your Master’s Certification in Data Science by Enrolling in Our Data Science Masters Course.
Step 2: Create the JavaScript for Validation
Now, let’s implement the JavaScript function to validate the email.
Understanding Regular Expressions for Email Validation
Let’s break down the regular expression used for email validation: var regex = /^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/;
Testing the Email Validation
Once you’ve implemented email validation using JavaScript or HTML5, it’s important to thoroughly test the functionality to ensure it works correctly in different scenarios. Begin by entering a correctly formatted email address, such as “user@example.com”, and confirm that the form accepts it without any error messages. Then, test various incorrect formats to check if the validation correctly identifies and rejects them. Examples include missing the “@” symbol, missing the domain part (e.g., “user@”), or using invalid characters. Try entering an email address without a top-level domain like “.com” or with multiple “@” symbols, such as “user@@example.com” a situation where Top Python Libraries For Data Science like Pandas or NumPy can help identify and clean invalid data formats. Make sure these are flagged as invalid. Also, check how the form responds when the field is left empty, especially if it is marked as required. If you’re using JavaScript for custom validation, test edge cases and unexpected input types to see if the validation holds up. It’s also a good idea to test the validation across different browsers and devices to ensure consistency. Thorough testing helps catch potential issues early, ensures a smooth user experience, and maintains data integrity for your application.
Are You Preparing for Data Science Jobs? Check Out ACTE’s Data Science Interview Questions & Answer to Boost Your Preparation!
Conclusion
Email validation is a crucial part of building reliable and secure web forms, especially when handling user authentication, registration, or communication. Implementing JavaScript-based email validation allows you to check if users have entered a properly formatted email address before submitting the form. This helps reduce errors and improves data accuracy. In this guide, we explored different techniques for validating email addresses, from using basic regular expressions (regex) to leveraging HTML5 input types like type=”email”. You also learned how to handle common edge cases, such as missing characters or invalid domain formats, and followed best practices to make the validation process more effective skills that are crucial in Data Science Training for ensuring clean, accurate data inputs. However, it’s important to remember that client-side validation alone is not enough. Users can bypass it, so always perform server-side validation as well to ensure the data is safe and valid before processing any sensitive information. By combining both client-side and server-side validation, you can provide a smooth, user-friendly experience while maintaining the security and integrity of your web application.