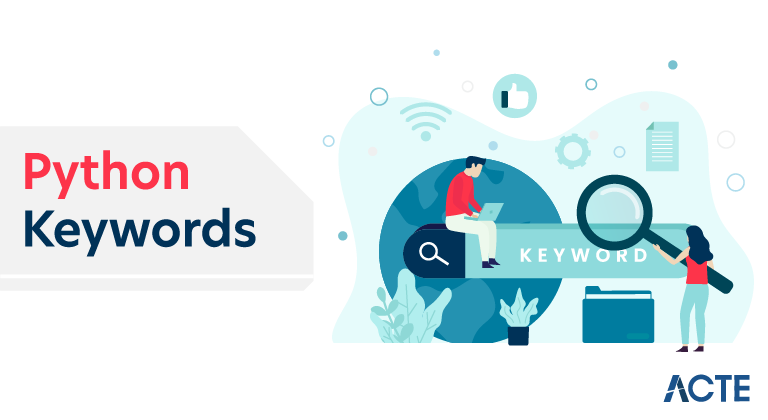
A python keyword is a reserved word which you can’t use as a name of your variable, class, function etc. These keywords have a special meaning and they are used for special purposes in Python programming language. For example – Python keyword “while” is used for while loop thus you can’t name a variable with the name “while” else it may cause compilation error.
There are total 33 keywords in Python 3.6. To get the keywords list on your operating system, open command prompt (terminal on Mac OS) and type “Python” and hit enter. After that type help() and hit enter. Type keywords to get the list of the keywords for the current python version running on your operating system.
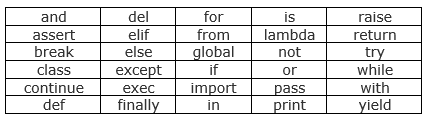
Top 24 Keywords of Python
The following are the different keywords of Python:
1. and
The word ‘and’ is reserved for Boolean or logical operations. When implying this keyword it means that an operation will be applied only when both conditions stand true.
Example:
- check_value = 5
- if check_value > 1 and check_value < 10:
- print(“Hello World!\n”)
- else:
- print(“Nothing to print”)
Output:

2. or
The word ‘or’ is also reserved for Boolean or logical operations. When implying this keyword it means that an operation will be applied even when one of the conditions stands true.
Example:
- check_value = 5
- if check_value > 1 or check_value < 10:
- print(“Hello World!\n”)
- else:
- print(“Nothing to print”)
Output:

3. not
The word ‘not’ is also reserved for Boolean or logical operations. When implying this keyword it means that an operation will be applied when the given conditional expression is not satisfied.
Example:
- check_value = 5
- if check_value not in [1,7,4,6]:
- print(“Hello World!\n”)
- else:
- print(“Nothing to print”)
Output:

4. break
A break is a loop control statement. It helps to control the execution of the loops. Specifically, the break is responsible for terminating the execution of the loop
Example:
- fruits = [“apple”, “banana”, “cherry”, “jackfruit”] for x in fruits:
- if x == “apple”:
- continue
- print(x)
- if x == “banana”:
- pass
- if x == “cherry”:
- break
- print(x)
Output:

5. continue
Continue is a loop control statement. It helps to control the execution of the loops. Specifically, Continue is responsible for switching the loop control to the condition statement again.
Example:
- def fruit_check(fruits) :
- for x in fruits:
- if x == “banana”:
- continue
- if x == “cherry”:
- return
- fruits = [“apple”, “banana”, “cherry”] object_value = fruit_check(fruits)
- print(object_value)
Output:

6. true
This keyword represents the Boolean value ‘true’.
Example:
- check_string = ‘123’
- print(check_string.isdigit())
Output:

7. false
This keyword represents the Boolean value ‘false’.
Example:
- check_string = ‘asd’
- print(check_string.isdigit())
Output:

8. if
The if keyword represents a condition instance in python.
Example:
- check_value = int(input(” Please enter the desired value : “))
- if check_value < 3:
- print (“list value : “, check_value)
Output:

9. else
The else keyword is used to represent the false execution of an if statement.
Example:
- check_value = int(input(” Please enter the desired value : “))
- if check_value < 3:
- print (“list value : “, check_value)
- else:
- print(“value is greater than 3”)
Output:

10. elif
The elif keyword is used to represent the false execution with a different additional condition check for an if statement.
Example:
- check_value = int(input(“Please enter the desired value : “))
- if check_value < 3:
- print (“list value : “, check_value)
- elif check_value > 5:
- print(“value is greater than 5”)
Output:

11. for
The for keyword is used to represent the for loop instance.
Example:
- list = [1,2,3,4] for i in list:
- print(i)
Output:

12. while
The while keyword is used to represent the while loop instance.
Example:
- check_value = int(input(“Please enter the desired value : “))
- while check_value < 10:
- print(check_value)
- check_value = check_value + 1
Output:
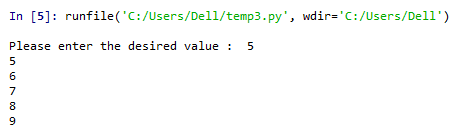
13. lambda
All anonymous functions are represented using lambda in python. anonymous functions are actually orphan functions that don’t have any name associated with them. so they will be called using the variable value assigned to them.
Example:
- Anonymous_add_function = lambda x, y : x + y >
- print(“Anonymous function output value : “, Anonymous_add_function(2, 3))
Output:

14. def
The def keyword is used for defining a function or method in python programming. the function is a block of code which can be executed.
Example:
- def fruit_check():
- fruits = [“apple”, “banana”, “cherry”] for x in fruits:
- if x == “banana”:
- continue
- if x == “cherry”:
- return
- object_value = fruit_check()
- # Function Call
- print(object_value)
Output:

15. class
Classes allow bundling of functionality, A user-defined prototype for an object that defines a set of attributes that characterize any object of the class.
Example:
- class employee:
- Firstname =”Anand”
- Lastname =”kumar”
- def sample_func(self,salary):
- print(“Firstname : ” ,self.Firstname)
- print(“salary : ” ,salary )
- salary=10000
- emp = employee()
- emp.sample_func(salary)
Output:

16. assert
A user-defined exception check in the program.
Example:
- a = 2
- try:
- assert a < 2
- print(“end”)
- except AssertionError:
- print(“Assert error triggered”)
Output:

17. except
An exception is an error happening, which takes place all through the execution of a program that interrupts the normal flow of the program’s instructions. The progression of capturing these exceptions and conduct them suitably is called exception handling. The except keyword is used for declaring the exceptions.
try: The try keyword is used to kick start the process of parsing the code for exception.
finally: The finally keyword is used to represent the block of code in the program which needs to be mandatorily executed irrespective of the error checks.
Example:
- try:
- print(“@@@@ PROGRAM TO CHECK EXCEPTION HANDELING @@@@”)
- Input1, Input2 = eval(input(“Enter two numbers, separated by a comma :”))
- #eval() will evaluate two inputs with comma sepearation is passed
- result = Input1 / Input2
- print(“Output is “, result)
- except ZeroDivisionError:
- print(“!!error in division – kindly verify the inputs !!”)
- except (IOError,TypeError):
- print(“!!Error with the input!!”)
- except SyntaxError:
- print(“Comma is missing. Enter numbers separated by comma like this 1, 2”)
- except :
- print(“!!ERROR!!”)
- else:
- print(“Exception not found”)
- finally:
- print(” Finally section”)
- print(” !———- END ————–!”)
Output:
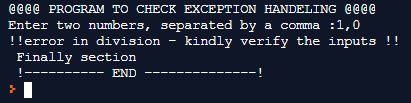
18. import
The import keyword is used for importing the necessary libraries into the program.
Example:
- import math
- # In the below given example we have used math.pi library for importing pi constant
- print( math.pi )
Output:

19. from
The import keyword is used for importing the necessary libraries into the program.
Example:
- from math import pi
- # In the below given example we have used math.pi library for importing pi constant
- print(pi)
Output:

20. global
The global keyword is used to modify the scope of the given variable. Usually mentioning a variable as global alters the variable scope from a local instance to a universal instance. So this means the variable will be available for access through the entire program at all possible instances.
Example:
- check_variable = 0 # global variable
- def add():
- global check_variable
- check_variable = check_variable + 2 # increment by 2
- print(” Value Inside add() function: “, check_variable)
- add()
- print(” Value In main function :”, check_variable)
Output:

21. exec
The functions is intended for the vibrant implementation of the Python program which can moreover be object code or else a specific string. In the case of a string, the string is parsed as a group of Python statements, which intern is executed until any python oriented errors are populated in the program in an object code perspective just a static execution is been carried out. We have to be cautious so as to the go back statements might not be worn external of function definitions which are not still contained by the background of code agreed to the exec() function. Additionally, instead of returning any value it actually returns None.
Example:
- from math import *
- print(“below are the list of functions in comments”)
- print(” “)
- math_list = [‘Anonymous_add_function’, ‘In’, ‘Out’, ‘_’, ‘__’, ‘___’, ‘__builtin__’, ‘__builtins__’, ‘__doc__’, ‘__file__’, ‘__loader__’, ‘__name__’, ‘__package__’, ‘__spec__’, ‘_dh’, ‘_i’, ‘_i1’, ‘_i10’, ‘_i11’, ‘_i12’, ‘_i13’, ‘_i14’, ‘_i15’, ‘_i16’, ‘_i17’, ‘_i18’, ‘_i19’, ‘_i2’, ‘_i20’, ‘_i21’, ‘_i22’, ‘_i23’, ‘_i24’, ‘_i25’, ‘_i3’, ‘_i4’, ‘_i5’, ‘_i6’, ‘_i7’, ‘_i8’, ‘_i9’, ‘_ih’, ‘_ii’, ‘_iii’, ‘_oh’, ‘a’, ‘acos’, ‘acosh’, ‘add’, ‘asin’, ‘asinh’, ‘atan’, ‘atan2’, ‘atanh’, ‘ceil’, ‘check_value’, ‘check_variable’, ‘copysign’, ‘cos’, ‘cosh’, ‘degrees’, ‘e’, ’emp’, ’employee’, ‘erf’, ‘erfc’, ‘exit’, ‘exp’, ‘expm1’, ‘fabs’, ‘factorial’, ‘floor’, ‘fmod’, ‘frexp’, ‘fruit_check’, ‘fsum’, ‘gamma’, ‘gcd’, ‘get_ipython’, ‘hypot’, ‘inf’, ‘isclose’, ‘isfinite’, ‘isinf’, ‘isnan’, ‘ldexp’, ‘lgamma’, ‘log’, ‘log10’, ‘log1p’, ‘log2’, ‘math’, ‘modf’, ‘nan’, ‘np’, ‘num1’, ‘num2’, ‘object_value’, ‘pd’, ‘pi’, ‘plt’, ‘pow’, ‘quit’, ‘radians’, ‘result’, ‘salary’, ‘sin’, ‘sinh’, ‘sqrt’, ‘tan’, ‘tanh’, ‘tau’, ‘trunc’] print(math_list)
- print(” “)
- print(“below are the functions from exec() method”)
- print(” “)
- exec(“print(dir())”)
Output:
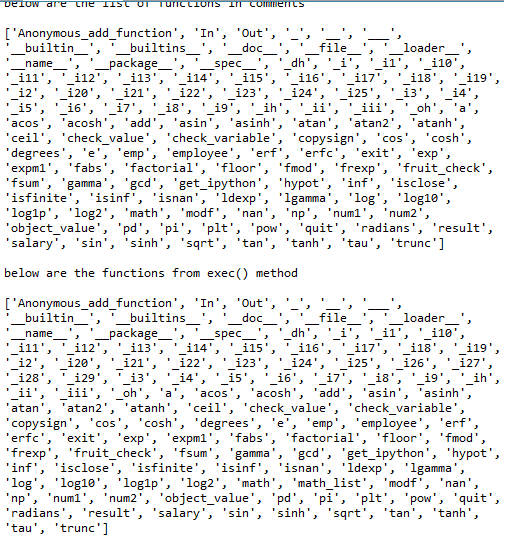
22. print
The print keyword is used for displaying output in the terminal
Example:
- import math
- print( ” !PYTHON CONSTANS! “)
- print(” PI value : ” , math.pi)
- print(” E value : ” , math.e)
- print(” nan value : ” , math.nan)
- print(” E value : ” , math.inf)
Output:
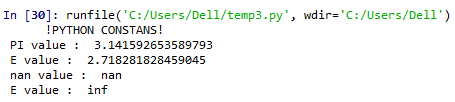
23. return
The return keyword is used for returning some value as an output from a function. If no return value is specified a function by default returns none.
Example:
- def fruit_check(fruits) :
- for x in fruits:
- if x == “banana”:
- continue
- if x == “cherry”:
- return x
- fruits = [“apple”,”cherry”,”banana” ] object_value = fruit_check(fruits)
- print(object_value)
Output:

24. yield
The yield declaration hangs up function’s implementation, in addition, to propel a value to the respective caller, but retains an adequate amount of circumstances to facilitate function to take up again anywhere it is missing off. When recommence, the function carries on execution right away following the most recent yield run. This permits its system to construct a sequence of standards over time, rather them calculate at once and sending them back like a list.
Example:
- def yieldFun():
- yield 1
- yield 2
- yield 3
- # Driver code to check above generator function
- for value in yeildFun():
- print(value)
Output:

Conclusion
Python holds a very sophisticated set of reserved words with it. The major advantage with this programming language is that it holds a lesser number of keywords which makes it a very efficient programming outfit to choose upon.