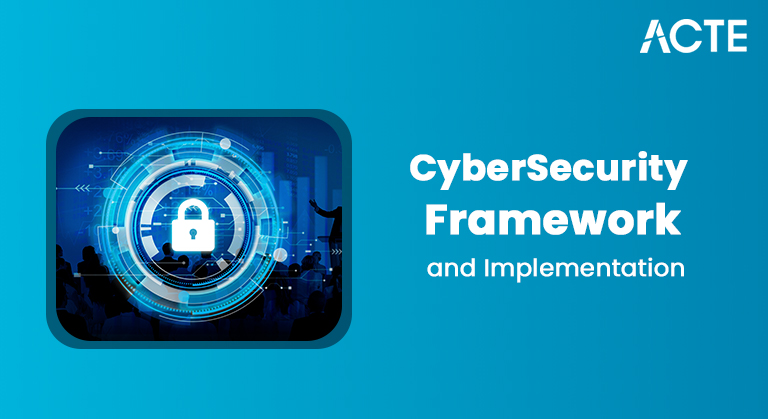
- What Is an Algorithm?
- What Makes the Algorithm the Best Choice?
- Using the A Algorithm’s Pseudocode
- Benefits of AI’s A* Algorithm
- The A* Algorithm’s Code
- The A* Algorithm’s Drawbacks in AI
- The A* Algorithms Used in artificial intelligence
- Conclusion
What Is an Algorithm?
The A* algorithm is a search algorithm designed to find the shortest path from a starting point to a goal. It is part of the informed search algorithms family, as it uses a heuristic to guide the search process more intelligently, rather than blindly exploring all possible paths. A* combines the strengths of two well-known algorithms: Dijkstra’s Algorithm and Greedy Best-First Search. Dijkstra’s Algorithm guarantees finding the shortest path by systematically exploring all nodes in the graph. However, it doesn’t consider the destination, making it inefficient in large search spaces, a limitation discussed in Data Science Training. On the other hand, Greedy Best-First Search uses a heuristic to prioritize nodes that appear closest to the goal, but it doesn’t account for the cost of the path taken so far, which can lead to suboptimal results. By combining these two approaches, A* is able to efficiently find the shortest path, while also intelligently prioritizing nodes based on both the path cost and proximity to the goal. This balance makes A* both optimal and efficient for pathfinding tasks.
Are You Interested in Learning More About Data Science? Sign Up For Our Data Science Course Training Today!
What Makes the Algorithm the Best Choice?
The A* algorithm is widely considered one of the best choices for pathfinding and search problems due to several key characteristics:
- Optimality and Completeness: A* guarantees it will find the shortest path to the goal, given that the heuristic is admissible (it never overestimates the cost). This makes it ideal for situations requiring an optimal solution, such as GPS navigation or robot movement.
- Efficiency: A* is more efficient than Dijkstra’s algorithm because it uses a heuristic to prioritize which nodes to explore. While Dijkstra’s algorithm explores every possible node, A* intelligently narrows its search to focus on the most promising paths. This allows it to find the solution more quickly.
- Flexibility: Adjusting the heuristic function can adapt the A* algorithm to various applications. The heuristic can be designed to fit specific environments, such as grid-based maps or 3D spaces, a concept closely tied to What is Data Analysis.
- Heuristic Guidance: By incorporating a heuristic, A* can more effectively guide its search process toward the goal. The heuristic function helps the algorithm estimate the cost of getting from the current node to the goal, enabling it to make decisions that bring it closer to the goal while avoiding unnecessary exploration.
- Handling Dynamic Environments: A* is capable of adapting to dynamic environments where obstacles or conditions may change during the search. With minor adjustments to the heuristic or cost function, A* can continue to find the shortest path, even when the environment evolves in real-time, making it suitable for applications like autonomous vehicles or real-time strategy games.
- Memory Usage: One trade-off with A* is its memory usage. Since it stores all explored nodes in memory, it can become memory-intensive, especially for large or complex search spaces. However, this comprehensive storage ensures that A* can track the best path efficiently, and various optimizations like iterative deepening A* (IDA*) are available to mitigate memory usage in large-scale problems.
- function A*(start, goal)
- open list = {start}
- closed-list = {}
- g(start) = 0
- h(start) = heuristic(start, goal)
- f(start) = g(start) + h(start)
- while the open list is not empty
- current = node in open list with the lowest f value
- if current == goal
- return reconstruct_path(current)
- remove current from the open list
- add current to the closed list
- for each neighbor of the current
- if a neighbor in the closed list
- continue
- tentative_g = g(current) + dist_between(current, neighbor)
- if the neighbor is not on the open list
- add neighbor to open-list
- else if tentative_g >= g(neighbor)
- continue
- parent(neighbor) = current
- g(neighbor) = tentative_g
- h(neighbor) = heuristic(neighbor, goal)
- f(neighbor) = g(neighbor) + h(neighbor)
- return failure
- Optimal Pathfinding: Given an admissible heuristic, a* guarantees the shortest path.
- Efficiency: A* is more efficient than many other algorithms using the heuristic function.
- Flexibility: The heuristic function can be customized to fit various applications.
- Widely Used: It has been successfully applied in robotics, gaming, and route optimization, showcasing its potential in the Future Scope of Machine Learning.
- Handling Complex Constraints: A* can accommodate additional constraints, such as avoiding certain areas or factoring in variable terrain costs, making it useful in environments with more complex requirements.
- Real-Time Performance: In many scenarios, A* is optimized for real-time performance. By using techniques like pruning or iterative deepening, it can find solutions quickly enough for applications that require immediate results, such as robotic navigation or gaming AI.
- Scalability: A* scales well to larger problems, as it can efficiently handle expanded search spaces, making it applicable to everything from small-scale pathfinding to large-scale network routing.
- import heapq
- def heuristic(a, b):
- return abs(a[0] – b[0]) + abs(a[1] – b[1])
- def start(start, goal, grid):
- open_list = []
- heapq.help push(open_list, (0, start))
- g_costs = {start: 0}
- f_costs = {start: heuristic(start, goal)}
- came_from = {}
- while open_list:
- _, current = heapq.heappop(open_list)
- if current == goal:
- path = []
- while current in came_from:
- path.append(current)
- current = came_from[current]
- return path[::-1] # Return reversed path
- for neighbor in get_neighbors(current, grid):
- tentative_g_cost = g_costs[current] + 1 # Assuming each move has a cost of 1
- if neighbor not in g_costs or tentative_g_cost < g_costs[neighbor]:
- came_from[neighbor] = current
- g_costs[neighbor] = tentative_g_cost
- f_costs[neighbor] = tentative_g_cost + heuristic(neighbor, goal)
- heapq.heappush(open_list, (f_costs[neighbor], neighbor))
- return None
- def get_neighbors(node, grid):
- neighbors = []
- x, y = node
- directions = [(-1, 0), (1, 0), (0, -1), (0, 1)]
- For dx, dy in directions:
- nx, ny = x + dx, y + dy
- if 0 <= nx < len(grid) and 0 <= ny < len(grid[0]) and grid[nx][ny] != 1:
- neighbors.append((nx, NY))
- return neighbors
- Memory Consumption: A* can require much memory, especially when the search space is ample.
- Heuristic Dependency: The performance of A* heavily depends on the quality of the heuristic function. A poor heuristic can reduce the algorithm’s efficiency.
- Not Ideal for Dynamic Environments: A* assumes that the environment is static and known ahead of time. It may struggle to adapt to dynamic or changing environments.
- Slow with Large Search Spaces: A* can become slower in situations with large and complex search spaces, as it needs to explore a significant number of nodes to find the optimal path, a challenge that can be addressed using techniques similar to those in Simple Linear Regression for optimization.
- Overhead in Complex Scenarios: In highly complex scenarios with numerous constraints, A* might incur significant computational overhead. The time and memory requirements can grow quickly as more variables or constraints are added, making it inefficient in certain contexts.
- Risk of Getting Stuck in Local Minima: Although A* is designed to find the optimal path, it can sometimes get stuck in local minima if the heuristic is not well-tuned or if the search space has unexpected complexities. This is especially true in situations with poorly designed or misleading heuristics.
- Limited Handling of Uncertainty: A* operates under the assumption of known, deterministic environments. It is not inherently capable of handling uncertainty or probabilistic environments, such as those found in robotic navigation with sensor noise or uncertain map data.
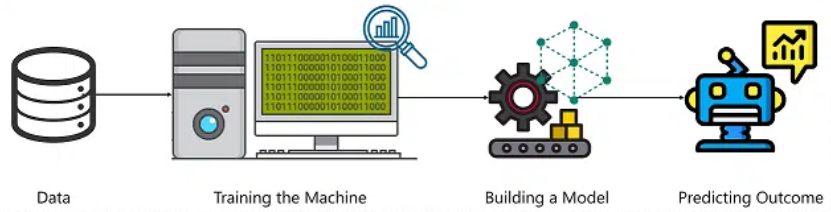
Using the A Algorithm’s Pseudocode*
The A* algorithm efficiently finds the shortest path by combining the benefits of Dijkstra’s algorithm and heuristic-guided search, a technique often explored in Data Science Training. It evaluates nodes based on both their distance from the start and estimated distance to the goal. Here’s a simplified version of the pseudocode for the A* algorithm:
The pseudocode for the A* algorithm outlines the process of finding the shortest path from a start node to a goal node. It begins by initializing two lists: open (nodes to be evaluated) and closed (nodes already evaluated). The algorithm selects the node with the lowest f-score (the sum of the cost to reach the node and the estimated cost to the goal) from the open list. It then explores neighboring nodes, calculates their f-scores, and updates the lists accordingly. This process continues until the goal node is reached or all possibilities are exhausted.
Want To Obtain Your Data Science Certificate? View The Data Science Course Training Offered By ACTE Right Now!
Benefits of AI’s A* Algorithm
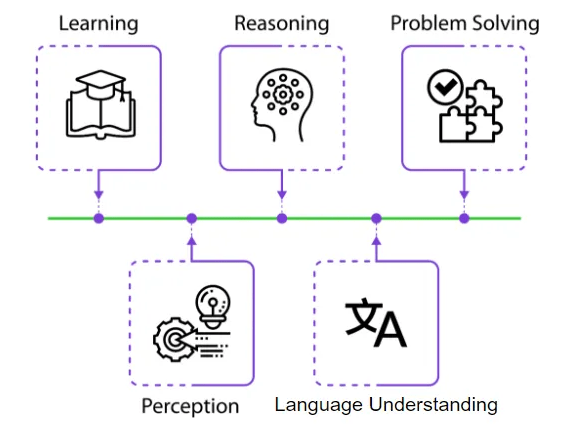
The A* Algorithm’s Code
Before we dive into the Python implementation of the A* algorithm, let’s first understand how it works in a 2D grid, a concept that can be linked to Dispersion in Statistics when analyzing spatial data. A* efficiently finds the shortest path by combining the advantages of both Dijkstra’s algorithm and greedy best-first search. Here is a simple implementation of the A* algorithm in Python using a 2D grid as an example:
Are You Considering Pursuing a Data Science Master’s Degree? Enroll For Data Science Masters Course Today!
The A* Algorithm’s Drawbacks in AI
The A* Algorithms Used in artificial intelligence
A* is widely used in various applications that require efficient pathfinding. In robotics, it helps robots navigate through obstacle-filled environments by calculating the shortest path from their current location to a goal. The algorithm is also integral to AI in gaming, where it allows characters or enemies to find the most optimal route across a map, avoiding obstacles and other entities. Another common application is in route planning, particularly in GPS navigation systems, where A* is used to determine the shortest driving or walking path, taking into account factors like distance, traffic, or road types, a concept that can be optimized using techniques like Ridge and Lasso Regression Explained Using Python. Additionally, A* is utilized in AI simulations within virtual environments, where AI agents or characters need to navigate between points efficiently, ensuring realistic movement and decision-making. These applications highlight A*’s versatility and its ability to find optimal paths in various dynamic and complex scenarios, making it an essential tool in fields ranging from robotics to gaming and navigation systems.
Want to Learn About Data Science? Explore Our Data Science Interview Questions & Answer Featuring the Most Frequently Asked Questions in Job Interviews.
Conclusion
The A* algorithm is a robust and versatile tool in artificial intelligence, combining the optimality of Dijkstra’s algorithm with the heuristic-driven search of the Greedy Best-First Search. This combination makes A* highly effective for solving pathfinding and search problems. By carefully selecting and tuning the heuristic function, A* can be applied across a wide range of real-world scenarios, such as robotics, gaming, and automated navigation systems. A* excels in efficiently finding the shortest path while intelligently guiding the search, prioritizing promising paths to improve speed, a concept often covered in Data Science Training. However, it does come with certain drawbacks, such as high memory consumption and a strong dependency on the quality of the heuristic used. A poor heuristic can slow down the process or reduce efficiency. Despite these limitations, the A* algorithm remains a top choice for pathfinding problems, offering a balance between optimality and computational efficiency, making it invaluable in fields that require fast, accurate, and reliable pathfinding solutions.