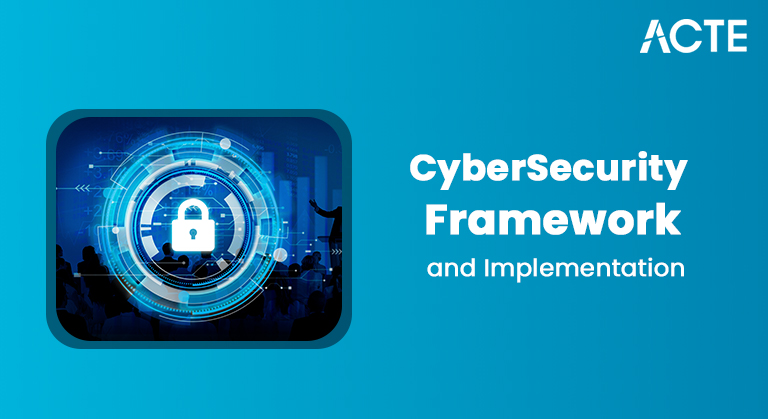
- Introduction
- What is the pass Keyword in Python?
- Why Would You Use pass?
- Basic Syntax of pass
- Common Use Cases for pass
- Example: Using pass in Python
- When to Avoid Using pass
- Conclusion
Introduction
For individuals who are unfamiliar with Python, the keyword pass may appear perplexing at first. It is challenging to comprehend its purpose because, at first appearance, it is a no-op (no operation) command that does nothing when performed. But when you learn how it works, you’ll see that pass is a placeholder in your code. It is employed when a syntactically necessary statement needs to be made without executing any operations. This may occur when you define an empty class, method, or loop with the intention of adding functionality later. Rather than leaving the code block empty, which would result in an error, you use the pass to ensure the structure remains valid. Gaining knowledge about when and why to use a pass provides several opportunities for your coding practice, especially when paired with Data Science Training to build robust and efficient programs. As you progress through the various stages of development, you will be able to preserve the integrity of your code. For instance, you may use a pass as a placeholder to signal that specific code sections will be finished later when prototyping or organizing a function or class hierarchy. Doing so lets you focus on other parts of your program without worrying about syntax issues or empty blocks. Ultimately, pass is a small but powerful tool that ensures your code remains organized and functional, even when parts of it are still under construction.
Eager to Acquire Your Data Science Certification? View The Data Science Course Offered By ACTE Right Now!
What is the Pass Keyword in Python?
In Python, the pass keyword serves as a placeholder that allows you to define a block of code without any actual functionality. It essentially performs no action, making it useful when you need to satisfy the syntax requirements of the language but don’t want the code to do anything immediately. This can seem a bit odd at first, as it seems like a “do nothing” operation, but it plays a crucial role in keeping your code structure intact while you decide how to implement the functionality in Python Keywords. The primary benefit of using pass arises when you want to create a skeleton of your program or define areas that will be filled in later. It helps in setting up stubs for parts of the code, making it easy to structure your program in a way that allows for future development. This is especially helpful in larger projects where you may need to lay out the overall framework before diving into the specific details of implementation.
Why Would You Use a Pass?
- Placeholder for future code: When you’re sketching out the structure of your program and want to leave certain parts unfinished or to be filled in later.
- Empty function or method: Sometimes, you should define a function or process that doesn’t do anything yet, but you still need to include it for future Devops Implementation.
- Control structures with no action: Sometimes, like loops or conditionals, you might need to define a body that does nothing for logical reasons.
- Minimalist error handling: Sometimes, you may want to handle specific exceptions without doing anything.
Pass allows you to avoid syntax errors while leaving clear intentions for future development.
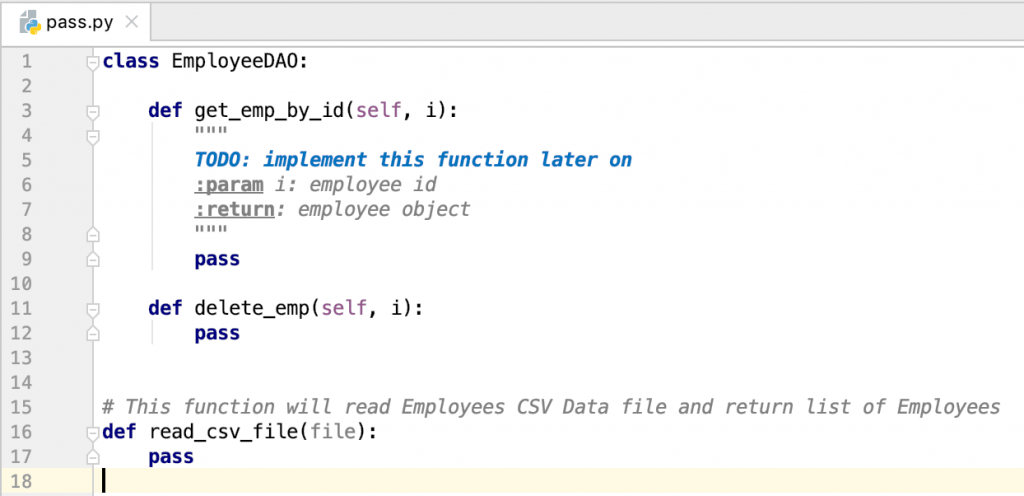
Basic Syntax of pass
In Python, the pass statement is a special keyword used when a statement is required syntactically, but you don’t want to execute any code. It acts as a placeholder and literally does nothing when the program runs. This can be particularly helpful during the development phase when you’re still planning your code. For example, if you’re defining a function, a class, or writing an if statement and haven’t written the actual logic yet, using pass prevents Python from throwing an error due to an empty code block. Without it, Python expects some kind of instruction, and leaving the block empty would result in an IndentationError or SyntaxError. By writing a pass, you’re telling Python that you’re intentionally leaving that section blank for now, and you’ll come back to complete it later, a practice that aligns well with the iterative process in Data science Training. It helps keep your code structure organized and error-free even when parts of your program are incomplete. The pass statement is often used in loops, exception handling blocks, and class declarations when the actual implementation is pending or when you want to skip execution in certain cases temporarily. It’s also useful in situations where you need your code to run but you don’t want any specific action to take place for a particular condition — acting as a “do nothing” response that satisfies Python’s requirement for syntactically correct code blocks.
Excited to Obtaining Your Data Science Certificate? View The Data Science Training Offered By ACTE Right Now!
Everyday Use Cases for pass
Inside Empty Functions
If you’re defining a function but don’t have the code written out yet, you can use the pass to prevent an error. This is especially useful when you’re working with incomplete code or scaffolding out your program:
- def my_function():
- pass
In this case, my_function() does nothing but still satisfies Python’s requirement for a function body. You can replace the pass with the actual code when you implement it.
Placeholder in Conditional Statements
Sometimes, you might have a conditional statement (like an if, else, or elif) where you want to empty one of the branches. Instead of leaving it blank and causing a syntax error, you can use pass:
- x = 10
- if x > 5:
- pass # Do nothing for now
- else:
- print(“x is less than or equal to 5”)
In this case, the program does not ng if x exceeds 5. The pass ensures that Python does not make a syntax error when It expects an indented block.
Interested in Pursuing Data Science Master’s Program? Enroll For Data Science Master Course Today!
Empty Classes or Methods
When defining a class or method that will be implemented later, you can use the pass to indicate that it’s a placeholder. This is especially common during the early stages of software design.
- Class MyClass:
- pass
- def my_method():
- pass
In the example above, the class MyClass and the method my_method() do nothing for now but can be fleshed out later.
Loop Placeholder
Sometimes, you should iterate over a loop but have no action inside the loop. Using pass allows you to define an empty loop that will perform no actions:
- for i in range(5):
- pass # Loop runs, but nothing happens
This could be useful when setting up the loop structure but hasn’t decided what actions to take inside the loop.
Preparing for a Data Science Job Interview? Check Out Our Blog on Data Science Interview Questions & Answer
Example: Using pass in Python
Let’s consider a practical example where the pass is used in a function to create an outline for a program:
- def handle_user_input(user_input):
- if user_input == ‘start’:
- print(“Starting the program…”)
- elif user_input == ‘stop’:
- print(“Stopping the program…”)
- else:
- Pass # Handle unknown input later
- # Simulating user input
- handle_user_input(‘unknown’) # This will do nothing for now
In the above example, the else block handles unknown user input, but for now, we don’t have any logic to handle it. We use the pass as a placeholder; later, we could add a message or error handling in that section.
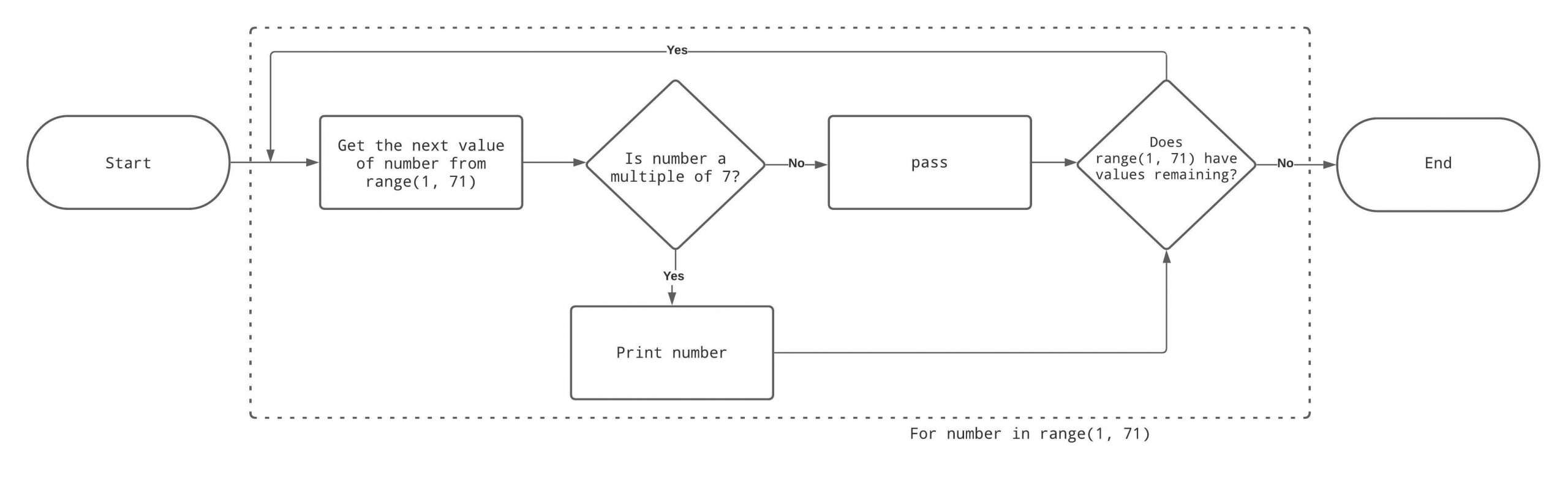
When to Avoid Using pass
- Unnecessary use: If you’re putting pass in areas where you don’t need it (e.g., in empty loops or functions that aren’t part of the code you’re still developing), you may end up with overly complex or confusing code, especially when working with Identifiers in Python. It’s better to leave the area blank or remove unnecessary placeholders when possible.
- Clarity of code: Overusing passes can make your code more challenging to read, especially for beginners. Always try to use passes in a way that makes your intentions clear. For example, using the pass as a placeholder might indicate that you haven’t implemented that part yet, but too many pass statements could clutter the code.
Conclusion
Pass is a simple but essential keyword for structuring your code in Python. Whether you’re defining empty functions, skipping certain conditions, or leaving placeholders in your program, pass helps you avoid syntax errors and build code incrementally. While it’s a no-op in terms of execution, it has the valuable role of maintaining your program’s flow and integrity as you continue developing your code, particularly when applying concepts from Data Science Training. As a beginner, understanding when and where to use pass will make writing straightforward and maintainable code easier, especially during the early stages of software design. So, next time you encounter an incomplete section of code or need to avoid a syntax error, consider using a pass to keep things moving smoothly.