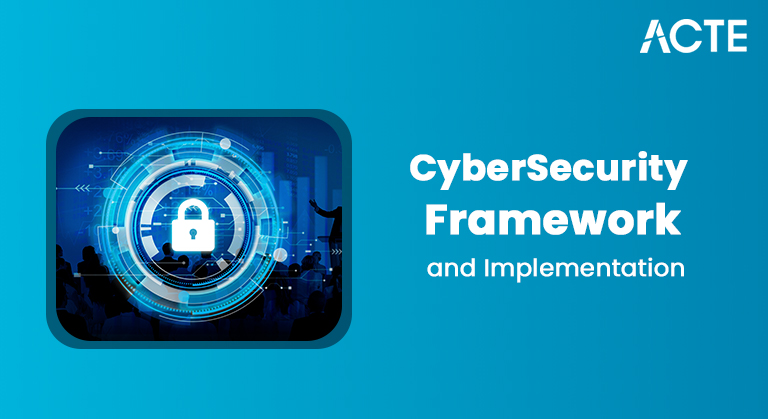
- Introduction to Python for Data Science
- Key Features of Python
- Interpreted and Dynamically Typed Language
- Python’s Simplicity and Readability
- Object-Oriented and Functional Programming
- Extensive Standard Library
- Cross-Platform Compatibility
- Garbage Collection and Memory Management
- Support for GUI Programming
- Integration with Other Languages
- Popular Python Libraries and Frameworks
- Future Trends in Python Development
Introduction to Python for Data Science
Python is a high-level, interpreted programming language that has gained immense popularity among developers, data scientists, and engineers across various industries. Designed by Guido van Rossum and released in 1991, Python Development is celebrated for its simplicity, readability, and flexibility, making Data Science Course Training an ideal choice for beginners and professionals. Python’s diverse applications range from web development and automation to data analysis and artificial intelligence (AI). Python for Data Science is recognized as one of the most versatile programming languages. Its extensive ecosystem of libraries and frameworks enables developers to build everything from simple scripts to complex machine-learning models. This introduction will explore Python’s key features, core characteristics, and the reasons for its widespread use.
Key Features of Python
- Simplicity and Readability: Python emphasizes readability, making it easy to write, understand, and maintain code. Its syntax is clear, concise, and minimalistic, allowing developers to express concepts in fewer lines of code than other languages.
- Versatility: Python is a general-purpose language in various domains, including web development, data science, automation, scripting, and artificial intelligence. Whether you’re building Elasticsearch vs Solr , analyzing big data, or creating a chatbot, Python can handle many tasks.
- Extensive Libraries and Frameworks: Python comes with a comprehensive set of built-in libraries and frameworks that support different tasks, such as web development (Flask, Django), data analysis (Pandas, NumPy), machine learning (TensorFlow, sci-kit-learn), and more.
- Comunity Support: Python Development has a large and active community of developers. This community contributes to its continuous improvement and provides many resources, including tutorials, forums, and open-source projects.
- Cross-Platform Compatibility: Python is platform-independent, meaning code written in Python can run on different operating systems (Windows, macOS, Linux) without modification.
Learn the fundamentals of Data Science with this Data Science Online Course .
Interpreted and Dynamically Typed Language
- Interpreted Language: Python is an interpreted language that does not require compilation before execution. Instead of compiling code into machine language, an interpreter executes Python code line-by-line. This approach allows for faster development and testing cycles, as developers can immediately see the results of their code changes.
- Dynamically Typed: Python is also dynamically typed, meaning that you do not need to declare the data type of a variable when defining it. Python automatically assigns data types based on the values assigned to variables. This makes the language more flexible and allows for rapid development, but Data Science Course Training can lead to potential runtime errors if types are mismatched. This dynamic nature enhances code flexibility but requires the developer to be careful with variable assignments to avoid type-related issues during runtime.
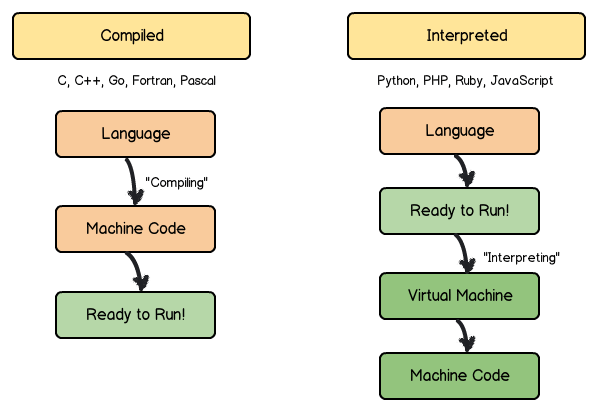
Python’s Simplicity and Readability
One of Python’s most celebrated aspects is its simplicity and readability. Unlike many other programming languages requiring complex syntax, Python uses a clean and straightforward syntax closely resembling English. Garbage Collection characteristic makes it an excellent choice for beginners who are learning to code.
Key features contributing to Python’s readability:
- Indentation-based Block Structure: Python uses indentation (whitespace) to define code blocks, such as loops, functions, and conditionals, rather than relying on curly braces or other delimiters. Data Wrangling reduces visual clutter and encourages consistent code formatting.
- Minimalist Syntax: Python emphasizes brevity, allowing developers to achieve more with fewer lines of code. This can lead to more efficient and easier-to-maintain code.
- Encapsulation: Bundling data and methods that operate on the data into a single unit (i.e., a class).
- Inheritance: Deriving new classes from existing ones to promote code reuse.
- Polymorphism: Allowing objects of different classes to be treated as instances of the same class through method overriding.
- Class Dog:
- def __init__(self, name, breed):
- self.name = name
- self.breed = breed
- def bark(self):
- print(f”{self.name} is barking!”)
- dog1 = Dog(“Buddy,” “Golden Retriever”)
- dog1.bark()
- Os: Operating system interface for file and directory manipulation.
- Sys: System-specific parameters and functions.
- Math: Mathematical functions, including trigonometric and logarithmic operations.
- DateTime: Working with dates and times.
- json: Encoding and decoding JSON data.
- Re: Regular expressions for pattern matching in strings.
- PyQt: A set of Python bindings for the Qt toolkit, enabling the creation of advanced and cross-platform desktop applications.
- wxPython: Another popular choice for building cross-platform GUI applications.
- Cython: A superset of Python that allows you to write C extensions for Python to improve performance.
- Python-C API: Allows developers to extend Python with C modules.
- Jython: Python implementation on the Java Virtual Machine (JVM), enabling Python to interact with Java code.
- PyObjC: A bridge that allows Python to interact with Objective-C (macOS and iOS).
- Django and Flask (Web Development): Django is a high-level web framework that follows the “batteries-included” philosophy, while Flask is a Pandas vs Numpy micro-framework suitable for smaller projects.
- NumPy and Pandas (Data Science): NumPy supports large, multi-dimensional arrays, and Pandas is used for data manipulation and analysis.
- TensorFlow and PyTorch (Machine Learning/artificial intelligence): These libraries provide tools for deep learning, neural networks, and artificial intelligence development.
- Matplotlib and Seaborn (Data Visualization): These libraries are widely used to create static, animated, and interactive visualizations in Python..
- BeautifulSoup (Web Scraping): A popular library for extracting data from HTML and XML documents.
- Machine Learning and AI: Python is becoming the dominant language for machine learning and artificial intelligence. Libraries like TensorFlow, Keras, and sci-kit-learn are pushing Python’s role in artificial intelligence research and production.
- Data Science and Big Data: Python is a go-to data science and analysis language. With frameworks like Pandas, Dask, and Apache Spark, Data Science Course Training can handle large-scale data processing.
- Python for IoT (Internet of Things):Python’s simple syntax and powerful libraries are increasingly used in IoT applications. MicroPython, a variant of Python designed for microcontrollers, is being used in embedded systems.
- Python for Cloud Computing: As cloud platforms become more prevalent, Python is increasingly used to write cloud-native applications and scripts for cloud infrastructure management.
Dive into Data Science by enrolling in this Data Science Online Course today.
Object-Oriented and Functional Programming
Python supports object-oriented programming (OOP) principles, allowing developers to define classes and objects. OOP encourages code reuse, modularity, and the organization of complex systems into manageable components.
Python’s support for OOP allows for:
Example of a class in Python:
Functional Programming:
Python also supports functional programming features, where functions can be used as first-class citizens, passed as arguments, and returned from other functions. Python’s built-in map(), filter(), and reduce() functions, along with lambda functions, make functional programming easy and concise.
Extensive Standard Library
Python’s standard library is one of its greatest strengths. Calculator Using Javascript includes a vast collection of modules and packages that allow developers to perform tasks without writing code from scratch. Some of the most notable libraries in Python’s standard library include:
Take charge of your Data Science career by enrolling in ACTE’s Data Science Master Program Training Course today!
Cross-Platform Compatibility
Python is cross-platform, meaning code written in Python can run on different operating systems, such as Windows, macOS, and Linux, without modification. This is one of the key reasons Python is popular in fields like web development, automation, and scientific computing, where applications must run in diverse environments. The Python interpreter facilitates Python’s cross-platform nature by abstracting the underlying platform details, allowing developers to write code that is portable across operating systems.
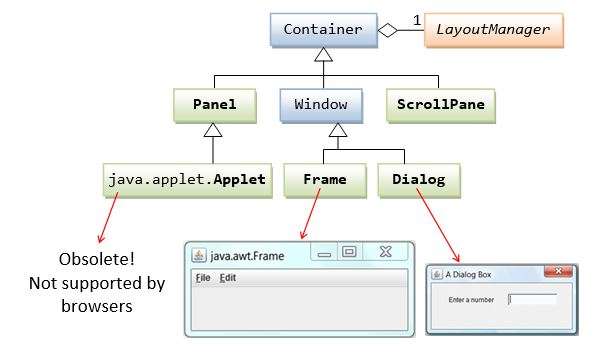
Garbage Collection and Memory Management
Python features automatic memory management, which includes garbage collection. The Python runtime automatically manages memory allocation and deallocation for objects, freeing up memory no longer in use. The garbage collector identifies and removes objects no longer referenced in the program, reducing the risk of memory leaks and ensuring efficient usage. While Python’s memory management is largely automated, developers can still control it using tools like the GC (garbage collection) module to manage objects when necessary manually.
Support for GUI Programming
Python offers robust support for creating graphical user interfaces (GUIs). Several libraries and frameworks make Seaborn in Python easy to design and implement GUI-based applications. Some of the most popular libraries for GUI programming in Python include: Tkinter: The standard Python interface to the Tk GUI toolkit. It is easy to use and comes bundled with Python.
These libraries allow developers to create user-friendly applications with buttons, text boxes, labels, and other interactive elements.
Integration with Other Languages
Python’s flexibility extends to its ability to integrate with other programming languages. Python can easily interface with C, C++, Java, and other languages, allowing developers to use Web Crawler code written in these languages and extend Python’s capabilities. Python provides several ways to interact with other languages:
This cross-language interoperability is useful in various contexts, such as leveraging libraries written in other languages or optimizing performance-critical components of an application.
Want to ace your Data Science interview? Read our blog on Data Science Interview Questions and Answers now!
Popular Python Libraries and Frameworks
Python for Data Science has a vast library and framework ecosystem, enabling developers to build sophisticated applications across various domains quickly. Some of the most popular libraries and frameworks include:
Future Trends in Python Development
As Python continues to evolve, several trends are emerging in its development landscape: