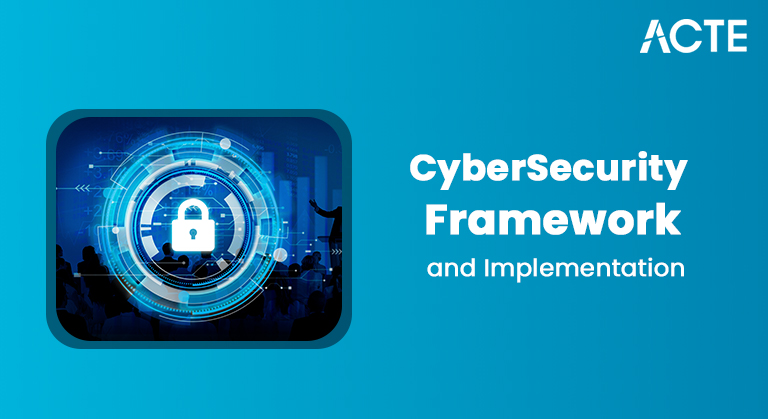
- Introduction to Aggregate Functions
- Common Aggregate Functions (SUM, AVG, COUNT, etc.)
- Using GROUP BY with Aggregate Functions
- HAVING Clause vs WHERE Clause
- Nested Aggregate Functions
- Using Aggregate Functions with Joins
- Window Functions vs Aggregate Functions
- Use Performance Optimization for Aggregate Queries
- Handling NULL Values in Aggregates
- Real-World Applications of Aggregate Functions
- Best Practices for SQL Aggregation
- Conclusion
Introduction to Aggregate Functions
In SQL, aggregate functions are essential tools used to perform calculations across multiple rows of data, returning a single, summarized value. These functions are particularly valuable in the context of data analysis, reporting, and decision-making, as they allow users to condense large volumes of data into meaningful insights. Commonly used alongside the GROUP BY clause, aggregate functions enable the grouping of records based on specific columns, so calculations can be performed within each group rather than on the entire dataset. This is especially useful in Data Science Training when analyzing trends or comparing values across categories like departments, regions, or time periods. Aggregate functions such as sum, average, count, minimum, and maximum help in operations like totaling sales, calculating average income, counting entries, or identifying extreme values in data. Through these tools, SQL empowers users to derive patterns and summaries that inform strategies, highlight performance, and support effective data-driven decisions.
Eager to Acquire Your Data Science Certification? View The Data Science Course Offered By ACTE Right Now!
Common Aggregate Functions (SUM, AVG, COUNT, etc.)
- SUM(): Calculates the total sum of a numeric column.
- SELECT SUM(sales_amount) FROM orders;
- AVG(): Computes the average value of a numeric column.
- SELECT AVG(age) FROM employees;
- COUNT(): Counts the number of rows in a dataset or the number of non-NULL values in a column.
Example:
- SELECT COUNT(*) FROM products;
This returns the total number of rows in the products table.
- MIN(): Finds the minimum value in a column.
Example:
- SELECT MIN(price) FROM products;
This returns the lowest price in the products table.
- MAX(): Finds the maximum value in a column.
Example:
- SELECT MAX(salary) FROM employees;
This returns the highest salary in the employee’s table.
- GROUP_CONCAT() (MySQL specific): Concatenates values from a column into a single string.
Example:
- SELECT GROUP_CONCAT(product_name) FROM products;
This returns a concatenated string of all product names in the products table.
These aggregate functions are often used with grouping (GROUP BY), filtering (HAVING), and other clauses to aggregate and summarize data.
Using GROUP BY with Aggregate Functions
The GROUP BY clause is combined with aggregate functions to group rows with the same value in one or more columns. This is especially useful for calculating totals or averages for specific categories.
Example:
- SELECT department, AVG(salary) AS average_salary
- FROM employees
- GROUP BY department;
This query groups the employees by department and calculates the average salary for each department.
Important Notes:
- You must specify the columns in which you want to group the data in the GROUP BY clause.
- You can group by multiple columns to create more specific aggregations.
- SELECT department, AVG(salary)
- FROM employees
- WHERE salary > 50000
- GROUP BY department;
- SELECT department, AVG(salary) AS average_salary
- FROM employees
- GROUP BY department
- HAVING AVG(salary) > 60000;
- WHERE is used to filter rows before aggregation.
- HAVING is used to filter groups after aggregation.
- SELECT MAX(AVG(salary))
- FROM employees
- GROUP BY department;
- SELECT department, AVG(salary)
- FROM employees
- WHERE salary > (SELECT AVG(salary) FROM employees);
- SELECT department, SUM(sales_amount)
- FROM employees
- JOIN sales ON employees.employee_id = sales.employee_id
- GROUP BY department;
- Ensure that the columns you join are indexed to optimize performance.
- Be mindful of the number of rows the JOIN returns to avoid unnecessarily performing aggregates on large datasets.
- Aggregate Functions: Aggregate functions collapse the rows into a single result per group. For example, SUM() or AVG() will return a single value for each group when used with GROUP BY.
- Window Functions: Window functions perform calculations across rows, but unlike aggregate functions, they do not collapse rows. Instead, they return the result for each row while allowing access to the grouped data.
- SELECT department, AVG(salary)
- FROM employees
- GROUP BY department;
- SELECT employee_id, department, salary,
- AVG(salary) OVER (PARTITION BY department) AS avg_salary
- FROM employees;
- Aggregate Functions collapse results in a single output per group.
- Window Functions retain the original row count while aggregating a specified “window” of rows.
- Indexes: Create indexes on columns frequently used in GROUP BY, ORDER BY, or as conditions in the WHERE clause. This helps speed up data retrieval before aggregation.
- Avoid Complex Subqueries: Complex subqueries can significantly slow down aggregate queries. When possible, try to use joins or temporary tables.
- Reduce the Dataset: Apply filters early in the query using the WHERE clause to reduce the number of rows processed before aggregation.
- Use Efficient Data Types: Ensure that the columns used for aggregation are of the appropriate data type, as this can affect the performance of aggregate operations.
- Use Partitioning: For large datasets, partition your data and perform aggregations on smaller, more manageable chunks.
- SELECT COUNT(salary) FROM employees;
- SELECT AVG(salary) FROM employees;
- SELECT SUM(COALESCE(salary, 0)) FROM employees;
- Sales Analysis: Summing up sales amounts, calculating average sales, and counting the number of transactions or customers over specific periods.
- Employee Salary Data: Calculating the average salary, the highest and lowest salaries, and the total salary expense within departments.
- Inventory Management: Summing up inventory counts, determining the maximum or minimum stock levels, and calculating the average sale per item.
- Financial Reports: Generating financial reports such as total revenue, expenses, profit margins, and average monthly earnings.
- Use Proper Indexing: Ensure relevant columns (especially those used in GROUP BY, JOIN, or WHERE clauses) are indexed.
- Avoid Aggregation on Large Datasets: Filter data as much as possible before performing aggregation, especially when dealing with large datasets.
Ready to Earn Your Data Science Certificate? View The Data Science Course Offered By ACTE Right Now!
HAVING Clause vs WHERE Clause
Both HAVING and WHERE filter results serve different purposes when used with aggregate functions.
WHERE Clause: This clause filters rows before aggregation. It works on individual records and filters rows before grouping or aggregating them.
This filters employees with a salary greater than 50,000 before performing the aggregation.
HAVING Clause: Filters results after aggregation. It is used to filter groups after the aggregate functions have been applied.
After grouping and calculating the average, this filters departments whose average salary exceeds 60,000.
Key Differences
Nested Aggregate Functions
A nested aggregate function is an aggregate function used within another aggregate function. This can be useful when you need to perform multiple levels of aggregation in a single query.
Example:
This query first calculates the average salary for each department and then finds the maximum average salary across all departments.
Example with Subqueries:
This query compares each employee’s salary to the overall average salary (calculated using a subquery) and only includes those with salaries above the overall average.
Interested in Pursuing Data Science Master’s Program? Enroll For Data Science Master Course Today!
Using Aggregate Functions with Joins
You can combine aggregate functions with JOIN operations to calculate aggregates across related tables. This is useful when you need to compute summaries based on data from various sources.
Example:
This query joins the employee table with the sales table and calculates the department sales amount.
Key Points:
Window Functions vs Aggregate Functions
Both window functions and aggregate functions perform calculations across rows, but they differ in how they handle results:
Example of Aggregate Function:
This returns one row per department with the average salary.
Example of Window Function:
This returns each row from the employee’s table along with the average salary for each department, but the rows are not collapsed.
Key Difference:
Preparing for a Data Science Job Interview? Check Out Our Blog on Data Science Interview Questions & Answer
Performance Optimization for Aggregate Queries
Aggregate queries can sometimes be slow, especially when working with large datasets, which is a common challenge highlighted in Data science Training. Here are a few tips for optimizing aggregate queries:
Handling NULL Values in Aggregates
In SQL, NULL values are treated differently by aggregate functions. Here are some important considerations:
COUNT(): The COUNT() function counts only non-NULL values. It does not count rows with NULL values in the specified column.
This counts only the rows where salary is not NULL.
SUM(), AVG(), MIN(), and MAX(): These functions ignore NULL values. For example, SUM() will only sum non-NULL values, and AVG() will calculate the average excluding NULL values.
This will calculate the average salary, excluding NULL values in the salary column.
Handling NULLs Explicitly: You can use COALESCE() or IFNULL() (depending on the database) to replace NULL values with a default value in aggregate functions.
This ensures that NULL values are treated as 0 when calculating the sum.
Real-World Applications of Aggregate Functions
Best Practices for SQL Aggregation
- Use Aliases for Readability: Always use aliases (e.g., AS average_salary) to make aggregate functions easier to understand.
- Test Queries: Always test aggregate queries on small datasets first to ensure correctness before scaling to larger datasets.
By following these best practices, you can effectively use aggregate functions to extract meaningful insights from your data while maintaining query performance and avoiding common pitfalls.
Data Science Sample Resumes! Download & Edit, Get Noticed by Top Employers! DownloadConclusion
Aggregate functions are fundamental tools in SQL that empower you to perform powerful data summarizations, generate insights, and drive informed decision-making. From calculating totals and averages to identifying trends and anomalies, these functions are critical in a wide range of applications—from business reporting to system monitoring. By mastering concepts such as GROUP BY, HAVING, joins, and nested queries through Data Science Training, and by understanding how aggregate functions differ from window functions, you can write more efficient and meaningful queries. Furthermore, optimizing performance, handling NULL values properly, and following best practices ensures that your queries remain accurate and scalable, even on large datasets. Ultimately, a solid grasp of aggregate functions enhances your ability to transform raw data into actionable intelligence—an essential skill for analysts, developers, and data engineers alike.
Example:
This returns the total sales amount from the orders table.
Example:
This returns the average age of employees in the employee’s table.