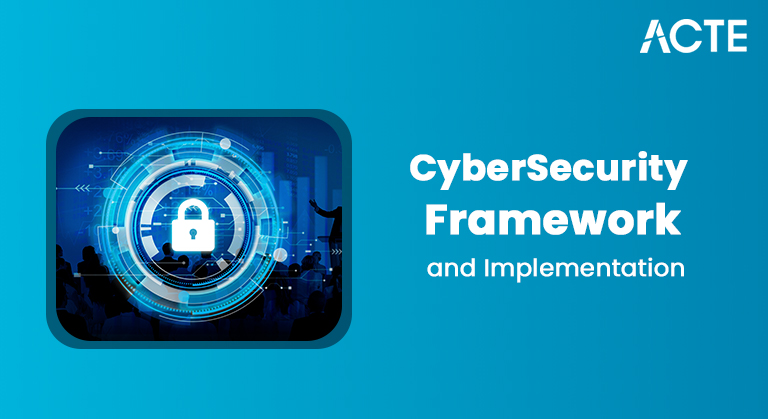
- Introduction to Map Function in Python
- How the Map Function Works
- Using Map with Built-in Functions and Lambda Functions
- Performance Comparison: Map vs List Comprehension
- Chaining Map with Other Functions
- Best Practices for Using Map Function
- Error Handling with Map
- Use Cases of Map in Data Science and Web Development
- Conclusion
Introduction to Map Function in Python
The map() function in Python is a powerful and flexible built-in feature that allows developers to apply a specified function to each item in an iterable, such as a list, tuple, or set. Instead of using traditional loops, which can often be verbose and repetitive, map() enables a cleaner and more concise way to perform operations on sequences of data. This makes it especially useful when the same transformation or calculation needs to be applied to multiple elements. What sets map() apart is that it returns a map object, which is essentially an iterator. This means it doesn’t compute all the results at once, but instead generates each value on demand. This approach, known as lazy evaluation, is highly efficient—something often emphasized in a Cloud Computing course particularly when dealing with large data sets, because it minimizes memory usage and allows for smoother data processing. Another advantage of map() is its ability to work seamlessly with both built-in and custom functions. You can pass in a named function, a lambda expression, or any callable object, making it highly versatile in various coding scenarios. In addition, map() can accept multiple iterables at once, applying the function to the corresponding elements from each iterable in parallel, which can be very convenient for operations involving multiple data sources. Because of its readability, efficiency, and the functional programming approach it encourages, map() is widely used in real-world Python applications. From transforming strings and performing mathematical operations to handling complex data processing tasks in areas like data science, automation, and web development, map() provides a streamlined way to manipulate and transform data with minimal code. In essence, the map() function is more than just a shortcut for loops—it’s a tool that promotes clearer, more Pythonic code and enhances the overall performance and maintainability of programs.
Excited to Obtaining Your Cloud Computing Certificate? View The Cloud Computing Course Offered By ACTE Right Now!
How the Map Function Works
The map() function takes two arguments: Functions and Iterable(s).
- Function: The function you want to apply to each element in the iterable. This can be a built-in function, a lambda function, or any custom function.
- Iterable(s): The iterable (or multiple tables) on which the function is applied. Typically, you pass in a list or a tuple, but other tables, like sets or dictionaries, can also be used.
- numbers = [1, 2, 3, 4, 5]
- result = map(lambda x: x * 2, numbers) # Apply lambda function that multiplies each number by 2
- print(list(result)) # Convert map object to list
- [2, 4, 6, 8, 10]
- Functionality: More functional programming style.
- Performance: Since map() returns an iterator, it is often more memory-efficient, especially for large datasets.
- Readability: Can be less readable, especially with complex functions.
- Functionality: A more Pythonic way of processing data.
- Performance: It generally performs well for smaller datasets but can consume more memory than a map() since it directly creates a list.
- Readability: Career in Cloud Computing are often easier to understand and follow, especially when the information is presented in a clear and straightforward manner.
- # Using map
- numbers = [1, 2, 3, 4, 5]
- result_map = list(map(lambda x: x * 2, numbers))
- # Using list comprehension
- result_list_comprehension = [x * 2 for x in numbers]
- print(result_map)
- print(result_list_comprehension)
- [2, 4, 6, 8, 10]
- [2, 4, 6, 8, 10]
- numbers = [1, 2, 3, 4, 5]
- result = map(lambda x: x * 2, map(lambda x: x + 1, numbers)) # First add 1, then multiply by 2
- print(list(result))
- [4, 6, 8, 10, 12]
- Keep Functions Simple: If the function passed to map() is too complex, it may decrease readability. Consider using list comprehensions or breaking the logic into smaller steps for more complex transformations.
- Use Map with Built-in Functions: Use built-in functions with map() for cleaner and more efficient code.
- Avoid Side Effects: Ensure that the function you use with map() does not have side effects. Functions with side effects can lead to unintended behavior.
- Use Lambda Functions Wisely: Python Lambda Function are concise, but overuse can lead to less readable code. If the logic is too complex, consider using regular functions.
- Handle Edge Cases: Always account for empty iterables or potential edge cases to avoid errors during transformation.
- Data Cleaning and Preprocessing in Data Science In data science, map() is often used for data cleaning tasks, such as converting string representations of numbers into actual numeric values. It can also handle other transformations like removing unwanted characters, normalizing data, or applying mathematical models to large datasets. This makes it a useful tool when dealing with messy or unstructured data, allowing for efficient and readable transformations without explicitly writing loops.
- Normalization and Transformation map() can be used to normalize or scale data for machine learning models. For example, applying a mathematical function like min-max scaling or z-score normalization across a dataset can be easily achieved with map(), saving time and reducing the complexity of the code. It also allows for consistent transformations across large datasets with minimal memory consumption, which is crucial when working with big data.
- Processing User Input in Web Development In Web Developement languages, map() is frequently used to process user input. For instance, if a web application receives a list of user IDs or form inputs, map() can quickly apply necessary transformations such as sanitizing the data, formatting it, or converting it into a required format for further processing.
- Formatting Data for APIs When interacting with external APIs, data often needs to be transformed into a specific format. map() can be used to process and reformat data before sending it to or receiving it from an API. For example, converting a list of timestamps into readable date strings, or adjusting field names to match API specifications, is a task that can be done efficiently using map().
- Manipulating Data Structures (JSON, Lists of Records) Data structures like JSON or lists of records are common in both data science and web development. map() is ideal for iterating over these structures and performing operations on each element. For example, in web development, you might need to extract specific fields from a JSON object or update values in a list of dictionaries, and map() allows for these operations to be done in a clean and efficient manner.
- Enhancing Code Efficiency and Readability In both fields, the ability to handle large datasets or complex transformations concisely is highly valuable. By using map(), developers can avoid writing cumbersome loops, which not only makes the code more readable but also enhances its performance by leveraging Python’s iterator protocol, especially when dealing with memory-intensive tasks.
- Batch Processing and Parallel Data Handling map() is often used in batch processing tasks, where multiple operations need to be applied to data in parallel. Whether processing logs, images, or large sets of numerical data, map() can apply the required transformations efficiently without loading everything into memory at once, making it highly effective for large-scale data processing.
Syntax:
map(function, iterable) The map() function returns a map object, an iterator that can be converted into a list, tuple, or other iterable types.
Examples:
Output
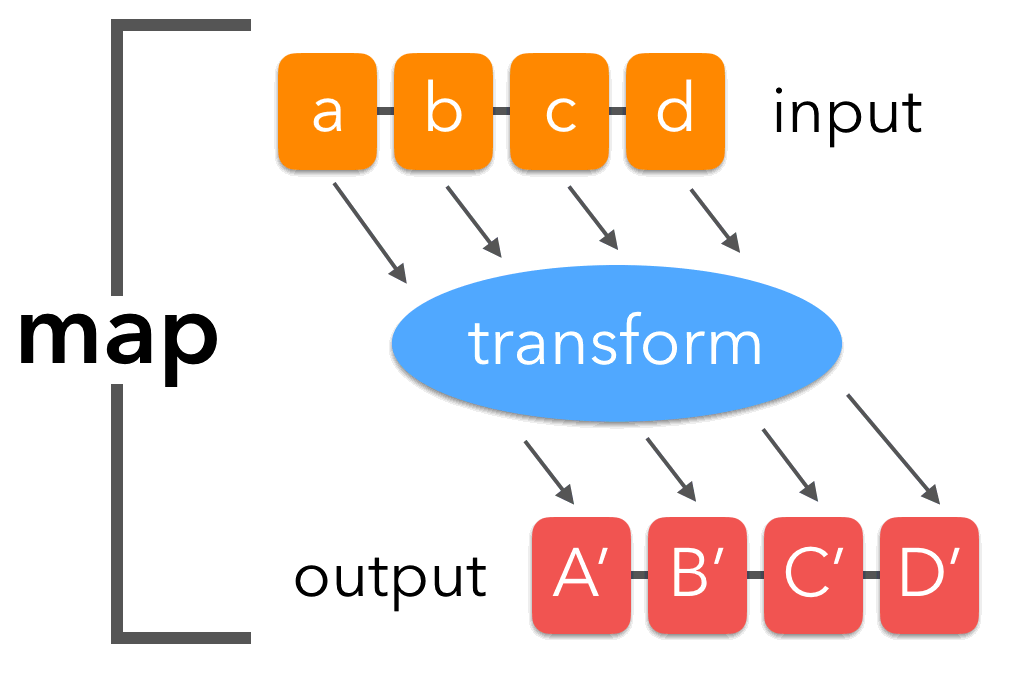
Using Map with Built-in Functions and Lambda Functions
The map() function is commonly used in combination with Python’s built-in functions to apply those functions to each element in an iterable. This approach simplifies many tasks that would otherwise require explicit loops. For example, when you need to convert a list of integers into strings, you can use the built-in str() function with map(). In this case, each number in the list is individually passed to the str() function, and the result is a new iterable where every element is a string. This method is efficient, concise, and maintains the readability of the code, making it a practical choice for transforming data types or applying any standard function across a collection, especially in a Cloud Computing Course where scalability and clarity are essential. Lambda functions, also known as anonymous functions, are particularly useful when paired with map() for performing quick, one-line operations. These functions don’t require a formal definition using the def keyword and are ideal for situations where a simple transformation is needed. When used with map(), a lambda function can be applied to each item in an iterable to perform element-wise modifications. For instance, if you want to square each number in a list, a lambda function that takes a single argument and returns its square can be passed directly to map(). This results in a streamlined and readable expression, making the transformation clear and efficient without cluttering the code with additional function definitions.
Performance Comparison: Map vs List Comprehension
Both map() and list comprehension are commonly used to transform data in Python. While both approaches serve the same purpose, they differ in syntax and performance characteristics.
Map:
List Comprehension:
Example of Performance Comparison:
Output:
Both approaches produce the same result. The main difference lies in performance and memory usage. When working with larger datasets or tables that don’t need to be fully loaded into memory, map() may be a better choice. However, for smaller datasets and readability, list comprehension is often preferred.
Ready to Earn Your Cloud Computing Certificate? View The Cloud Computing Course Offered By ACTE Right Now!
Chaining Map with Other Functions
One of the powerful features of the map() function is its ability to be chained with other functions, including built-in functions, lambda functions, or even other map () calls. This allows you to apply multiple transformations to data in a single line of code.
Example:
Output
In this example, the first map() adds 1 to each element, and the second map() multiplies each result by 2. The result is a sequence of numbers with both transformations applied.
Interested in Pursuing Cloud Computing Master’s Program? Enroll For Cloud Computing Master Course Today!
Best Practices for Using Map Function
By following these best practices, the map() function can significantly enhance the readability and performance of your Python code.
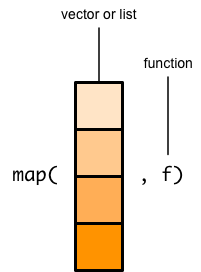
Error Handling with Map
Error handling is a critical aspect of working with the map() function. Since the function passed to map() is applied to each element in the iterable, it is essential to ensure that the function can handle various types of data and edge cases. This includes checking for incompatible data types that might not be suitable for the operation at hand. For example, if a function expects a numeric value but encounters a string or a None type, it could raise an exception and potentially halt the program. To avoid this, it’s advisable to implement input validation before applying the function, ensuring that each element in the iterable meets the required criteria. You could also incorporate exception handling (such as try-except blocks) within the function itself to catch and manage errors gracefully. This approach allows the program to continue processing other elements even if one element causes an error, providing a more robust and user-friendly experience. Additionally, it’s important to consider how the map() function behaves with empty iterables. Since map() returns an iterator, an empty iterable will simply result in an empty map object, and no elements will be processed—this is particularly relevant in event-driven environments like AWS Lambda, where input data may occasionally be absent or filtered out. While this may not cause an error, it could lead to unexpected results if not accounted for. For instance, if your program depends on processing a non-empty iterable, you may want to check the iterable’s length or ensure that it contains data before passing it to map(). This will help prevent unnecessary computations or issues when dealing with empty or invalid input data. In summary, handling errors in the context of the map() function requires anticipating potential problems such as type mismatches, empty iterables, and other edge cases. By using proper validation, exception handling, and thoughtful checks, you can prevent your program from crashing and ensure that the map() function runs smoothly across a wide range of scenarios.
Use Cases of Map in Data Science and Web Development
The map() function plays a crucial role in data science and web development due to its efficiency and versatility in handling large datasets and performing transformations in a concise manner. Below are several key use cases in these fields:
Preparing for Your Cloud Computing Interview? Check Out Our Blog on Cloud Computing Interview Questions & Answer
Conclusion
The map() function is a foundational feature in Python that empowers developers to process and transform data with efficiency, clarity, and minimal code. Its functional programming approach allows for elegant solutions to otherwise repetitive tasks, making it an invaluable tool when working with iterables like lists, tuples, or sets. By enabling element-wise transformations using built-in functions, custom functions, or lambda expressions, map() enhances both the performance and readability of code. Throughout various domains—whether in data science for data preprocessing and normalization, in web development for formatting and handling user inputs, or as part of a Cloud Computing course for managing distributed data workflows map() provides a scalable and memory-efficient method to manage complex data operations. When combined thoughtfully with other Python functionalities and guided by best practices, it can simplify logic, reduce errors, and promote clean, maintainable code. In summary, mastering the use of map() not only enriches your Python programming toolkit but also opens doors to writing more Pythonic, robust, and high-performing applications across a wide range of use cases.