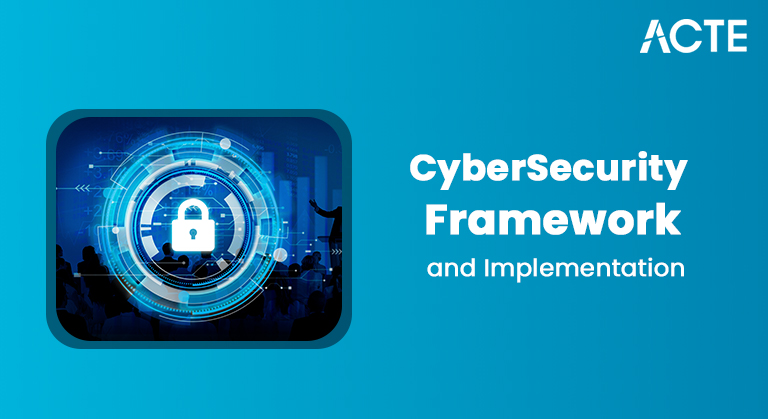
- Overview of AWS SDK
- Supported Languages and Installation
- Authentication and Authorization in AWS SDK
- Working with AWS Services (S3, EC2, DynamoDB)
- Error Handling and Logging
- Performance Optimization Strategies
- AWS SDK vs AWS CLI: When to Use What
- Real-World Applications and Use Cases
- Conclusion
Overview of AWS SDK
The AWS Software Development Kit (SDK) is a collection of libraries, tools, and documentation that allows developers to build applications that interact with Amazon Web Services (AWS). The SDK provides a simplified and more efficient way to access AWS services programmatically by abstracting complex API calls and offering high-level interfaces for multiple programming languages. The Amazon Web Service Training supports various AWS services, including computing, storage, databases, machine learning, and more. It helps developers integrate their applications with AWS services like Amazon S3, EC2, DynamoDB, Lambda, SNS, SQS, and others. Using the SDK, developers can automate infrastructure, manage resources, handle scalability, and enable features like encryption, authentication, and access control.
The AWS SDK makes it easier for developers to work with AWS by offering:
- High-level API libraries in several programming languages.
- Utility functions for automatic retries, error handling, and credentials management.
- Support for multiple platforms, including mobile devices, serverless applications, and web apps.
Supported Languages and Installation
AWS SDKs are available in a variety of programming languages, ensuring developers can integrate AWS services into applications regardless of their preferred development environment. Below are the key languages supported by AWS SDKs:
JavaScript (Node.js and Browser)
- Installation: You can install the AWS SDK for JavaScript via npm (Node Package Manager). Run the following command in your terminal:
- npm install aws-sdk
For browser-based applications, the AWS SDK is available as a JavaScript library.
Python (Boto3)
- Installation: The AWS SDK for Python is called Boto3. It can be installed using pip (Python package manager):
- pip install boto3
Java
- Installation: The AWS SDK for Java can be integrated into a project via Maven or Gradle. To add it via Maven, include the following dependency in your pom.xml:
com.amazonaws aws-java-sdk 1.11.0
C# (.NET)
- Installation: The AWS SDK for .NET can be installed via the NuGet package manager:
- Install-Package AWSSDK.Core
Ruby
- Installation: The Ruby SDK can be installed via RubyGems:
- Gem install aws-sdk
Go
- Installation: The Go SDK can be installed using the Go module system:
- go get github.com/aws/aws-sdk-go
PHP
- Installation: The AWS SDK for PHP can be installed via Composer:
- composer require aws/aws-sdk-php
Android (Java)
- Installation: Android developers can use the AWS SDK for Android by adding it as a dependency in their build.gradle file:
- implementation ‘com.amazonaws:aws-android-sdk-core:2.16.0’
iOS (Swift/Objective-C)
- Installation: iOS developers can integrate the AWS SDK for iOS via CocoaPods:
- pod ‘AWSCore’
Each SDK typically has its installation guides and documentation, ensuring developers can quickly get started on their preferred platform.
Advance your AWS career by joining this AWS Online Course now.
Authentication and Authorization in AWS SDK
Authentication and authorization are critical aspects of working with AWS SDKs. They ensure that only authorized users and services can access resources on AWS. Authentication verifies the identity of users or services, while authorization determines the level of access they have to AWS resources. AWS provides various methods for authentication, including IAM roles, access keys, and temporary credentials through services like AWS STS. Properly configuring authentication and authorization policies ensures security and compliance within the AWS environment.
Authentication
AWS uses Access Keys for authentication. These consist of:
- Access Key ID
- Secret Access Key
These keys are used to sign API requests made by the SDK. For added security, AWS also provides temporary credentials via the AWS Security Token Service (STS).There are several ways to authenticate using the AWS SDK, For local development, you can set the AWS_ACCESS_KEY_ID and AWS_SECRET_ACCESS_KEY environment variables, The AWS CLI and SDKs can use the ~/.aws/credentials file, which stores multiple profiles of access keysYou can assign IAM roles to EC2 instances, Lambda functions, or ECS containers to authenticate automatically without embedding keys in the application. Additionally, IAM roles for EC2 instances can be assigned permissions to interact with other AWS services securely, eliminating the need for static credentials. For enhanced security in production environments, AWS recommends using AWS Snowmobile Exabyte Scale Data Migration and Access Management (IAM) roles and policies to manage access dynamically. Furthermore, AWS Secrets Manager can securely store and manage API keys, credentials, and other sensitive information, ensuring they are not hard-coded into applications.
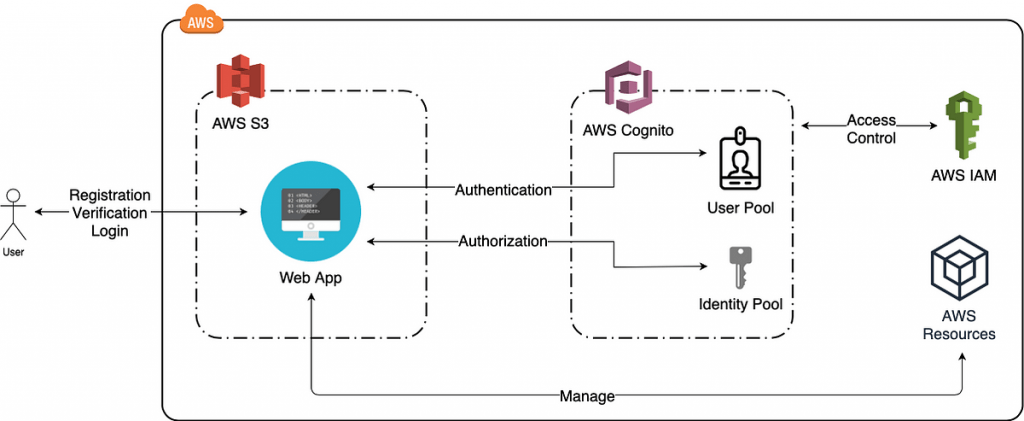
Authorization
Authorization in AWS is managed through AWS Identity and Access Management (IAM). IAM enables you to define, Who can access AWS resources? Define what actions users and services can perform on specific resources. Assign permissions for services like EC2 or Lambda to access resources on behalf of the user. You can create and manage individual IAM users and organize them into groups for easier permission management. IAM allows you to define roles with specific permissions, which can be assumed by users, services, or applications to perform actions on AWS resources. Policies are JSON documents that define permissions and can be attached to users, groups, or roles to control access to AWS services and resources. AWS provides temporary security credentials through AWS STS (Security Token Service) for limited access to resources, enhancing security for short-term tasks or external applications. MFA adds an extra layer of security by requiring users to provide two forms of authentication, such as a password and a code from an MFA device, before accessing AWS resources. AWS Well Architected Framework for Success tool helps identify unintended access to resources by analyzing resource policies and generating findings that guide users in adjusting permissions to ensure security. IAM encourages the use of the least privilege principle, ensuring that users and services only have the minimum permissions necessary to perform their tasks, reducing the risk of unauthorized access or accidental damage. In the AWS SDK, you can specify which IAM role or user should be assumed when interacting with resources. The SDK will then use the appropriate permissions attached to that IAM identity to authorize access.
Working with AWS Services (S3, EC2, DynamoDB)
The AWS SDK provides powerful methods to interact with AWS Training like Amazon S3, EC2, and DynamoDB.
Amazon S3 (Simple Storage Service)
Amazon S3 is an object storage service for storing and retrieving any amount of data. The AWS SDK makes it simple to manage buckets and objects.
Basic Operations:
- Create a Bucket: Create an S3 bucket to store data.
- Upload and Download Files: Upload files to S3 and download files from S3 using the SDK.
- Delete Files: You can delete objects from your S3 bucket programmatically.
Example in Python (Boto3):
- import boto3
- s3 = boto3.client(‘s3’)
- s3.upload_file(‘local_file.txt’, ‘my-bucket’, ‘remote_file.txt’)
Amazon EC2 (Elastic Compute Cloud)
Start your journey in AWS by enrolling in this AWS Online Course .
Amazon EC2 provides scalable computing capacity in the cloud. With the AWS SDK, you can manage EC2 instances, such as starting, stopping, and terminating instances.
Basic Operations:
- Launch an Instance: SDK is used to launch EC2 instances with specific configurations.
- Monitor EC2 Instances: Retrieve instance states and monitor resource usage.
- Terminate Instances: Programmatically stop or terminate EC2 instances.
Example in Python (Boto3):
- import boto3
- ec2 = boto3.client(‘ec2’)
- response = ec2.run_instances(
- ImageId=’ami-0abcdef1234567890′,
- InstanceType=’t2.micro’,
- MinCount=1,
- MaxCount=1)
Amazon DynamoDB
DynamoDB is a NoSQL database service provided by AWS. The SDK allows you to interact with DynamoDB tables for data storage and retrieval.
Basic Operations:
- Create a Table: Define the schema and provision capacity for DynamoDB tables.
- Insert and Query Data: Add, retrieve, and update items in the database.
- Delete Items: Programmatically delete items from DynamoDB.
Example in Python (Boto3):
- import boto3
- dynamodb = boto3.resource(‘dynamodb’)
- table = dynamodb.Table(‘MyTable’)
- response = table.put_item(
- Item={
- ‘ID’: ‘123’,
- ‘Name’: ‘John Doe’
- }
- )
Error Handling and Logging
AWS SDKs include robust error handling and logging mechanisms to make it easier for developers to diagnose issues.
Take charge of your AWS career by enrolling in ACTE’s AWS Master Program Training Course today!
Error Handling
The SDK provides exceptions that capture errors from API calls, which can be caught using try-catch blocks in most programming languages. Common errors include throttling, which occurs when API rate limits are exceeded, and authentication errors, which arise from invalid credentials or lack of permissions. Another frequent error is ResourceNotFoundException, raised when attempting to access a resource that doesn’t exist. AccessDeniedException happens when the Seamless Cloud Migration Guide or service lacks sufficient permissions to perform the requested action. ThrottlingException occurs when the rate of requests exceeds the allowed limit for an AWS service, resulting in temporary throttling. ValidationException is triggered when input parameters for an API call are invalid or fail to meet required constraints. Finally, InternalServerError is generated when there is an unexpected issue on the AWS service’s side, preventing the request from being processed successfully.
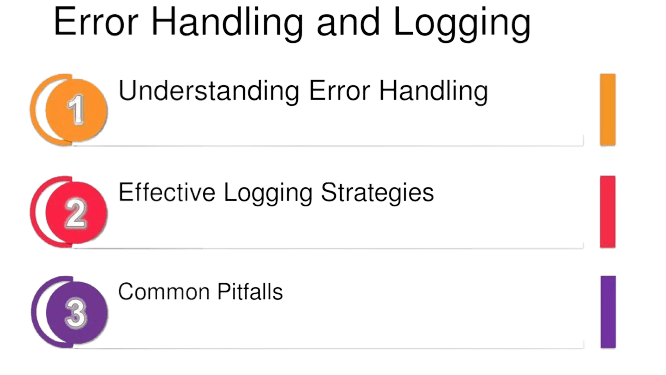
Logging
The AWS SDK integrates with AWS CloudWatch Logs and can also output logs locally for debugging. Logs can capture SDK interactions, request-response cycles, and error details. These logs provide valuable insights into the API calls made by your application, including the request parameters, responses, and any errors encountered. You can configure the SDK to log at different levels, such as INFO, DEBUG, or ERROR, to control the amount of detail captured. By integrating with AWS CloudWatch Logs, you can centralize and monitor logs across multiple AWS resources and AWS Journey 10 Beginner Friendly Projects. This makes it easier to track application performance, troubleshoot issues, and ensure smooth operation of your AWS-based applications. Additionally, local logging can be enabled to assist during development, providing immediate feedback without relying on cloud resources.
Performance Optimization Strategies
When interacting with AWS services via the SDK, performance can sometimes be a significant concern when scaling applications. Here are some strategies for optimization:
- Use Pagination: For services like S3 and DynamoDB that return large datasets, pagination retrieves data in chunks.
- Efficient Use of Batching: For operations like inserting multiple items into DynamoDB, use batch operations to reduce the number of API calls.
- Retries and Backoff: To handle throttling and transient errors, use the SDK’s built-in retry mechanisms with exponential backoff.
- Parallel Processing: Run multiple asynchronous operations in parallel to speed up the processing of large data volumes.
Preparing for AWS interviews? Visit our blog for the best AWS Interview Questions and Answers!
AWS SDK vs AWS CLI: When to Use What
The AWS SDK and the AWS CLI (Command Line Interface) are tools for interacting with AWS services but serve different purposes.
Feature | AWS SDK | AWS CLI |
---|---|---|
Ideal Use | Embedding AWS interactions directly into application code | Best for one-off tasks, scripts, and automation in shell environments |
Use Case | Programmatically accessing AWS services, managing resources dynamically, integrating with other software | Administrative tasks, resource management, quick operations without writing a complete application |
Environment | Used in applications across various languages (Python, Java, etc. | Primarily used in command-line interfaces or shell scripts |
Customization | Offers fine-grained control and customization for application-level logic | Quick, straightforward commands for resource management and automation |
Flexibility | Highly flexible, allowing integration with complex systems and workflows | Simplified and focused on command execution and scripting tasks |
Real-World Applications and Use Cases
- Web Application Hosting: EC2 instances are used for computing, S3 for file storage, and RDS for databases.
- Mobile App Backend: Using Lambda for serverless computation, Understanding the AWS Stat Machine for data storage, and SNS for push notifications.
- Data Pipelines: Automate data ingestion with S3, transform it with Lambda, and store it in Redshift for analysis.
- Real-time Analytics: Use Kinesis for real-time data streaming, Lambda for processing, and Elasticsearch for fast data search and analysis.
- Machine Learning: Leverage SageMaker for model training, S3 for data storage, and API Gateway to serve predictions in real-time.
- Content Delivery: Use CloudFront for global content distribution, S3 for static file storage, and Route 53 for DNS management and routing.
Conclusion
In conclusion, the AWS SDK is a vital tool for developers looking to leverage AWS services in their applications. Understanding its capabilities and how to use AWS Training effectively is key to building robust, scalable, and optimized applications. With its support for various programming languages and services, the SDK simplifies the process of integrating AWS resources into your code. By utilizing best practices for authentication, error handling, and performance optimization, developers can ensure their applications run smoothly and efficiently. Whether you’re building a web application, mobile app, or data pipeline, the AWS SDK provides the necessary tools to interact with AWS services seamlessly. Embracing the AWS SDK will empower developers to take full advantage of the cloud’s potential and build innovative solutions with ease.